Building a Chatbot using Flask and Microsoft Dialogpt
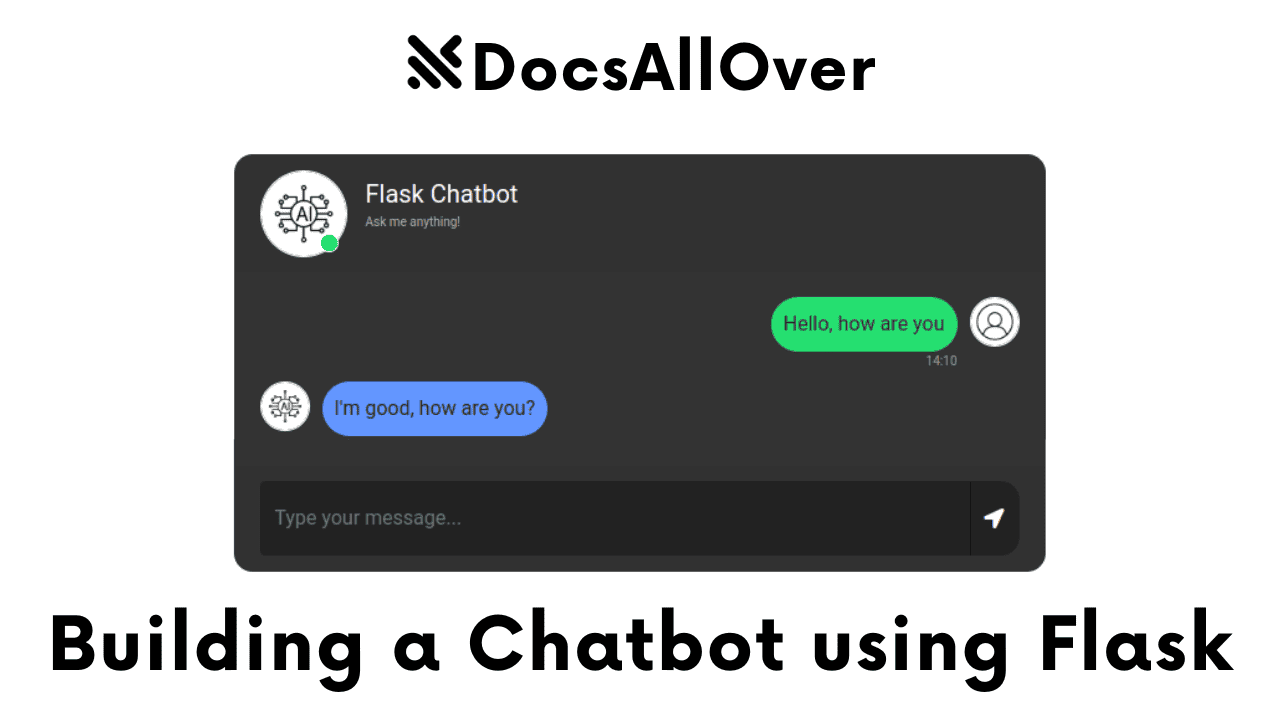
What is a Chatbot?
A chatbot is a computer program designed to simulate a conversation with a human user, typically through text or text-to-speech. Chatbots can be used for various purposes, such as customer service, providing information, and even entertainment.
Importance of Chatbots in Today's World
Chatbots have become increasingly important in today's digital world due to several factors:
- 24/7 Availability: Chatbots can provide 24/7 customer support and assistance, regardless of time zones or holidays.
- Increased Efficiency: They can automate repetitive tasks, freeing up human agents to focus on more complex issues.
- Improved Customer Experience: Chatbots can provide quick and personalized responses to customer inquiries, improving overall customer satisfaction.
- Cost-Effectiveness: Implementing chatbots can reduce operational costs associated with customer support.
Overview of Flask and its Role in Web Development
Flask is a lightweight and flexible Python web framework. It provides the necessary tools and libraries to build web applications, including routing, request handling, and template rendering. Flask's simplicity and ease of use make it an excellent choice for building web applications, including chatbots.
Introducing Microsoft DialoGPT: A Pre-trained Conversational AI Model
Microsoft DialoGPT is a powerful pre-trained transformer-based model specifically designed for conversational AI. It has been trained on a massive dataset of human conversations and can generate human-like text responses to a wide range of prompts. Using DialoGPT significantly reduces the time and effort required to build a high-quality chatbot.
Setting Up the Development Environment
Before we start building our chatbot, we need to set up the necessary development environment.
- Installing Python and Necessary Libraries
- Install Python: If you don't have Python installed, download and install the latest version from the official Python website (python.org).
- Install required libraries: Use
pip
, the Python package installer, to install the necessary libraries:pip install Flask transformers torch
Flask
: The web framework for building the chatbot application.transformers
: A library for working with state-of-the-art natural language processing models, including DialoGPT.torch
: The PyTorch deep learning library, required for running the DialoGPT model.
- Alternate method: Use pip, the Python package installer, to install the necessary libraries in
requirements.txt
file:pip install -r requirements
- Creating a Flask Project Structure
Create a new directory for your project (e.g.,
chatbot_project
). Inside the directory, create the following files and folders:app.py
: The main application file.templates/
: A directory to store HTML templates, such aschat.html
.requirements.txt
: A file to list the project dependencies.
- Setting Up a Virtual Environment (Recommended)
- Create a virtual environment to isolate project dependencies and avoid conflicts with other projects:
python -m venv venv
- Activate the virtual environment:
- On Linux/macOS:
source venv/bin/activate
- On Windows:
venv\Scripts\activate
- On Linux/macOS:
- Now, install the required libraries within the activated virtual environment:
pip install Flask transformers torch
- Create a virtual environment to isolate project dependencies and avoid conflicts with other projects:
With the development environment set up, we are ready to start building our chatbot application. In the next section, we will delve into the implementation details.
Project Implementation
Now, let's build our chatbot application step-by-step!
- Loading the Model:
This code imports the necessary libraries and loads the pre-trained DialoGPT model and tokenizer from the transformers library. The tokenizer helps convert text into numerical representations the model understands, and the model performs the text generation based on the input.
- Flask App Setup:
This code creates a Flask application instance (
app
) and defines two routes:/
: This route renders thechat.html
template, which will likely contain an input field for the user to type their message and a display area to show the conversation history./get
: This route handles both GET and POST requests for chat interaction. It extracts the user's message from the request form and calls theget_Chat_response
function to generate a response.
- Chat Response Generation (
get_Chat_response function
): - HTML Template (chat.html)
Output:
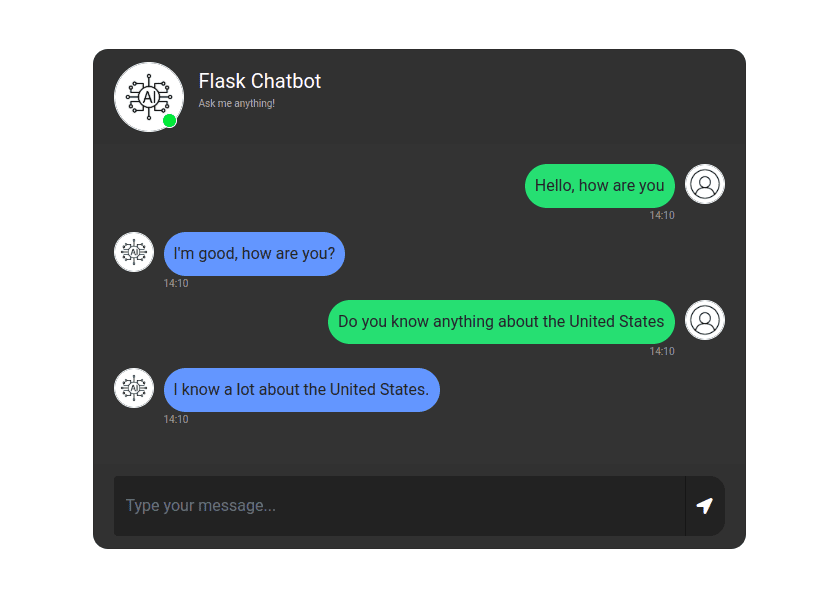
Download From Github
Download RepositoryNote:
This is a basic implementation, and you can enhance it further by:
- Implementing a more sophisticated chat history management mechanism.
- Adding features like sentiment analysis or personalization based on conversation context.
- Designing a more user-friendly chat interface using HTML, CSS, and JavaScript.
This implementation provides a starting point for building a chatbot using Flask and Microsoft DialoGPT. Remember to experiment and explore the capabilities of these libraries to create more advanced chatbots.