Scikit-learn: Machine Learning in Python
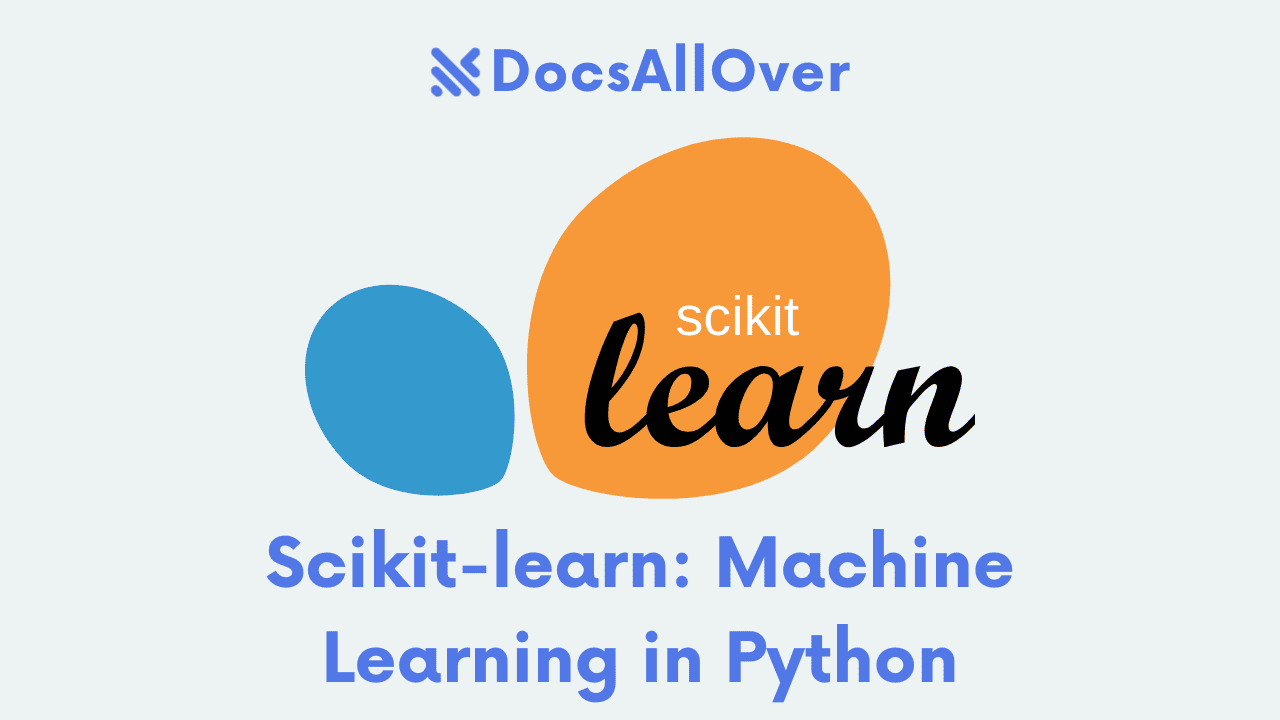
What is Scikit-learn?
Scikit-learn is a powerful open-source Python library for machine learning. It provides a simple and efficient interface for building and training various machine learning models. Scikit-learn is built on top of NumPy, SciPy, and Matplotlib, making it a versatile tool for data scientists and machine learning practitioners.
Key Features of Scikit-learn
- Wide range of algorithms: Scikit-learn includes a comprehensive collection of machine learning algorithms, covering supervised learning (classification and regression), unsupervised learning (clustering and dimensionality reduction), and ensemble methods.
- User-friendly API: The library has a consistent and intuitive API, making it easy to learn and use.
- Efficiency: Scikit-learn is optimized for performance and can handle large datasets efficiently.
- Integration with other libraries: It seamlessly integrates with other popular Python libraries like NumPy, Pandas, and Matplotlib.
- Community and support: Scikit-learn has a large and active community, providing extensive documentation, tutorials, and support.
Benefits of Using Scikit-learn for Machine Learning
- Efficiency: Scikit-learn is designed for efficient computation, making it suitable for large datasets.
- Ease of use: The library's user-friendly API and consistent interface make it easy to learn and use.
- Versatility: Scikit-learn offers a wide range of algorithms for various machine learning tasks.
- Integration with other tools: It seamlessly integrates with other popular Python libraries, making it a valuable tool for data scientists.
- Community and support: The large and active community provides extensive documentation, tutorials, and support.
Example:
In this example, we load the Iris dataset, split it into training and testing sets, create a decision tree classifier, train the model, make predictions, and evaluate the accuracy.
Core Concepts of Machine Learning
Supervised Learning
In supervised learning, the algorithm is trained on a dataset with labeled examples. The goal is to learn a mapping function that can predict the correct output for new, unseen data.
- Classification: Predicting categorical outcomes (e.g., spam or not spam, customer churn or not churn).
- Regression: Predicting numerical values (e.g., house prices, sales revenue).
Unsupervised Learning
In unsupervised learning, the algorithm is trained on a dataset without labels. The goal is to find patterns, structures, or relationships within the data.
- Clustering: Grouping similar data points together.
- Dimensionality reduction: Reducing the number of features in a dataset while preserving important information.
Classification
Classification algorithms predict categorical outcomes. Examples include:
- Decision Trees: Create tree-like models to make decisions based on attributes.
- Support Vector Machines (SVMs): Find a hyperplane to separate data points into different classes.
- Naive Bayes: Based on Bayes' theorem, assuming independence between features.
- K-Nearest Neighbors (KNN): Classifies data points based on their similarity to nearby neighbors.
Regression
Regression algorithms predict numerical values. Examples include:
- Linear Regression: Fits a linear relationship between the features and the target variable.
- Ridge Regression: A regularization technique that adds a penalty term to the loss function to prevent overfitting.
- Lasso Regression: Another regularization technique that can be used for feature selection.
Clustering
Clustering algorithms group similar data points together. Examples include:
- K-means clustering: Partitions data into K clusters based on the distance between data points.
- Hierarchical clustering: Creates a hierarchy of clusters, starting with individual data points and merging them into larger clusters.
Dimensionality Reduction
Dimensionality reduction techniques reduce the number of features in a dataset while preserving important information. Examples include:
- Principal Component Analysis (PCA): Finds the principal components of the data and projects the data onto these components.
- t-SNE (t-Distributed Stochastic Neighbor Embedding): Preserves local structure in the data while mapping it to a lower-dimensional space.
By understanding these core concepts, you can effectively apply machine learning techniques to solve various problems.
Common Algorithms in Scikit-learn
Linear Regression
- Used for: Regression tasks where the relationship between the features and the target variable is linear.
- Equation:
y = w0 + w1*x1 + w2*x2 + ... + wn*xn
- Example: Predicting house prices based on features like size, location, and number of bedrooms.
Logistic Regression
- Used for: Classification tasks where the target variable is binary (e.g., spam or not spam, customer churn or not churn).
- Equation:
p(y=1|x) = 1 / (1 + exp(-w0 - w1*x1 - w2*x2 - ... - wn*xn))
- Example: Predicting whether a customer will churn based on factors like account balance, transaction frequency, and customer service interactions.
Decision Trees
- Used for: Both classification and regression tasks.
- Algorithm: Creates a tree-like model where each node represents a decision and each branch represents a possible outcome.
- Example: Predicting whether a loan applicant will default based on factors like income, credit score, and debt-to-income ratio.
Random Forests
- Used for: Both classification and regression tasks.
- Algorithm: An ensemble method that creates multiple decision trees and combines their predictions.
- Example: Predicting customer churn by combining the predictions of multiple decision trees.
Support Vector Machines (SVMs)
- Used for: Classification and regression tasks.
- Algorithm: Finds a hyperplane that separates the data points into different classes.
- Example: Classifying images into different categories (e.g., cat, dog, car).
K-Nearest Neighbors (KNN)
- Used for: Classification and regression tasks.
- Algorithm: Classifies or predicts the value of a new data point based on the majority class or average value of its k nearest neighbors.
- Example: Predicting a user's movie preferences based on the preferences of similar users.
Naive Bayes
- Used for: Classification tasks.
- Algorithm: Assumes that the features are independent given the class label.
- Example: Classifying email as spam or not spam based on the frequency of certain words.
Clustering Algorithms
- K-means: Partitions data into K clusters based on the distance between data points.
- Hierarchical clustering: Creates a hierarchy of clusters, starting with individual data points and merging them into larger clusters.
Example:
These are just a few examples of the many algorithms available in Scikit-learn. The choice of algorithm depends on the specific problem you are trying to solve and the characteristics of your data.
Implementing Machine Learning Models with Scikit-learn
Data Preprocessing
Before training a machine learning model, it is essential to preprocess your data. This typically involves:
- Handling missing values: Imputing missing values or removing rows with missing values.
- Encoding categorical features: Converting categorical features into numerical representations (e.g., one-hot encoding).
- Feature scaling: Normalizing or standardizing numerical features to ensure they have a similar scale.
- Feature selection: Selecting the most relevant features to improve model performance and reduce overfitting.
Example:
Model Selection and Training
- Choose a suitable algorithm: Select an algorithm based on the nature of your problem (classification, regression, clustering, etc.) and the characteristics of your data.
- Create a model instance: Instantiate the chosen algorithm.
- Train the model: Fit the model to your training data using the
fit()
method.
Example:
Model Evaluation
Evaluate the performance of your model using appropriate metrics. Common metrics include:
- Accuracy: For classification problems.
- Precision, recall, F1-score: For classification problems.
- Mean squared error (MSE): For regression problems.
- R-squared: For regression problems.
Example:
Hyperparameter Tuning
Hyperparameters are parameters that are set before training the model. Tuning hyperparameters can help improve model performance.
Example:
By following these steps and experimenting with different algorithms and hyperparameters, you can effectively implement machine learning models using Scikit-learn.
Case Studies: Real-world Applications of Scikit-learn
Customer Churn Prediction
- Problem: A telecommunications company wants to identify customers who are likely to churn so they can take proactive steps to retain them.
- Solution: Use Scikit-learn to build a classification model that predicts customer churn based on factors like usage patterns, customer satisfaction, and contract length.
Fraud Detection
- Problem: A financial institution wants to detect fraudulent transactions before they occur.
- Solution: Use Scikit-learn to build a classification model that identifies fraudulent transactions based on patterns in transaction data.
Image Classification
- Problem: Classify images into different categories (e.g., cat, dog, car).
- Solution: Use Scikit-learn's image processing tools and classification algorithms to build an image classification model.
Medical Diagnosis
- Problem: Predict diseases based on patient symptoms and medical history.
- Solution: Use Scikit-learn to build a classification model that diagnoses diseases based on relevant features.
Customer Segmentation
- Problem: Group customers into different segments based on their characteristics and behaviors.
- Solution: Use clustering algorithms in Scikit-learn to identify distinct customer segments.
Recommendation Systems
- Problem: Recommend products or services to users based on their preferences and past behavior.
- Solution: Use collaborative filtering or content-based filtering techniques in Scikit-learn to build recommendation systems.
Natural Language Processing (NLP)
- Problem: Analyze and understand text data.
- Solution: Use Scikit-learn's NLP tools for tasks like sentiment analysis, text classification, and topic modeling.
These are just a few examples of how Scikit-learn can be applied to real-world problems. The versatility of Scikit-learn makes it a valuable tool for data scientists and machine learning practitioners across various industries.