Demystifying Inheritance and Polymorphism in Java
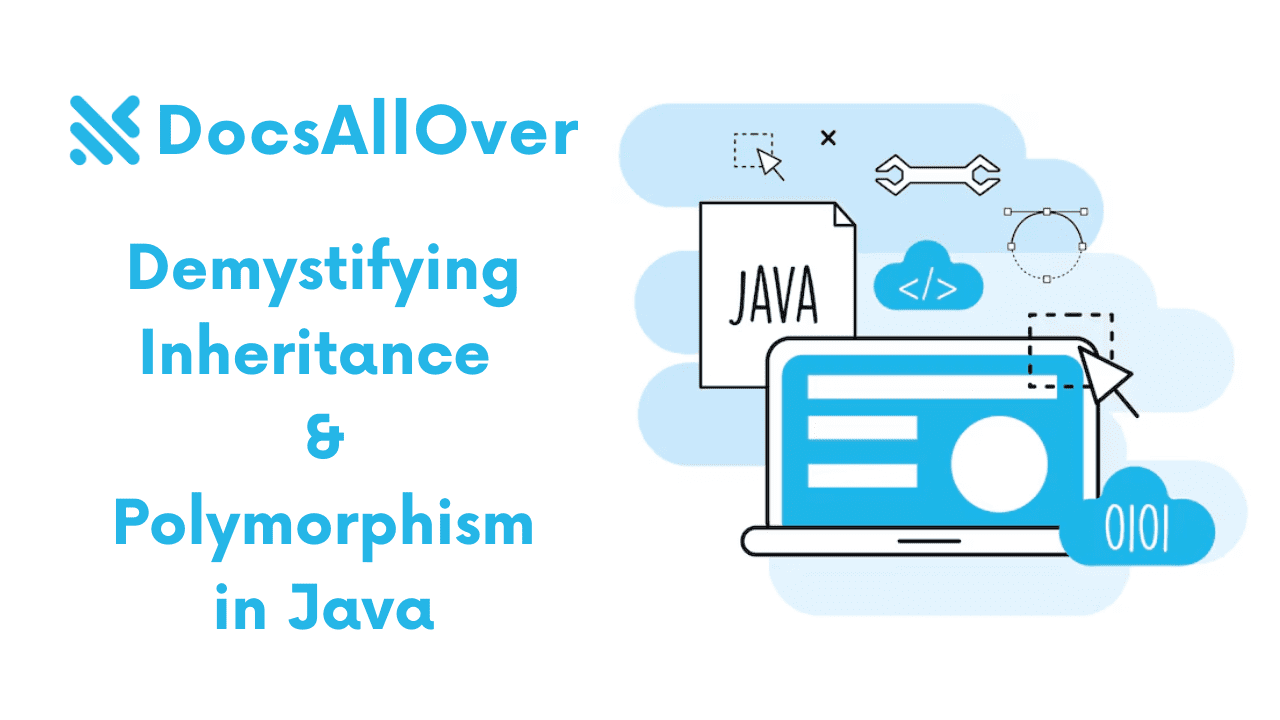
Object-Oriented Programming: Building Better Code with Reusability and Structure
Ever spent hours writing similar Java code, only to realize you're essentially copying and pasting yourself into a maze of redundancy? Or perhaps you've grappled with the challenge of managing intricate relationships between objects in your codebase, feeling like you're wrangling a tangled mess. If these scenarios sound familiar, fret no more, fellow Java developer, for Object-Oriented Programming (OOP) swoops in as your knight in shining armor!
OOP is a fundamental programming paradigm that prioritizes code reusability, maintainability, and modularity. Imagine constructing a magnificent building using pre-fabricated components that are designed to work together seamlessly, rather than having to meticulously craft every single brick from scratch. That's the magic of OOP! Within the realm of OOP, two cornerstone concepts, inheritance and polymorphism, empower you to architect flexible and reusable code structures.
Inheritance acts as a blueprint inheritance, allowing you to establish a hierarchy between classes. Just like a blueprint for a house serves as a foundation for building similar houses, inheritance enables you to create new classes (subclasses) that inherit properties and behaviors from existing classes (superclasses). This inheritance mechanism is a powerful tool that saves you time and effort, as you don't have to rewrite common functionalities from scratch. Imagine creating a base class for all Shape
objects in your program, and then deriving specific subclasses like Circle
, Square
, and Triangle
from this base class. The base class can define methods to calculate properties like area and perimeter, which can then be inherited and potentially overridden by the subclasses to provide more specific implementations. This inheritance hierarchy fosters code reusability and reduces redundancy in your Java applications.
Polymorphism, on the other hand, injects a layer of flexibility into your code by enabling objects of different classes to respond differently to the same method call. Imagine having a method called draw
that can be invoked on various shapes in your program. The specific implementation of the draw
method might differ based on the actual shape (circle, square, triangle). Polymorphism ensures that the appropriate draw
method is executed at runtime depending on the object's class. This allows you to write generic code that can operate on different types of objects without explicitly knowing their exact classes beforehand. By harnessing the power of inheritance and polymorphism, you'll be well on your way to crafting clean, efficient, and powerful Java applications that are a joy to maintain and scale.
Inheritance: Building Upon Existing Code
Now that we've explored the wonders of OOP and its emphasis on reusability, let's delve deeper into inheritance, a cornerstone concept that empowers you to leverage existing code to build new classes.
What is Inheritance?
Imagine you're a skilled architect. Inheritance allows you to create new building blueprints (subclasses) that inherit core elements like walls, foundations, and doors from a pre-existing blueprint (superclass) for a generic building type. In the world of OOP, inheritance works similarly. A subclass inherits properties (fields) and behaviors (methods) from a superclass, establishing an is-a
relationship between them. For instance, a class named Animal
could be a superclass, containing properties like name
and age
and methods like eat
and sleep
. A subclass named Dog
can then inherit these properties and methods from Animal
, while potentially adding its own specific properties like breed
and methods like bark
. This inheritance mechanism reduces redundancy and streamlines code development.
Benefits of Inheritance:
There are several advantages to incorporating inheritance into your Java code:
- Code Reusability: By inheriting properties and methods from a superclass, you avoid duplicating code, saving time and effort. Imagine writing an
Animal
class with amove
method. Subclasses likeDog
andBird
can inherit this method and potentially override it to provide specific movement behaviors (walking for dogs, flying for birds). - Reduced Redundancy: Inheritance eliminates the need to rewrite common functionalities in each class. The superclass acts as a central repository for shared code, promoting a cleaner and more maintainable codebase.
- Hierarchical Class Organization: Inheritance fosters a natural hierarchical structure for your classes, reflecting real-world relationships. Superclasses represent broader categories, while subclasses inherit and specialize those functionalities.
Types of Inheritance in Java:
Java supports various inheritance patterns, allowing you to model different class relationships:
- Single Inheritance: A subclass inherits from one direct superclass. This is the most common and straightforward type of inheritance.
- Multilevel Inheritance: A subclass inherits from a superclass that itself inherits from another superclass, forming a chain of inheritance.
- Hierarchical Inheritance: Multiple subclasses inherit from a single superclass, creating a branching hierarchy.
- Hybrid Inheritance (not recommended in practice): This is a theoretical concept where a subclass inherits from multiple superclasses directly. However, due to potential complexities, it's generally discouraged in Java programming.
Code Example: Let's See Inheritance in Action!
Here's a simple Java code example showcasing inheritance:
In this example, the Dog
class inherits properties (name
and age
) and a method (eat
) from the Animal
class. The Dog
class also has its own specific property (breed
) and method (bark
). This demonstrates how inheritance allows you to create new classes that leverage existing functionality while adding their own unique characteristics.
By understanding inheritance and its different forms, you can construct well-organized and reusable class hierarchies in your Java programs.
Polymorphism: Many Faces of a Method
What is Polymorphism?
Polymorphism, another cornerstone of OOP, introduces the concept of objects taking on multiple forms, allowing them to respond differently to the same method call. This dynamic behavior adds flexibility and adaptability to your code.
Method Overriding vs. Overloading
While both involve methods with the same name, they have distinct characteristics:
- Method Overriding: This occurs when a subclass provides a specific implementation for a method inherited from its superclass. The method signature (name and parameter list) remains the same, but the body of the method is different. This allows you to customize behavior based on the specific subclass.
- Method Overloading: This refers to having multiple methods with the same name in the same class, but with different parameter lists. The compiler determines which method to call based on the arguments passed at runtime. This enables you to create methods with the same name but different functionalities based on the input parameters.
Runtime Polymorphism (Dynamic Binding)
The magic of polymorphism truly shines in runtime polymorphism, also known as dynamic method dispatch. This occurs when a method call is resolved at runtime based on the actual object type, not the declared type of the reference variable.
Consider the following example:
In this example, the variable animal
is of type Animal
, but it actually refers to a Dog
object. When the makeSound()
method is called, the compiler doesn't know the exact type of the object at compile time. It's only at runtime when the JVM determines the actual object type (Dog
) that the correct implementation of the makeSound()
method is invoked, resulting in the output Dog barks
. This is the essence of runtime polymorphism.
Static Polymorphism (Compile-Time Binding)
In contrast to runtime polymorphism, static polymorphism (method overloading) is resolved at compile time. The compiler determines which method to call based on the argument types passed to the method. This is less dynamic than runtime polymorphism but still offers flexibility in method usage.
By understanding these concepts and their interplay, you can harness the power of inheritance and polymorphism to create flexible, reusable, and maintainable Java applications.
The Power Duo: Inheritance and Polymorphism Working Together
Inheritance and polymorphism are not isolated concepts; they work in tandem to create robust and flexible object-oriented systems.
Inheritance as a Foundation for Polymorphism
Inheritance provides the structural framework for polymorphism to shine. By establishing a class hierarchy, inheritance creates a foundation for different objects to share commonalities and specific differences. This hierarchical structure is essential for enabling polymorphic behavior.
Consider a simple example:
In this example:
- The
Animal
class serves as the superclass, defining a common behavior for all animals through themakeSound()
method. - The
Dog
andCat
classes inherit fromAnimal
, providing specific implementations of themakeSound()
method through method overriding. - The
main
method demonstrates polymorphism. The variablesanimal1
andanimal2
are of typeAnimal
, but they hold objects of different concrete types (Dog
andCat
). When themakeSound()
method is called, the appropriate implementation based on the object's actual type is executed at runtime.
Real-World Examples: Bringing Inheritance and Polymorphism to Life
Let's explore how inheritance and polymorphism are used in practical scenarios:
Graphical User Interfaces (GUIs):
- A base
Component
class defines common properties and behaviors for all GUI elements (buttons, labels, text fields, etc.). - Subclasses like
Button
,Label
,TextField
inherit fromComponent
and add specific properties and methods. - Polymorphism allows you to create a generic
render()
method in a container component that can handle different types of components without knowing their exact type.
E-commerce Applications:
- A base
Product
class defines common product attributes (name, price, description). - Subclasses like
Book
,Electronics
, andClothing
inherit fromProduct
and add specific properties and methods relevant to their respective product types. - Polymorphism enables you to create a shopping cart that can hold products of different types, treating them uniformly through a common
addToCart()
method.
Shape Drawing Application:
- A base
Shape
class defines properties like color and position. - Subclasses like
Circle
,Rectangle
, andTriangle
inherit fromShape
and provide specific calculations for area, perimeter, and drawing methods. - Polymorphism allows you to create a list of shapes and iterate through it, calling the
draw()
method on each shape, regardless of its concrete type.
By understanding and applying inheritance and polymorphism effectively, you can create more flexible, maintainable, and scalable Java applications.