Exploring Java Collections Framework: Lists, Sets, Maps, and More
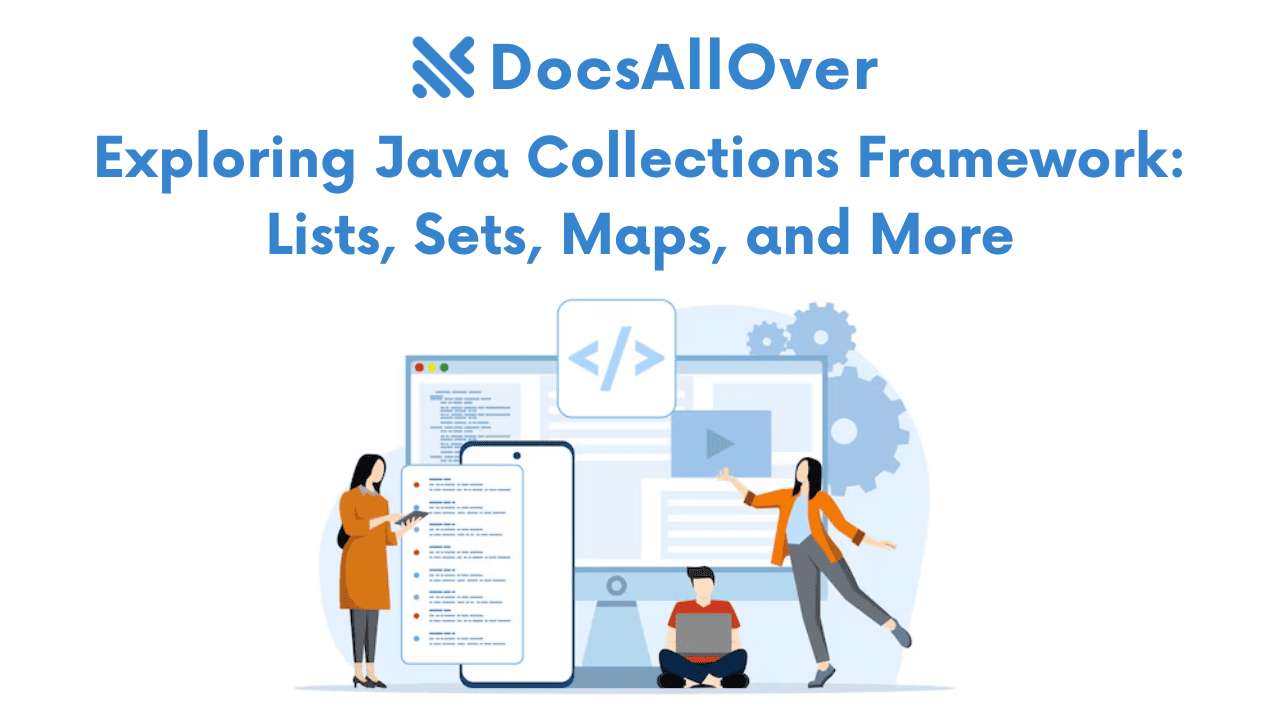
What is the Java Collections Framework?
The Java Collections Framework (JCF) is a set of interfaces and classes that provide data structures for storing and manipulating collections of objects. It is an essential part of the Java programming language, offering efficient and flexible ways to manage data.
Importance of Understanding Collections in Java
Understanding collections is crucial for Java developers as they are used extensively in various applications. By mastering the JCF, you can write more efficient, organized, and maintainable code.
Overview of Different Types of Collections
The JCF provides several types of collections, each with its own characteristics and use cases:
- Lists: Ordered collections that allow duplicate elements. Examples include ArrayList and LinkedList.
- Sets: Unordered collections that do not allow duplicate elements. Examples include HashSet and TreeSet.
- Maps: Store key-value pairs. Examples include HashMap and TreeMap.
- Queues: Ordered collections that follow the FIFO (First-In-First-Out) principle.
- Stacks: Ordered collections that follow the LIFO (Last-In-First-Out) principle.
- Deques: Double-ended queues that allow elements to be added or removed from both ends.
Example:
In the following sections, we will explore each type of collection in more detail.
Lists
Definition and Characteristics of Lists
Lists are ordered collections of objects in Java. They allow duplicate elements and provide methods for accessing, adding, removing, and modifying elements based on their index.
ArrayList vs. LinkedList
- ArrayList:
- Uses an array-based implementation.
- Efficient for random access operations (e.g.,
get
,set
). - Less efficient for insertions and deletions at the beginning or middle of the list.
- LinkedList:
- Uses a doubly-linked list implementation.
- Efficient for insertions and deletions at the beginning or middle of the list.
- Less efficient for random access operations.
Example:
Common List Operations
add(element)
: Adds an element to the end of the list.add(index, element)
: Inserts an element at a specific index.remove(index)
: Removes the element at a specific index.remove(object)
: Removes the first occurrence of the specified element.get(index)
: Retrieves the element at a specific index.set(index, element)
: Replaces the element at a specific index with a new element.size()
: Returns the number of elements in the list.isEmpty()
: Checks if the list is empty.contains(element)
: Checks if the list contains a specific element.
Use Cases for Lists
Lists are commonly used in various scenarios, such as:
- Storing ordered sequences of elements: For example, a list of products in a shopping cart or a list of tasks in a to-do list.
- Implementing stacks and queues: Lists can be used to implement stacks (LIFO) and queues (FIFO).
- Iterating over elements: Lists can be easily iterated over using a
for
loop or an iterator.
Sets
Definition and Characteristics of Sets
Sets are unordered collections of objects in Java. They do not allow duplicate elements. Sets are primarily used for membership testing and removing duplicates from a collection.
HashSet vs. TreeSet
- HashSet:
- Uses a hash table implementation.
- Elements are not stored in any particular order.
- Provides fast lookup and insertion operations.
- TreeSet:
- Uses a red-black tree implementation.
- Elements are stored in ascending order according to their natural ordering or a custom comparator.
- Provides efficient searching, insertion, and deletion operations.
Example:
Common Set Operations
add(element)
: Adds an element to the set.remove(element)
: Removes the specified element from the set.contains(element)
: Checks if the set contains a specific element.size()
: Returns the number of elements in the set.isEmpty()
: Checks if the set is empty.iterator()
: Returns an iterator to iterate over the elements in the set.
Use Cases for Sets
Sets are commonly used in various scenarios, such as:
- Removing duplicates: Sets can be used to remove duplicate elements from a collection.
- Membership testing: Checking if a specific element is present in a set.
- Implementing set operations: Performing set operations like union, intersection, and difference.
- Implementing data structures: Sets can be used as building blocks for other data structures like graphs and maps.
Maps
Definition and Characteristics of Maps
Maps are data structures that store key-value pairs. Each key is associated with a corresponding value. Maps are used to efficiently retrieve values based on their keys.
HashMap vs. TreeMap
- HashMap:
- Uses a hash table implementation.
- Elements are not stored in any particular order.
- Provides fast lookup and insertion operations.
- Does not guarantee the order of elements.
- TreeMap:
- Uses a red-black tree implementation.
- Elements are stored in ascending order according to their natural ordering or a custom comparator.
- Provides efficient searching, insertion, and deletion operations.
- Guarantees the order of elements.
Example:
Common Map Operations
put(key, value)
: Inserts a key-value pair into the map.get(key)
: Retrieves the value associated with a given key.remove(key)
: Removes the key-value pair associated with a given key.containsKey(key)
: Checks if the map contains a specific key.containsValue(value)
: Checks if the map contains a specific value.size()
: Returns the number of key-value pairs in the map.keySet()
: Returns a set of all keys in the map.entrySet()
: Returns a set of all entries in the map.
Use Cases for Maps
Maps are commonly used in various scenarios, such as:
- Storing key-value pairs: For example, storing user preferences, configuration settings, or data from databases.
- Implementing dictionaries: Maps can be used to create dictionaries that map words to their definitions or meanings.
- Caching data: Maps can be used to store frequently accessed data in memory for faster retrieval.
- Implementing custom data structures: Maps can be used as building blocks for other data structures, such as graphs or trees.
Other Collections
Queue
- Definition: A queue is a linear data structure that follows the FIFO (First-In-First-Out) principle. Elements are added to the rear of the queue and removed from the front.
- Implementation: Java provides
Queue
interface and its implementations likeLinkedList
andPriorityQueue
.
Example:
Stack
- Definition: A stack is a linear data structure that follows the LIFO (Last-In-First-Out) principle. Elements are added and removed from the top.
- Implementation: Java provides
Stack
class, which extendsVector
.
Example:
Deque (Double-Ended Queue)
- Definition: A deque is a generalized queue that supports adding and removing elements from both ends.
- Implementation: Java provides
Deque
interface and its implementations likeLinkedList
andArrayDeque
.
Example:
These are some of the other commonly used collections in Java. The choice of collection depends on the specific requirements of your application.
Best Practices for Using Collections
Choosing the Right Collection for Your Needs
- List vs. Set: If you need to maintain the order of elements and allow duplicates, use a list. If you need to ensure uniqueness of elements and don't care about order, use a set.
- ArrayList vs. LinkedList: ArrayList is generally more efficient for random access operations, while LinkedList is more efficient for insertions and deletions at the beginning or middle of the list.
- HashMap vs. TreeMap: HashMap is faster for most operations, but TreeMap maintains elements in sorted order. Use TreeMap if you need to iterate over elements in sorted order.
- Queue vs. Stack: Use a queue for FIFO (First-In-First-Out) order, and use a stack for LIFO (Last-In-First-Out) order.
Efficient Use of Collections
- Avoid unnecessary iterations: If possible, perform operations on the entire collection rather than iterating over each element individually.
- Use enhanced for loops: Use enhanced for loops for iterating over collections, as they are more concise and readable.
- Consider generics: Use generics to improve type safety and reduce the likelihood of runtime errors.
- Be aware of performance implications: Some collections may have performance implications, especially for large datasets.
Avoiding Common Pitfalls
- NullPointerException: Avoid using
null
values in collections. Usenull
checks or optional types to prevent this exception. - ConcurrentModificationException: Be careful when modifying a collection while iterating over it. Use a copy of the collection or use a concurrent collection if necessary.
- Infinite loops: Avoid infinite loops when iterating over collections, especially if you're modifying the collection within the loop.
- Inefficient algorithms: Choose appropriate algorithms for the tasks you're performing. For example, using a linear search on a sorted list is less efficient than using binary search.
Example:
By following these best practices, you can effectively use collections in your Java applications and avoid common pitfalls.