Java Exception Handling: Catching Errors Before They Crash Your Code
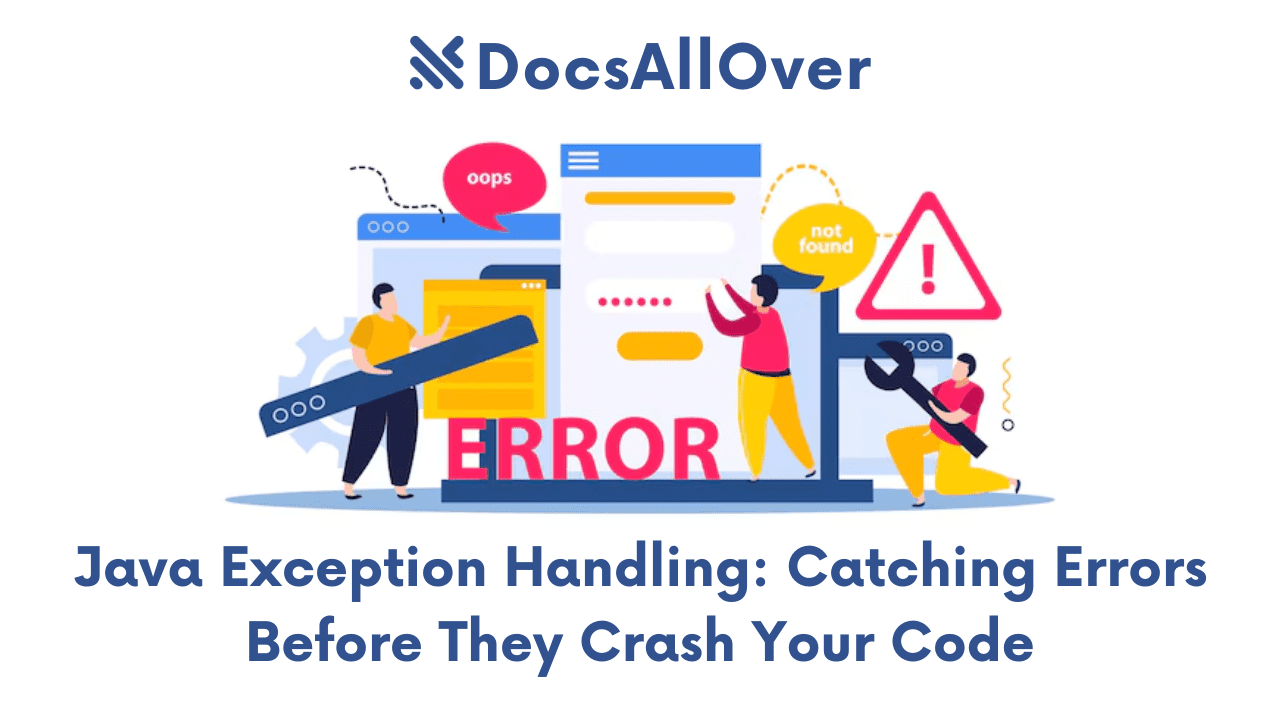
What are Exceptions in Java?
Exceptions in Java are objects that represent errors or abnormal situations that occur during program execution. When an error occurs, the Java runtime system throws an exception object, which can be caught and handled by your code.
Importance of Exception Handling
Proper exception handling is crucial for writing robust and reliable Java applications. It prevents unexpected program termination and helps to maintain the integrity of your code. By anticipating potential errors and handling them gracefully, you can provide a better user experience and avoid costly downtime.
Benefits of Proper Exception Handling
- Improved code reliability: By handling exceptions, you can prevent your program from crashing unexpectedly.
- Enhanced user experience: Graceful error handling can provide informative messages to users and avoid frustrating crashes.
- Easier debugging: Exceptions can help pinpoint the root cause of errors, making debugging easier.
- Maintainability: Well-handled exceptions make your code more maintainable and easier to understand.
In the next section, we'll delve deeper into the different types of exceptions in Java.
Types of Exceptions
Java exceptions are broadly classified into two categories: checked exceptions and unchecked exceptions.
Checked Exceptions
- Definition: These are exceptions that the compiler checks for at compile time. You must either handle them using a
try-catch
block or declare them in the method signature using thethrows
keyword. - Examples:
IOException
,SQLException
,ClassNotFoundException
Unchecked Exceptions
- Definition: These exceptions are not checked at compile time. You can choose to handle them or let them propagate up the call stack.
- Examples:
NullPointerException
,ArithmeticException
,ArrayIndexOutOfBoundsException
Custom Exceptions
You can create your own custom exceptions to represent specific error conditions in your application. Custom exceptions should extend the Exception
class or one of its subclasses.
Example:
By understanding the different types of exceptions, you can write more robust and maintainable Java code.
Exception Handling Mechanisms
try-catch
Blocks
The try-catch
block is the primary mechanism for handling exceptions in Java. It consists of a try
block that contains the code that might throw an exception, and one or more catch
blocks to handle specific types of exceptions.
Example:
finally
Block
The finally
block is optional and is executed regardless of whether an exception is thrown or caught. It's often used for cleanup tasks, such as closing files or database connections.
Example:
throw
and throws
Keywords
throw
: Used to explicitly throw an exception.throws
: Used in method signatures to declare that a method might throw a specific type of exception.
Example:
By understanding these mechanisms, you can effectively handle exceptions in your Java code and prevent unexpected program termination.
Best Practices for Java Exception Handling
Catching Specific Exceptions
- Avoid generic
catch
blocks: Instead of catchingException
, catch specific exception types to provide more targeted handling. - Use a hierarchy of
catch
blocks: Catch more specific exceptions first, then handle more general exceptions.
Example:
Using Meaningful Exception Messages
- Provide informative messages: Include details about the error, such as the cause and context.
- Use a consistent format: Use a consistent format for exception messages to make them easier to understand.
Example:
Avoiding Empty catch
Blocks
- Empty
catch
blocks: Avoid emptycatch
blocks, as they can hide potential errors. - Log or rethrow exceptions: If you don't want to handle an exception, log it or rethrow it for further processing.
Proper Logging and Error Reporting
- Log exceptions: Use a logging framework (e.g., Log4j, SLF4J) to log exceptions and their stack traces.
- Report errors to users: Provide informative error messages to users, but avoid exposing sensitive information.
Using Custom Exceptions
- Create custom exceptions: Define custom exceptions to represent specific error conditions in your application.
- Provide meaningful messages: Include relevant information in the exception message.
Example:
By following these best practices, you can write more robust and maintainable Java code that effectively handles exceptions.
Common Exceptions and Their Uses
NullPointerException
- When thrown: When you attempt to access a member of an object that is
null
. - Example:
IndexOutOfBoundsException
- When thrown: When you try to access an array element using an invalid index.
- Example:
NumberFormatException
- When thrown: When a string cannot be parsed into a numerical value.
- Example:
IOException
- When thrown: When an input/output error occurs, such as when reading from or writing to a file.
- Example:
SQLException
- When thrown: When a database-related error occurs, such as when trying to execute an invalid SQL query or when the database is unavailable.
- Example:
Creating Custom Exceptions
Custom exceptions allow you to represent specific error conditions in your application, providing more informative messages and making your code easier to understand and maintain.
Defining Custom Exception Classes
To create a custom exception, you need to define a class that extends the Exception
class or one of its subclasses. You can provide a constructor to initialize the exception with a message.
Example:
Throwing Custom Exceptions
You can throw a custom exception using the throw
keyword.
Example:
By creating custom exceptions, you can provide more specific information about errors that occur in your application, making it easier to debug and fix issues.
Advanced Exception Handling Techniques
Exception Chaining
Exception chaining allows you to link multiple exceptions together, providing a more complete error context. This can be helpful when one exception is caused by another.
Example:
In this example, if an IOException
occurs, it is wrapped in a RuntimeException
and rethrown, preserving the original exception as the cause.
Declared Exceptions and Checked Exceptions
- Declared Exceptions: When a method might throw a checked exception, you must either handle it using a
try-catch
block or declare it in the method signature using thethrows
keyword. - Checked Exceptions: Checked exceptions are typically used for recoverable errors that can be handled gracefully.
Example:
In this example, the readFile
method declares that it might throw an IOException
, so the caller must handle it or declare it in their own method signature.