Java's Building Blocks: Understanding Variables and Datatypes
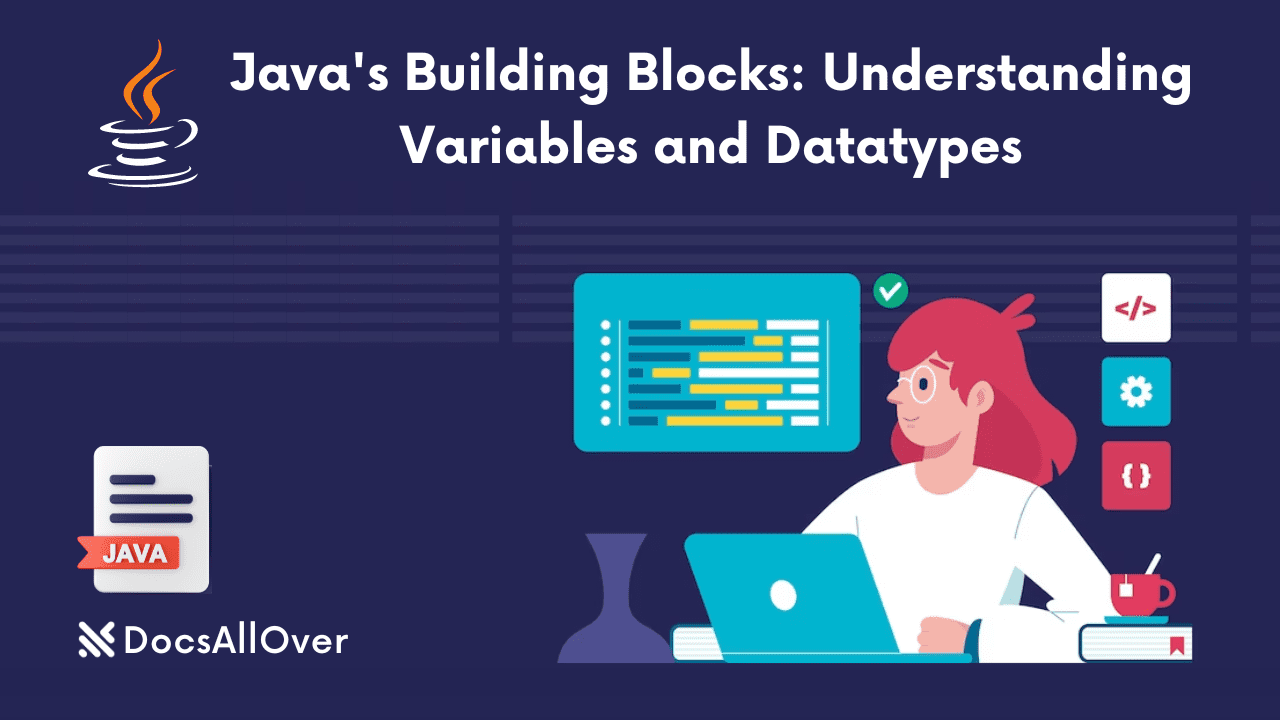
Java's Code Kitchen: Introducing Variables and Datatypes as the Basic Ingredients
Imagine stepping into a kitchen for the first time. Before you whip up culinary masterpieces, you need to understand the essentials – flour, eggs, milk, these are the building blocks of delicious creations. In the world of Java programming, variables and datatypes play a similar role. They are the basic ingredients that allow you to craft powerful and efficient programs.
So, how do these magic beans of Java work? Let's delve into the fascinating world of variables and datatypes:
From Words to Data:
Think of a variable as a named container that holds information in your program. Just like a chef labels jars "flour" or "sugar," you give names to variables to store different types of data like numbers, text, or even complex objects. For example, a variable named "age" might hold the value 25, while another called "username" could store "JavaMaster." These variables act as your memory slots, allowing you to access and manipulate the information they hold throughout your program.
Mastering the Data Types:
But not all ingredients are created equal. Just like you wouldn't use flour for soup, Java has different datatypes to specify the kind of information each variable can hold. Think of them as labels that tell Java what kind of data to expect:
- Primitive Datatypes: These are the fundamental building blocks, like whole numbers (int), decimals (double), characters (char), and true/false values (boolean). They are efficient and offer basic operations like addition or comparison.
- Non-Primitive Datatypes: These are more complex entities, like strings (sequences of characters), arrays (collections of data), and classes (reusable blueprints for objects). They provide advanced functionality and structure to your programs.
Choosing the right datatype is crucial. Using an "int" to store a username would cause errors, just like using flour for soup might lead to a disappointing outcome. By understanding and utilizing different datatypes, you ensure your programs run smoothly and efficiently.
Beyond the Basics:
This is just a glimpse into the exciting world of variables and datatypes. As you progress through your Java journey, you'll encounter more nuances like variable scope (where they're accessible), type casting (converting between datatypes), and advanced object-oriented concepts. But with a solid foundation in these basics, you'll be well-equipped to build your own culinary masterpieces in the Java kitchen.
Variables: Mastering the Language of Java Through Names, Scope, and Modifiers
We've established variables as the fundamental containers of information in your Java program. Now, let's delve deeper into the art of crafting meaningful variables, understanding their whereabouts within your code, and exploring tools to manipulate their behavior.
-
1. Naming the Game: Choosing Good Variable Names for Readability and Clarity
Ever received a cryptic recipe with ingredients like "X" and "Y"? Just as clear labels enhance culinary experiences, meaningful variable names elevate your code. Follow these tips to become a Java naming master:
- Be descriptive: Use names that accurately reflect the data stored, like "customerName" instead of "c."
- Avoid abbreviations: Unless widely understood, "custAge" might be less clear than "customerAge."
- Use consistent conventions: Stick to camelCase or snake_case for uniformity within your project.
- Stay concise: Long, convoluted names can clutter your code and hinder readability.
Remember, good names are self-documenting, saving you and others from deciphering cryptic symbols later. Think of them as mini roadmaps within your code, guiding everyone towards understanding and appreciation.
-
2. Scope Matters: Where Your Variables Live and Breathe in Your Code
Imagine ingredients magically teleporting around the kitchen! In Java, variables have their own "spaces" called scope, defining where they are accessible and how long they exist. Master these zones:
- Local: These variables live within a specific block of code, like a method or loop, and disappear when the block finishes. Think of them as borrowed ingredients, used temporarily for a specific recipe.
- Instance: These variables belong to objects and live as long as the object exists. Imagine them as personal pantry items each object carries around.
- Static: These variables are shared across all instances of a class and persist throughout the program's execution. Think of them as the communal spices on the kitchen shelf, accessible to everyone.
Understanding scope ensures your variables are used effectively and prevents unexpected access issues. It's like knowing where your ingredients are stored, enabling smooth and efficient cooking.
-
3. Initialization Techniques: Giving Your Variables a Starting Value
Ingredients left without preparation can lead to messy results. Similarly, variables need initial values to work with. Explore these initialization techniques:
- Explicit assignment: Declare the variable and assign a value directly, like
int age = 25;
. - Default values: Some datatypes have predefined default values, like 0 for integers.
- Constructor initialization: Object constructors can assign initial values to instance variables.
Choosing the right initialization technique ensures your variables start with the appropriate data, setting the stage for a well-coordinated recipe. Think of it as prepping your ingredients before diving into the actual cooking process.
- Explicit assignment: Declare the variable and assign a value directly, like
-
4. Variable Modifiers: Adding Flexibility with final, static, and more
Just as certain ingredients require special handling, Java offers modifiers to add flavor to your variables:
- final: Makes a variable constant, preventing its value from being changed, like a fixed spice blend.
- static: Declares a variable to be shared across all instances of a class, like a communal measuring cup.
- volatile: Ensures changes to a variable are immediately visible across threads, like a constantly updated timer.
Using modifiers wisely adds flexibility and control to your code. Think of them as specialized tools that enhance the versatility of your ingredients, allowing you to create even more complex and delicious dishes.
By mastering these aspects of variables – naming, scope, initialization, and modifiers – you'll elevate your code from a messy kitchen to a well-organized culinary masterpiece. Remember, the more conscious you are of your variables, the smoother and more efficient your Java programs will be. So, explore, experiment, and keep refining your naming skills, scoping techniques, and modifier usage to become a true Java code chef!
Exploring Datatypes: Unlocking the Flavorful Ingredients of Java
We've established variables as the containers, but now it's time to delve into the delicious contents themselves – the datatypes! These are the diverse ingredients that add texture, sweetness, and complexity to your Java programs. Let's explore some key datatypes, their unique characteristics, and how to use them effectively:
-
Primitive Powerhouse: Numbers, Booleans, and Characters - The Basic Types
Think of these as the foundational spices in your Java pantry:
- Numbers: Integers (int) for whole numbers, doubles for decimals, floats for lightweight decimals, and more – these handle numeric calculations, comparisons, and logical operations. Imagine them like salt, sugar, and pepper, adding basic flavors and structure to your code.
- Booleans: True or false values, like a light switch – used for controlling program flow and conditional statements. Think of them as the on/off switch that determines how your program reacts.
- Characters: Individual characters like letters, numbers, or symbols – used for text manipulation and building strings. Imagine them as individual spices that add specific notes and flavors to your text creations.
These basic datatypes are the workhorses of Java, providing efficient and versatile tools for building the core functionality of your programs.
- Numbers: Integers (int) for whole numbers, doubles for decimals, floats for lightweight decimals, and more – these handle numeric calculations, comparisons, and logical operations. Imagine them like salt, sugar, and pepper, adding basic flavors and structure to your code.
-
String Secrets: Understanding String Concatenation and Manipulation
Strings, like magical dough, can be kneaded, stretched, and combined to create diverse textual creations. Here are some key string secrets:
- Concatenation: Joining strings together using the `+` operator, like adding toppings to a pizza.
- String methods: Built-in functions like `toUpperCase()` or `substring()` to modify and analyze string content, like shaping the dough into different forms.
Strings offer immense flexibility for building user interfaces, processing text data, and even creating dynamic messages within your programs.
- Concatenation: Joining strings together using the `+` operator, like adding toppings to a pizza.
-
Reference Types Revealed: Objects and Arrays as Complex Data Structures
Beyond the basic spices, imagine complex dishes built with ingredients like vegetables and meats. These are like Java's reference types:
- Objects: Encapsulated collections of data and methods, representing real-world entities like users or products. Think of them as pre-prepared ingredients with specific properties and functions.
- Arrays: Ordered collections of data elements of the same type, like a row of neatly chopped vegetables. They allow efficient storage and manipulation of multiple values.
- Objects: Encapsulated collections of data and methods, representing real-world entities like users or products. Think of them as pre-prepared ingredients with specific properties and functions.
-
Casting Spells: Converting Between Datatypes for Flexibility
Imagine transforming ingredients from one form to another, like melting butter or grinding spices. Java allows type casting, where you convert a value from one datatype to another:
Casting allows you to bridge between different datatypes, adding flexibility and enabling diverse operations within your program.
By mastering these diverse datatypes and their functionalities, you'll unlock the full potential of Java's flavor palette. Remember, the more you experiment with different ingredients and techniques, the more delicious and sophisticated your Java programs will become! So, keep exploring, keep learning, and keep creating culinary.
Putting it all Together: Building Your Java Culinary Masterpiece
We've explored the pantry of Java's variables and datatypes, learning the properties and flavors of each ingredient. Now, let's get cooking! In this final chapter, we'll bring all your newfound knowledge together, crafting code that sings with efficiency and clarity.
1. Variable Declarations in Action: Writing Code with Your New Knowledge
Ready to put your learning into practice? Let's declare some variables and bake a simple program:
This code snippet showcases variable declaration, assignment, basic operations, and string concatenation. As you write more code, remember:
- Choose meaningful names: "age" is clearer than "x".
- Declare variables where needed: Avoid unnecessary clutter.
- Use appropriate datatypes: Don't store names in integers.
- Initialize variables thoughtfully: Assign relevant starting values.
By following these principles, you'll write code that's both efficient and understandable.
2. Real-World Examples: How Variables and Datatypes are Used in Different Applications
Java's building blocks power diverse applications! Imagine:
- E-commerce websites: Using arrays to store product information, string operations for search filters, and variables to track user accounts.
- Games: Utilizing integers for scores, floats for character positions, and objects for enemies and power-ups.
- Scientific simulations: Employing arrays for complex data sets, calculations with numbers, and string manipulation for analysis output.
Understanding how variables and datatypes are used in various contexts broadens your perspective and showcases their real-world significance.
3. Common Pitfalls and Debugging Tips: Avoiding Errors and Maintaining Clean Code
Even seasoned chefs encounter burnt batches! Java has its own challenges:
- Type mismatch errors: Trying to add strings and numbers. Double-check your variable declarations and operations.
- Uninitialised variables: Using them before assigning a value. Ensure proper initialization in relevant blocks.
- Logic errors: Unexpected program behavior. Use print statements and debuggers to trace the flow of execution.
Remember, error messages are clues, not punishments. Learn from them, adapt your code, and keep your program running smoothly.
4. Advanced Concepts: Exploring References, Null Pointers, and Object-Oriented Approaches
As you advance in your Java journey, you'll encounter deeper concepts:
- References: Variables hold references to objects, not the objects themselves. Understand this distinction for efficient memory management.
- Null pointers: Trying to access non-existent objects. Implement null checks to avoid crashes.
- Object-oriented programming: Organize your code with classes and objects, leveraging inheritance and polymorphism.
So, there you have it! From naming your ingredients (variables) to understanding their properties (datatypes), you've equipped yourself with the essential tools to conquer any Java recipe. Remember, just like a master chef, the more you practice and experiment, the more flavorful and efficient your code will become. So, roll up your sleeves, fire up your compiler, and start cooking up some amazing Java programs! Happy coding!