Working with Strings in Java: Effective String Manipulation Techniques
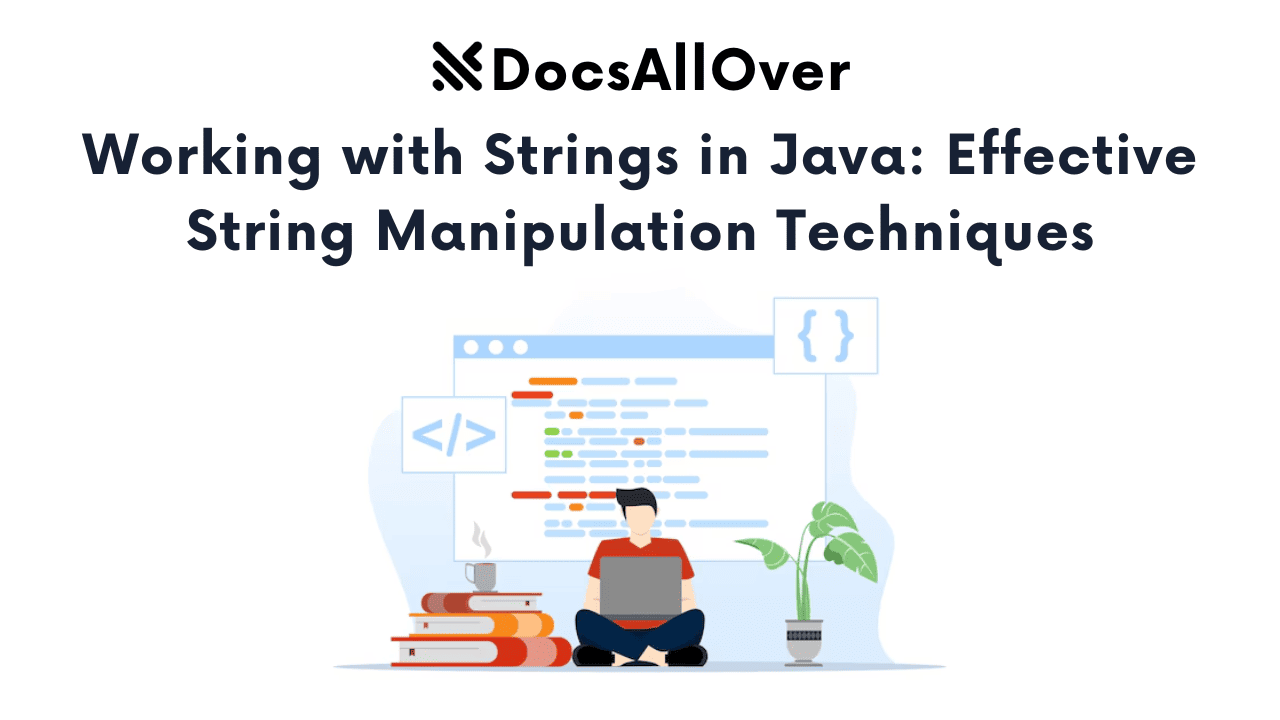
What is a String in Java?
A String in Java represents a sequence of characters. It's an immutable object, meaning once a String object is created, its value cannot be changed.
Creating and Initializing Strings
You can create Strings in Java in several ways:
- Using String Literals:
- Using the
new
keyword:
Basic String Operations
length()
: Returns the length of the string.
charAt(index)
: Returns the character at the specified index.
indexOf(str)
: Returns the index of the first occurrence of the specified substring.
lastIndexOf(str)
: Returns the index of the last occurrence of the specified substring.
String Concatenation
String concatenation is the process of combining two or more strings. Java provides several methods for string concatenation:
Using the +
Operator
The +
operator is a simple way to concatenate strings:
However, for frequent concatenations, this approach can be inefficient, especially in loops.
Using StringBuilder
and StringBuffer
StringBuilder
and StringBuffer
are classes designed for efficient string manipulation. They offer methods to append, insert, and delete characters.
StringBuilder:
- Not thread-safe
- Faster than
StringBuffer
for single-threaded environments
StringBuffer:
- Thread-safe
- Slower than
StringBuilder
but safe for multi-threaded environments
Performance Considerations
- StringBuilder and StringBuffer are generally more efficient than the
+
operator for frequent concatenations, especially in loops. - String immutability: Remember that Strings are immutable. When you concatenate strings, a new String object is created. This can lead to unnecessary object creation and garbage collection overhead.
String Manipulation
Substring Extraction
To extract a portion of a string, use the substring()
method:
Character and Code Point Manipulation
charAt(index)
: Returns the character at the specified index.codePointAt(index)
: Returns the Unicode code point at the specified index.toCharArray()
: Converts the string to a character array.
String Comparison
equals(str)
: Compares the content of two strings.equalsIgnoreCase(str)
: Compares the content of two strings, ignoring case.compareTo(str)
: Compares two strings lexicographically.
String Searching and Replacing
indexOf(str)
: Returns the index of the first occurrence of the specified substring.lastIndexOf(str)
: Returns the index of the last occurrence of the specified substring.contains(str)
: Checks if a string contains a specific substring.replace(oldChar, newChar)
: Replaces all occurrences of a character with another.replaceAll(regex, replacement)
: Replaces all occurrences of a regular expression pattern.replaceFirst(regex, replacement)
: Replaces the first occurrence of a regular expression pattern.
Regular Expressions
Regular expressions are powerful tools for pattern matching and text manipulation. They provide a concise and flexible way to search, replace, and extract information from text.
Introduction to Regular Expressions
A regular expression is a sequence of characters that defines a search pattern. It can be used to match specific patterns within a text string.
Pattern Matching and Searching
- Basic Patterns:
.
matches any single character.\d
matches a digit.\w
matches a word character (alphanumeric or underscore).\s
matches a whitespace character.
- Quantifiers:
+
: Matches one or more occurrences of the preceding element.*
: Matches zero or more occurrences of the preceding element.?
: Matches zero or one occurrence of the preceding element.{n}
: Matches exactly n occurrences.{n,}
: Matches at least n occurrences.{n,m}
: Matches at least n and at most m occurrences.
- Character Classes:
[abc]
: Matches any character within the brackets.[^abc]
: Matches any character not within the brackets.[a-z]
: Matches any lowercase letter from a to z.
String Splitting and Tokenization
split(regex)
: Splits a string into an array of substrings based on a regular expression pattern.
Validation and Data Extraction
- Validating Email Addresses:
String emailRegex = "^[\\w-\\.]+@([\\w-]+\\.)+[\\w-]{2,4}$";
- Extracting Phone Numbers:
String phoneRegex = "\\d{3}-\\d{3}-\\d{4}";
String Formatting
String formatting allows you to create formatted strings by inserting values into placeholders within a template string. Java provides several methods for string formatting:
Using String.format()
The String.format()
method is versatile and allows you to format various data types, including numbers, dates, and strings.
In this example:
%s
is a placeholder for a string.%d
is a placeholder for an integer.%.2f
is a placeholder for a floating-point number with two decimal places.
Using printf()
The printf()
method is similar to String.format()
, but it prints the formatted string directly to the console:
Formatting Numbers, Dates, and Other Data Types
- Numbers:
- Dates:
- Custom Formatting: You can use custom format specifiers to control the exact formatting of numbers, dates, and other data types.
Advanced String Techniques
String Immutability
In Java, Strings are immutable, meaning their contents cannot be changed once they are created. When you perform any operation on a String, a new String object is created.
String Pooling
The Java Virtual Machine (JVM) maintains a String pool to optimize memory usage. When you create a String literal, the JVM checks the pool for an existing identical String. If found, it returns a reference to the existing object instead of creating a new one.
Performance Optimization Tips
- Use
StringBuilder
orStringBuffer
for frequent concatenations. - Avoid unnecessary String object creation.
- Use
intern()
to explicitly add a String to the pool. - Be mindful of regular expression performance, especially when using complex patterns.
- Consider using libraries like Apache Commons Lang for advanced string manipulation.
By understanding these advanced string techniques, you can write more efficient and optimized Java code.