Building a Modern Frontend Tech Stack: Frameworks, Libraries, and Tools
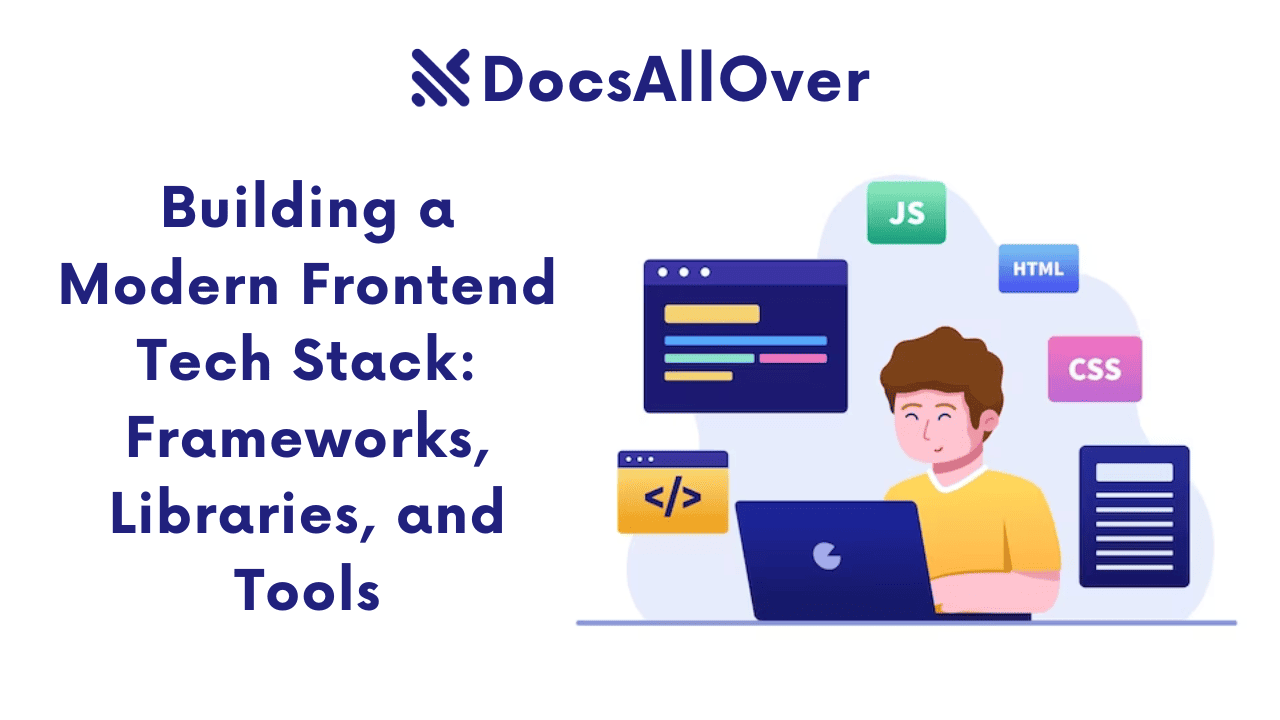
What is a Frontend Tech Stack?
A frontend tech stack refers to the combination of tools, technologies, and frameworks used to build the client-side of web applications. It's the part of the application that users interact with directly.
Why a Modern Frontend Stack Matters
A well-chosen frontend tech stack can significantly impact the performance, user experience, and maintainability of your web application. A modern stack should:
- Enhance User Experience: Provide smooth, interactive, and visually appealing interfaces.
- Improve Performance: Optimize load times and overall performance.
- Facilitate Development: Streamline the development process with efficient tools and frameworks.
- Enhance Security: Protect against common web vulnerabilities.
Core Technologies: HTML, CSS, and JavaScript
While modern web development relies on various frameworks and libraries, HTML, CSS, and JavaScript remain the fundamental building blocks:
- HTML (HyperText Markup Language): Defines the structure and content of a webpage.
- CSS (Cascading Style Sheets): Styles the visual presentation of HTML elements.
- JavaScript: Adds interactivity and dynamic behavior to web pages.
A strong foundation in these core technologies is essential for building robust and efficient frontend applications.
Frontend Frameworks
Frontend frameworks provide structured approaches to building complex web applications. Let's explore some of the most popular ones:
React

- Component-Based Architecture: React breaks down user interfaces into reusable components, making it easier to build and maintain complex applications.
- Virtual DOM: React uses a virtual DOM to efficiently update the actual DOM, leading to improved performance.
- JSX: A syntax extension for JavaScript that allows you to write HTML-like structures within JavaScript code.
- State Management:
- Redux: A popular state management library for managing global application state.
- Context API: A built-in React feature for sharing state across components.
- Routing: React Router is a powerful routing library for handling navigation and URL changes within React applications.
Angular

- TypeScript Support: Angular heavily relies on TypeScript, a typed superset of JavaScript, for improved code quality and maintainability.
- Component-Based Architecture: Similar to React, Angular uses a component-based approach to build modular and reusable UI components.
- Dependency Injection: Angular provides a built-in dependency injection framework for managing dependencies between components.
- Angular CLI: A command-line interface for generating components, services, and other project files, streamlining the development process.
Vue.js

- Progressive Framework: Vue.js can be used incrementally, making it suitable for both small and large-scale projects.
- Component-Based Architecture: Vue.js uses a component-based approach to organize UI components.
- Vuex: A state management library for Vue.js applications.
- Vue Router: A routing library for Vue.js applications.
Frontend Libraries
In addition to frameworks, frontend libraries can significantly enhance the development process and improve application performance. Here are some popular libraries:
Redux
- Centralized State Management: Redux provides a centralized store to manage the global state of your application.
- Predictable State Changes: Actions and reducers ensure a clear and predictable flow of state updates.
- Time Travel Debugging: Redux DevTools allow you to inspect the history of state changes.
Vuex
- State Management for Vue.js: Vuex is specifically designed for Vue.js applications and integrates seamlessly with Vue's component system.
- Modular State Management: Organizes state into modules to improve code organization and maintainability.
React Router
- Declarative Routing: Defines routes using a declarative syntax.
- Nested Routes: Creates hierarchical routing structures.
- Programmatic Navigation: Allows you to navigate to specific routes programmatically.
- Code Splitting: Optimizes performance by loading code on demand.
Axios
- Promise-Based HTTP Client: Makes it easy to make HTTP requests to APIs.
- Interceptors: Allows you to intercept requests and responses to add custom logic.
- Automatic JSON Data Transformation: Handles JSON data serialization and deserialization.
Frontend Build Tools
Frontend build tools automate tasks like bundling, minification, and optimization, improving the performance and efficiency of your web applications.
Webpack
- Powerful and Customizable: Webpack offers extensive configuration options and a rich ecosystem of plugins.
- Module Bundling: Combines multiple JavaScript modules into a single file.
- Code Splitting: Divides code into smaller chunks to improve initial load time.
- Asset Optimization: Minifies and compresses assets like CSS, JavaScript, and images.
Parcel
- Zero-Configuration: Parcel requires minimal configuration, making it easy to get started.
- Fast Build Times: Parcel is known for its fast build times.
- Built-in Optimization: Automatically handles tasks like minification, compression, and code splitting.
Rollup
- Tree Shaking: Removes unused code from your bundles.
- Code Splitting: Divides code into smaller chunks.
- Optimized for Libraries: Well-suited for building libraries and frameworks.
Frontend Testing Tools
Testing is an essential part of the development process to ensure code quality and prevent regressions. Here are some popular frontend testing tools:
Jest
- Flexible Testing Framework: Jest is a versatile testing framework that can be used to test JavaScript code, React components, and more.
- Snapshot Testing: Automatically generates snapshots of UI components and compares them to previous versions to detect changes.
- Mock Functions: Allows you to mock functions and control their behavior in tests.
React Testing Library
- Focused on User Experience: This library encourages testing React components from a user's perspective.
- Simulates User Interactions: You can test how components respond to user input and events.
- Encourages Best Practices: Encourages writing tests that are focused on the component's behavior, rather than its implementation details.
Cypress
- End-to-End Testing: Cypress allows you to test the entire user flow of your application, including interactions with the browser and network requests.
- Time Travel Debugging: You can step through test execution to understand what happened at each step.
- Automatic Waiting: Cypress automatically waits for elements to load before interacting with them.
Frontend Performance Optimization
Optimizing your frontend application's performance is crucial for delivering a seamless user experience. Here are some key techniques:
- Minification and Compression
- Reduce File Size: Minify and compress HTML, CSS, and JavaScript files to reduce their size.
- Minification: Removes unnecessary characters like whitespace and comments.
- Compression: Reduces file size using algorithms like Gzip.
- Code Splitting
- Load Critical Resources First: Prioritize loading the most critical resources initially.
- Lazy Loading: Load non-critical resources only when needed.
- Dynamic Imports: Load code dynamically as it's required.
- Tree Shaking
- Remove Unused Code: Identify and remove unused code from your bundles.
- Smaller Bundle Sizes: Reduces the amount of code that needs to be downloaded and parsed.
- Lazy Loading
- Delay Loading of Resources: Defer the loading of non-critical resources like images, scripts, and stylesheets.
- Improve Initial Load Time: Faster initial page loads.
- Caching
- Browser Caching: Use HTTP headers to instruct browsers to cache static assets.
- Service Worker Caching: Cache static assets offline for faster loading.
- Content Delivery Network (CDN): Distribute your content across multiple servers to improve load times and reduce server load.
Frontend Security Best Practices
Security is a critical aspect of modern web development. Here are some essential best practices to protect your frontend applications:
- Input Validation
- Sanitize User Input: Filter and validate user input to prevent malicious code injection.
- Escape User Input: Properly escape user input to avoid XSS attacks.
- Validate Data Types: Ensure that user input matches the expected data type (e.g., numbers, strings, dates).
- Cross-Site Scripting (XSS) Prevention
- Input Sanitization: Escape special characters to render them as plain text.
- Output Encoding: Encode output to prevent malicious scripts from executing.
- Use a Security Library: Utilize libraries like DOMPurify to automatically sanitize HTML content.
- Cross-Site Request Forgery (CSRF) Protection
- CSRF Tokens: Include a unique, random token in forms and AJAX requests.
- Server-Side Validation: Verify the token on the server to prevent unauthorized requests.
- Secure HTTP
- Use HTTPS: Always use HTTPS to encrypt data transmission between the client and server.
- Enable HSTS: Force browsers to use HTTPS for all requests to your website.
- Keep Certificates Up-to-Date: Regularly renew and update your SSL/TLS certificates.
In conclusion, building a modern frontend tech stack requires a careful selection of tools and technologies. By understanding the core concepts of HTML, CSS, and JavaScript, and leveraging the power of frameworks like React, Angular, and Vue.js, you can create robust, scalable, and user-friendly web applications.
Remember to prioritize performance optimization, security best practices, and accessibility. Stay up-to-date with the latest trends and technologies to continuously improve your frontend development skills.