Building RESTful APIs with Django REST Framework
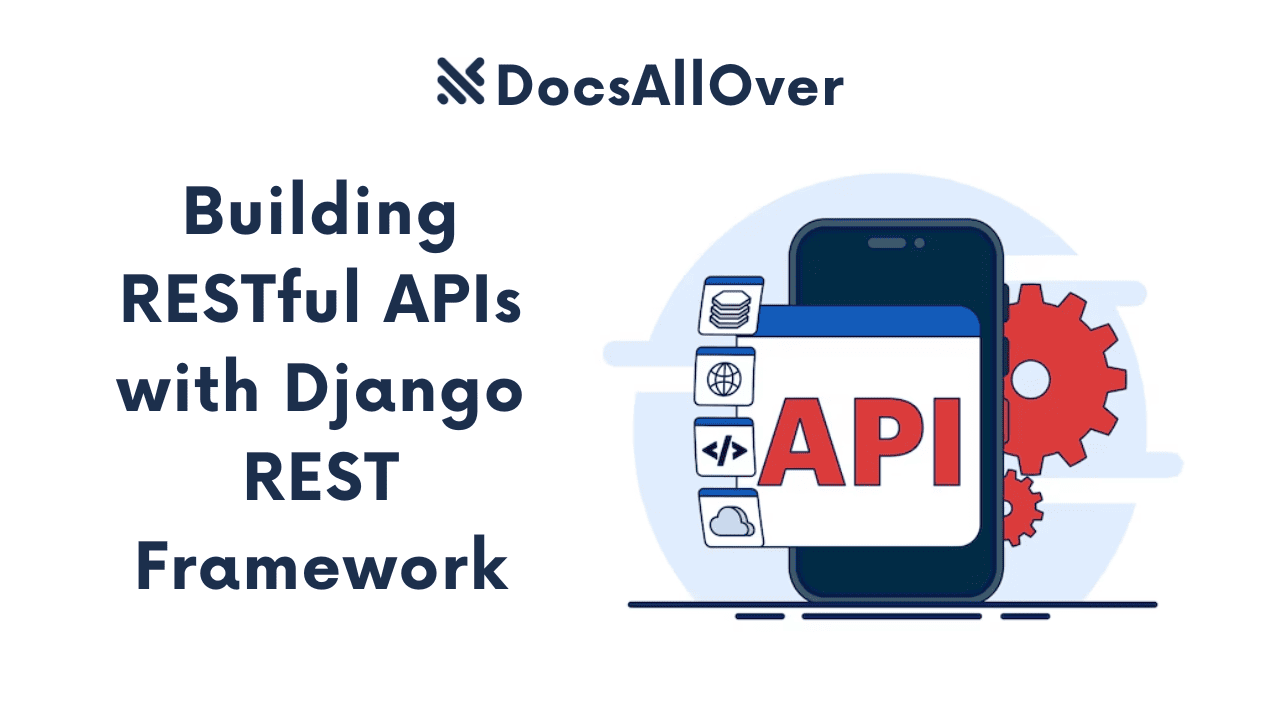
What is a RESTful API?
A RESTful API (Representational State Transfer API) is a software architectural style for designing networked applications. It's based on the HTTP protocol, using HTTP methods like GET, POST, PUT, DELETE, etc., to perform CRUD (Create, Read, Update, Delete) operations on resources.
Why use Django REST Framework (DRF)?
Django REST Framework (DRF) is a powerful and flexible toolkit for building Web APIs. It simplifies the process of building RESTful APIs by providing:
- Model Serializers: Easily convert Django models to JSON or other formats.
- ViewSets: Combine multiple view classes into a single class for efficient API design.
- Routers: Define URL patterns and map them to views.
- Authentication and Permissions: Implement robust authentication and permission systems.
- Pagination and Filtering: Control the number of results returned and filter data based on specific criteria.
Setting up a Django Project
Before diving into DRF, ensure you have Django installed. Create a new Django project and app:
Next, add rest_framework
to your requirements.txt
file and install it:
Remember to add rest_framework
to your INSTALLED_APPS
in settings.py.
In the next section, we'll delve into the core concepts of DRF, including Model Serializers, ViewSets, and Routers.
Core Concepts of DRF
Model Serializers
Model serializers are used to convert Django models into JSON or other formats, making them suitable for API responses.
ViewSets
ViewSets provide a convenient way to define a set of views for a particular resource. They combine common patterns like list
, retrieve
, create
, update
, and destroy
into a single class.
Routers
Routers are used to map URL patterns to views. DRF provides two types of routers:
- DefaultRouter: Automatically maps URL patterns for standard CRUD operations.
- SimpleRouter: Maps only a subset of CRUD operations.
Request and Response Handling
DRF provides tools for handling HTTP requests and responses:
- Request objects: Access request data, headers, and cookies.
- Response objects: Customize HTTP status codes, headers, and content.
- Parsers and Renderers: Handle different content types like JSON, XML, etc.
Authentication and Permissions
DRF offers built-in authentication and permission classes:
- Authentication:
- Session-based authentication
- Token-based authentication (JWT, TokenAuthentication)
- Basic authentication
- Permissions:
- AllowAny
- IsAuthenticated
- IsAdminUser
- Custom permissions
Building a Basic API
Creating a Simple Model
Let's create a simple Book model with fields for title
, author
, and publication_year
:
Defining a Serializer
The serializer will convert our Book
model instances into JSON format:
Creating a ViewSet
A ViewSet combines multiple view classes into a single class:
Configuring URL Patterns
Register the BookViewSet
with a router:
Testing the API
You can use tools like Postman or cURL to test your API. For example, to create a new book:
This will send a POST request to the books
endpoint with JSON data to create a new book.
Advanced Topics in DRF
API Versioning
To maintain backward compatibility and introduce new features, API versioning is crucial. DRF offers two primary techniques:
- URL-Based Versioning:
Append version numbers to URL paths:
/api/v1/books/
/api/v2/books/
- Header-Based Versioning:
Clients specify the desired API version in the Accept header:
Accept: application/json; version=2
Pagination
To handle large datasets efficiently, DRF provides pagination:
- Page-Based Pagination:
- Divides results into pages of a specific size.
- Use
PageNumberPagination
orLimitOffsetPagination
.
- Cursor-Based Pagination:
- Paginates results based on cursor positions.
- Use
CursorPagination
.
Filtering
DRF allows you to filter results based on query parameters:
- Filter Backends:
Use built-in filter backends like
DjangoFilterBackend
or create custom filter backends. - Search Filters:
Use
SearchFilter
to filter results based on specific fields.
Ordering
To sort results, use the OrderingFilter
:
Throttle Requests
To prevent abuse and rate limiting, use DRF's throttling features:
- Rate Limiting: Limits the number of requests per unit of time.
- Burst Rate Limiting: Allows a burst of requests within a short period.
You can configure throttling settings in your project's settings file.
Best Practices for Building RESTful APIs with DRF
API Design
- Adhere to RESTful Principles: Follow the core principles of REST, including resource-based URLs, HTTP methods, and statelessness.
- Consistent API Design: Maintain consistency in API design, including URL structure, request/response formats, and error handling.
- Clear and Concise Documentation: Provide comprehensive documentation that explains API endpoints, request parameters, response formats, and error codes.
Security
- Authentication: Implement robust authentication mechanisms like token-based authentication (JWT) or session-based authentication.
- Permissions: Control access to resources using permission classes and granular permissions.
- Data Validation: Validate input data to prevent security vulnerabilities and ensure data integrity.
- Secure Communication: Use HTTPS to encrypt communication between the client and the server.
Performance Optimization
- Caching: Implement caching strategies to reduce database queries and improve response times.
- Database Optimization: Optimize database queries and use appropriate indexing.
- Asynchronous Tasks: Use asynchronous tasks for long-running operations to improve responsiveness.
- Efficient Serialization: Choose the right serializer fields and optimize serialization processes.