Django Admin Interface: A Closer Look
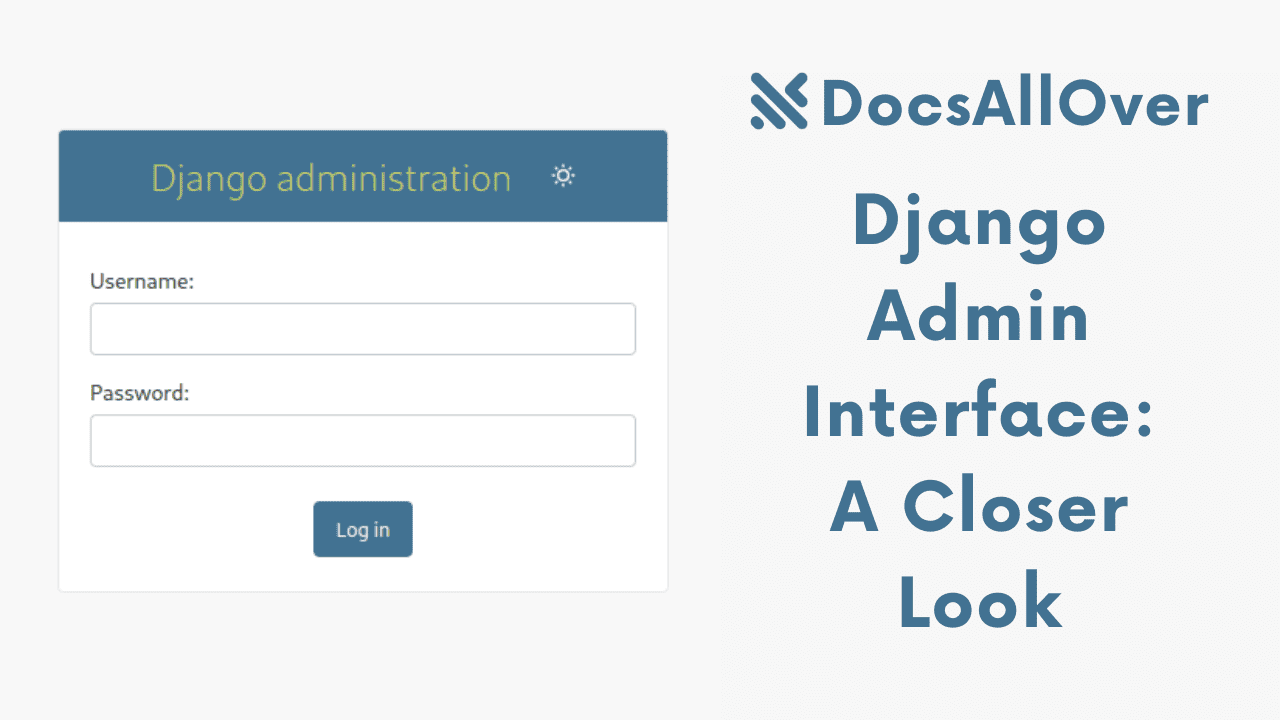
What is the Django Admin Interface?
The Django Admin Interface is a powerful web-based interface that allows you to manage and interact with your Django models. It's automatically generated by Django, saving you significant time and effort in building a custom admin interface.
Key Benefits of Using Django Admin
- Rapid development: Django Admin provides a ready-to-use interface for managing your data, accelerating development time.
- User-friendly interface: The intuitive interface makes it easy to add, edit, and delete data.
- Security: Django Admin comes with built-in security features to protect your data.
- Customizability: You can customize the admin interface to fit your specific needs.
Overview of the Basic Features
Model Administration:
- Automatic generation: Django automatically generates an admin interface for each model defined in your models.py file.
- CRUD operations: You can perform Create, Read, Update, and Delete (CRUD) operations on your models.
- Filtering and searching: Easily filter and search data within your models.
Example:
By defining this Book
model, Django Admin will automatically generate an interface to add, edit, and delete books.
Exploring the Django Admin Interface
Navigating the Admin Interface
Once you have a Django project set up and a superuser created, you can access the admin interface by going to the following URL in your browser:
http://127.0.0.1:8000/admin/
You will be prompted to log in with your superuser credentials.
Adding and Editing Models
- Adding a new record: Click the "Add" button at the top of the model's list page.
- Editing an existing record: Click the "Change" link next to the record you want to edit.
- Deleting a record: Click the "Delete" link next to the record you want to delete.
Filtering and Searching Data
- Filtering: Use the filter options at the top of the model's list page to narrow down the results.
- Searching: Use the search bar at the top of the model's list page to search for specific records.
Example:
If you have a Book
model with fields like title
, author
, and publication_date
, you can:
- Add a new book: Fill in the form fields for title, author, and publication date, and click "Save".
- Edit an existing book: Click the "Change" link for the book you want to edit, modify the fields, and click "Save".
- Filter books by author: Use the filter options to display only books by a specific author.
- Search for books by title: Use the search bar to find books that match a specific title.
By effectively navigating and using these features, you can efficiently manage your data within the Django admin interface.
Customizing the Django Admin Interface
Customizing Model Admin Classes
You can customize the admin interface for a specific model by creating a custom model admin class. This allows you to modify the display fields, add custom actions, and change the behavior of the admin interface for that model.
Example:
In this example, we've customized the admin interface for the Book
model to display specific fields, enable searching, and filtering by publication date.
Adding Custom Actions
You can define custom actions to perform specific tasks on selected objects within the admin interface.
Example:
This custom action allows you to mark selected books as published with a single click.
Registering Custom Models
If you have custom models that you want to manage in the admin interface, you need to register them.
Example:
Advanced Features of Django Admin
The Django Admin interface is a powerful tool for managing your Django models. It offers several advanced features to enhance your workflow:
Inlines
Inlines allow you to edit related models directly within the admin interface of a parent model. This simplifies data entry and management, especially for one-to-many and many-to-many relationships.
For example, if you have a Book
model with a ManyToManyField
to an Author
model, you can directly add or remove authors from a book within the book's admin form.
Model Hierarchies
Model hierarchies enable you to structure your data in a hierarchical manner. This is particularly useful for organizing complex data structures, such as categories and products.
By defining parent-child relationships between models, you can create intuitive admin interfaces that allow users to navigate and manage data effectively.
Exporting Data
While Django Admin doesn't have a built-in export function, you can use third-party libraries like django-import-export
to add export functionality. This allows you to export data in various formats, such as CSV, Excel, and JSON.
Filtering and Searching
Django Admin provides powerful filtering and searching options to help you find specific data. You can filter data by various fields, search for specific keywords, and sort data by different columns.
Additional Tips:
- Customizing the Admin Interface: You can customize the admin interface by creating custom admin classes, overriding default methods, and using template overrides.
- Using ModelAdmin Actions: You can define custom actions to perform specific tasks on selected objects, such as marking items as deleted or sending email notifications.
- Leveraging Django's Built-in Features: Take advantage of Django's built-in features like list filters, search fields, and ordering options to enhance the admin interface.
Security Considerations for Django Admin
The Django Admin interface is a powerful tool, but it's essential to implement robust security measures to protect your application.
User Authentication and Authorization
- Strong passwords: Encourage users to create strong, unique passwords.
- Two-factor authentication (2FA): Implement 2FA to add an extra layer of security.
- Regular password changes: Enforce regular password changes to minimize the risk of unauthorized access.
- Session timeouts: Set appropriate session timeout durations to automatically log out inactive users.
Protecting Against Common Attacks
- Cross-Site Request Forgery (CSRF): Django provides built-in CSRF protection. Ensure it's enabled and configured correctly.
- SQL Injection: Django's ORM helps prevent SQL injection attacks. However, be cautious when constructing raw SQL queries.
- Cross-Site Scripting (XSS): Django's template system and input validation can help mitigate XSS attacks.
- Clickjacking: Use appropriate HTTP headers to prevent clickjacking attacks.
- Regular security audits: Conduct regular security audits to identify and address vulnerabilities.
- Keep Django and dependencies up-to-date: Stay up-to-date with the latest security patches and updates.
Additional Tips:
- Limit access to the admin interface: Restrict access to authorized users only.
- Monitor access logs: Regularly review access logs to identify any suspicious activity.
- Use a web application firewall (WAF): A WAF can help protect your application from various web attacks.
- Implement rate limiting: Limit the number of requests to the admin interface to prevent brute-force attacks.
By following these security best practices, you can significantly enhance the security of your Django admin interface and protect your sensitive data.