Django Models and Database Interactions
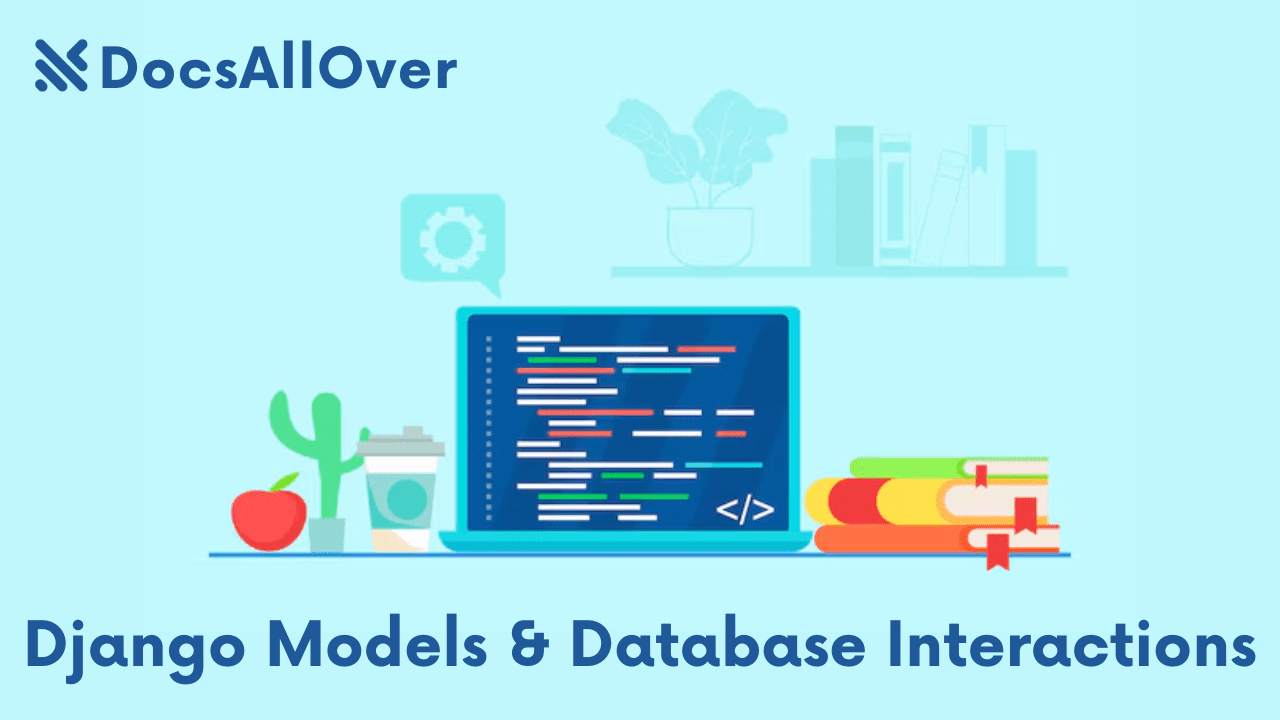
What are Django Models?
In the realm of Django, models serve as the architectural backbone for defining the structure of your application's data. They represent database tables and their corresponding fields. These models are Python classes that inherit from Django's Model
class.
The Role of Models in Django Applications
Django models play a pivotal role in:
- Database Schema Definition: They define the structure of your database tables, including columns, data types, and relationships.
- Object-Relational Mapping (ORM): They provide an abstraction layer over the database, allowing you to interact with data using Python objects instead of raw SQL.
- Data Validation: Ensure data integrity by defining validation rules for model fields.
- Data Relationships: Establish connections between different data models using relationships like
ForeignKey
andManyToManyField
.
Benefits of Using Django ORM
- Increased Productivity: The ORM simplifies database interactions, saving development time.
- Abstraction: Shields developers from database-specific SQL syntax.
- Data Integrity: Enforces data consistency through model definitions and validations.
- Cross-Database Compatibility: Django ORM supports multiple databases without significant code changes.
Basic Structure of a Django Model
A Django model is typically defined within a Python class that inherits from django.db.models.Model
. Each model field is represented by a field instance.
In this example, the Product
model defines three fields: name
, price
, and description
.
In the next section, we'll delve deeper into creating Django models.
Creating Django Models
Defining Model Fields
A Django model consists of fields that represent the columns in the corresponding database table. Each field has a specific data type and optional parameters.
In this example:
name
is a character field with a maximum length of 100 characters.price
is a decimal field with 10 digits total and 2 decimal places.description
is a text field that allows empty values.created_at
andupdated_at
are automatically set to the current date and time when a record is created or updated.
Common Field Types
Django provides a variety of field types to accommodate different data types:
- CharField: For text fields with a fixed maximum length.
- TextField: For long text without a fixed length.
- IntegerField: For integer values.
- DecimalField: For decimal numbers with precision.
- BooleanField: For boolean values (True or False).
- DateField: For dates.
- DateTimeField: For dates and times.
- EmailField: For email addresses.
- URLField: For URLs.
- ForeignKey: For one-to-one or many-to-one relationships.
- ManyToManyField: For many-to-many relationships.
Relationships Between Models
Django supports several types of relationships between models:
- ForeignKey: Represents a one-to-one or many-to-one relationship.
- ManyToManyField: Represents a many-to-many relationship.
In this example, Product
has a ForeignKey
to Category
, indicating that each product belongs to one category.
Meta Options for Model Customization
The Meta
class within a model allows you to customize various aspects of the model:
In this example, we've specified that products should be ordered by created_at
in descending order, and provided custom verbose names for the model.
In the next section, we'll explore database migrations in detail.
Model Fields in Detail
Core Field Types and Their Usage
Django offers a rich set of field types to accommodate various data requirements:
- CharField: Stores text with a maximum length.
- TextField: Stores long text without a maximum length.
- IntegerField: Stores integer values.
- DecimalField: Stores decimal numbers with precision.
- BooleanField: Stores boolean values (True or False).
- DateField: Stores dates.
- DateTimeField: Stores dates and times.
- EmailField: For storing email addresses.
- URLField: For storing URLs.
- FileField/ImageField: For uploading files/images.
Field Parameters and Options
Many field types accept additional parameters to customize their behavior:
- null: Indicates whether the field can be null.
- blank: Indicates whether the field can be blank (empty string).
- default: Specifies a default value for the field.
- choices: Defines a set of possible values for the field.
- unique: Ensures that the field value is unique across all instances.
- primary_key: Specifies the primary key for the model.
- auto_now: Sets the field to the current time on each save.
- auto_now_add: Sets the field to the current time only on the first save.
Custom Field Types and Validators
While Django provides a rich set of built-in field types, you can create custom fields for specific requirements. You can also define custom validators to enforce complex validation logic.
In the next section, we'll delve into database migrations.
Database Migrations
Understanding Migrations
Database migrations are a systematic approach to managing changes in your database schema over time. Django's migration system automates this process, ensuring that your database structure stays in sync with your models.
Creating Migrations
When you make changes to your models, you can use the makemigrations
command to generate a migration file:
This command creates a migration file in the migrations
directory of your app. The file contains instructions for modifying the database schema based on the changes you made to your models.
Applying Migrations
To apply the changes to your database, use the migrate
command:
This command executes the pending migrations, creating or altering tables as needed.
Managing Database Schema Changes
- Adding fields: Add new fields to a model and create a migration.
- Removing fields: Remove fields from a model and create a migration.
- Altering fields: Modify field types or other attributes and create a migration.
- Creating models: Create new models and generate corresponding migrations.
- Deleting models: Be cautious when deleting models, as it might affect related data.
Django's migration system helps you manage these changes efficiently and safely.
In the next section, we'll explore model methods in detail.
Model Methods
Creating Custom Methods for Models
You can define custom methods within your models to encapsulate logic related to the model's data. These methods provide a clean interface for interacting with model instances.
Managers and Custom Querysets
Django provides a Manager
class for handling database queries. You can create custom managers to provide additional query methods or to filter querysets.
Now you can use Product.objects.active_products()
to get active products.
Model Instances and Querysets
- Model Instances: Represent individual records in the database. They are created by saving a model object.
- Querysets: Represent a collection of model instances. They are lazy, meaning the database query is executed only when necessary.
By understanding model methods, managers, and querysets, you can effectively interact with your database and retrieve the data you need.
In the next section, we'll explore database queries in detail.
Database Queries
Querying Data Using Django ORM
The Django ORM provides a powerful and intuitive interface for interacting with your database.
Filtering, Ordering, and Limiting Querysets
- Filtering: Use the
filter()
method to retrieve specific records based on conditions. - Ordering: Use the
order_by()
method to sort querysets. - Limiting: Use the
limit()
method to restrict the number of results.
Related Objects and Prefetching
When working with related models, use the following:
- Accessing related objects: Use the related field name to access related objects.
- Prefetching: Optimize query performance by prefetching related objects.
Related Objects and Prefetching
When working with related models, use the following:
- Accessing related objects: Use the related field name to access related objects.
- Prefetching: Optimize query performance by prefetching related objects.
Raw SQL Queries (When Necessary)
While the Django ORM is powerful, there might be cases where raw SQL is required for complex queries or database-specific features.
Note: Use raw SQL judiciously as it can reduce the benefits of the ORM and introduce potential security vulnerabilities.
In the next section, we'll explore CRUD operations.
CRUD Operations
Creating, Retrieving, Updating, and Deleting Objects
The acronym CRUD encapsulates the fundamental operations performed on data in a database:
- Create: Inserting new data into the database.
- Retrieve: Fetching existing data from the database.
- Update: Modifying existing data in the database.
- Delete: Removing data from the database.
In Django, these operations are straightforward using model instances and querysets.
Model Forms for Data Input
Django provides a convenient way to create forms based on your models using the ModelForm
class. This simplifies data input and validation.
Handling Database Transactions
For complex operations involving multiple database changes, you can use database transactions to ensure data integrity.
In the next section, we'll explore optimization and performance considerations for Django models.
Optimization and Performance
Query Optimization Techniques
Efficient database queries are essential for application performance. Here are some techniques:- Select only required fields: Use
only()
ordefer()
to include or exclude specific fields. - Prefetching related objects: Use
prefetch_related()
to optimize related object fetching. - Selecting related objects: Use
select_related()
for one-to-one and many-to-one relationships. - Query chaining: Combine multiple query methods for complex filtering and ordering.
- Raw SQL (with caution): Use raw SQL for complex queries that cannot be efficiently expressed using the ORM.
Using Database Indexes Effectively
Indexes can significantly improve query performance. Create indexes on frequently searched fields:
The db_index=True
argument creates an index on the name
field.
Caching Strategies for Database Queries
Caching can reduce database load and improve performance:
- Django cache framework: Use the built-in cache framework to store query results.
- Third-party caching solutions: Consider using dedicated caching systems like Redis or Memcached.
- Cache invalidation: Implement strategies to invalidate cache entries when data changes.
By applying these optimization techniques, you can significantly enhance the performance of your Django application.
In the next section, we'll explore advanced topics related to Django models.
Advanced Topics
Model Inheritance
Django supports model inheritance, allowing you to create base models with shared attributes and then inherit from them to create more specific models.
- Abstract base classes: Define common fields and behaviors for multiple models without creating a concrete table.
- Multi-table inheritance: Create subclasses that inherit fields from a parent model and have their own database table.
- Proxy models: Create a new model that represents the same database table as another model, often used for customization.
Generic Relations
Generic relations allow you to create relationships between models without defining specific foreign keys in advance. This is useful for flexible data modeling.
Proxy Models
Proxy models provide a way to customize model behavior without altering the original model's definition. They are often used for administrative purposes or to modify model querysets.
Database-Specific Features
While Django ORM provides a high-level abstraction, you might need to interact with database-specific features for certain optimizations or complex queries.
- Raw SQL: Use raw SQL queries when necessary, but exercise caution due to potential security risks and reduced ORM benefits.
- Database functions: Leverage database-specific functions for performance or specialized operations.
By understanding these advanced topics, you can effectively model complex data structures and optimize database interactions in your Django applications.