Django Templates and Templating Engine
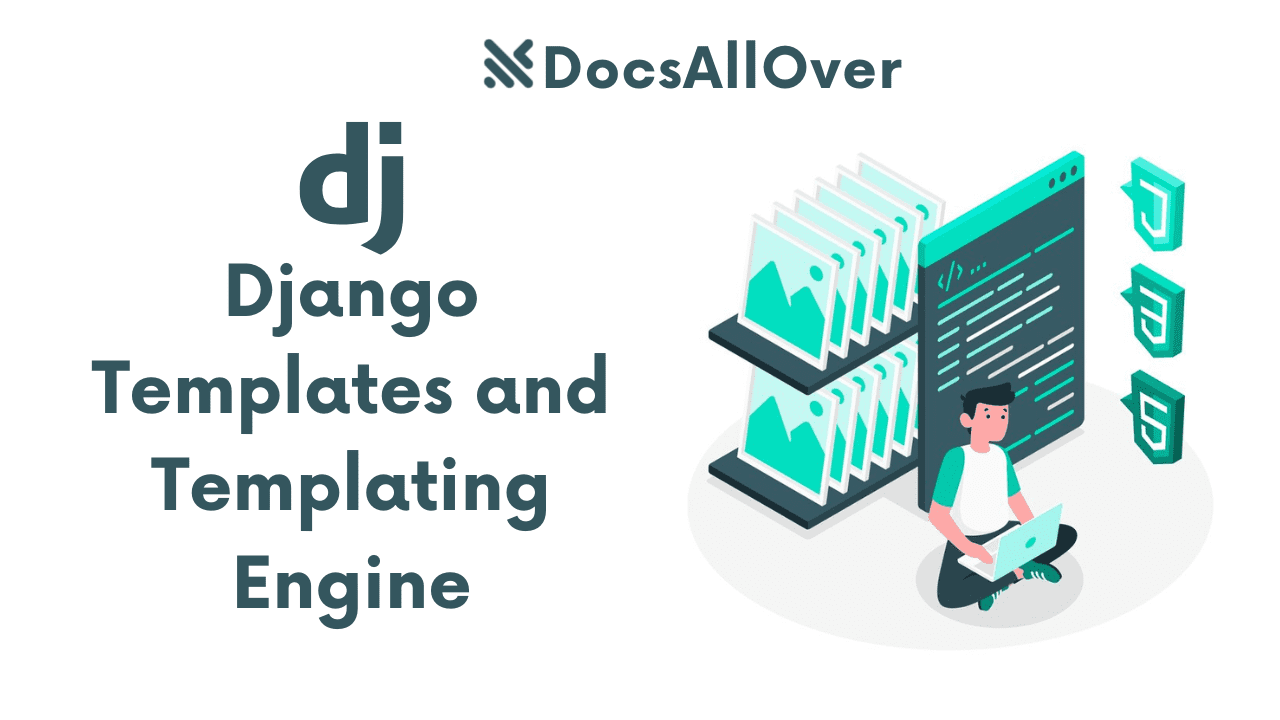
What are Templates in Django?
Templates in Django are HTML files that contain placeholders for dynamic content. They allow you to separate the presentation logic from the application logic, making your code more maintainable and reusable.
Role of the Templating Engine in Django
The Django templating engine is responsible for rendering templates and replacing placeholders with actual data from your Python code. It provides a powerful and flexible way to create dynamic web pages.
Benefits of Using Django Templates
- Separation of concerns: Templates separate the presentation logic from the application logic, making your code more organized and easier to maintain.
- Reusability: Templates can be reused across multiple views, reducing code duplication.
- Dynamic content: Templates allow you to easily generate dynamic content based on data from your Python code.
- Templating language: Django's templating language provides a simple and intuitive syntax for creating templates.
Template Syntax
Basic Template Syntax
Django templates use a simple syntax that is similar to HTML. The most common elements are:
- Variables: Placeholders for dynamic content.
- Tags: Special instructions for the templating engine.
- Filters: Functions that modify the output of variables.
- Comments: Non-rendered text for documentation.
Variables
Variables are enclosed in double curly braces {{ }}
.
Example:
Tags
Tags are enclosed in double curly braces with a %
sign at the beginning and end.
Example:
Filters
Filters are used to modify the output of variables.
Example:
This will display "N/A" if my_variable
is None
.
Comments
Comments are ignored by the templating engine. They are enclosed in curly braces with {#
and #}
.
Example:
By understanding these basic elements, you can start creating dynamic templates in Django.
Template Inheritance
Base Templates and Child Templates
Template inheritance allows you to create a base template that defines the overall structure of your pages, and then extend this base template with child templates that provide specific content.
Base template:
Child template:
In this example, the child template extends the base.html
template and overrides the title
and content
blocks.
Overriding and Extending Templates
- Overriding: Replacing the content of a block in a child template.
- Extending: Adding content to a block in a child template.
Example:
Using Template Inheritance Effectively
- Create a clear base template: Define the overall structure of your pages in the base template.
- Use blocks for reusable content: Place reusable content in blocks that can be overridden or extended in child templates.
- Avoid excessive nesting: Keep your template hierarchy simple to improve readability and maintainability.
Common Template Tags
{% if %}
, {% else %}
, and {% endif %}
These tags are used for conditional logic.
Example:
{% for %}
, {% endfor %}
These tags are used to iterate over sequences.
Example:
{% url %}
This tag is used to generate URLs for views.
Example:
{% include %}
This tag is used to include other templates within a template.
Example:
{% block %}
and {% endblock %}
These tags are used for template inheritance.
Example:
By understanding and using these common template tags, you can create dynamic and flexible templates in Django.
Custom Template Tags and Filters
Creating Custom Template Tags
Custom template tags allow you to create reusable logic that can be used in your templates.
Example:
This custom tag calculates the total price of a list of items.
Creating Custom Template Filters
Custom template filters allow you to modify the output of variables.
Example:
This custom filter censors profanity and obscenity in the given text.
Using Custom Tags and Filters in Templates
To use a custom tag or filter in a template, you must load the corresponding app in your settings.py
file. Then, you can use the custom tag or filter in your template.
Example:
By creating custom tags and filters, you can extend the functionality of Django's templating system and tailor it to your specific needs.
Best Practices for Django Templates
Keep Templates Clean and Readable
- Use consistent indentation: Maintain consistent indentation to improve readability.
- Avoid excessive nesting: Minimize the nesting of tags and blocks to keep templates easier to understand.
- Use meaningful variable names: Choose descriptive names for variables and context variables.
Use Template Inheritance Effectively
- Create a base template: Define the overall structure of your pages in a base template.
- Extend the base template: Use child templates to override or extend specific sections of the base template.
- Avoid excessive inheritance: Limit the depth of inheritance to keep your templates manageable.
Avoid Complex Logic in Templates
- Move logic to views: Handle complex logic in your Python views rather than in templates.
- Use custom template tags and filters: Create custom tags and filters to encapsulate complex logic.
- Keep templates focused on presentation: Templates should primarily be concerned with presenting data, not performing complex calculations or operations.
Test Templates Thoroughly
- Test with different data: Ensure that your templates work correctly with various data inputs.
- Check for errors: Look for any syntax errors or unexpected behavior in your templates.
- Use a template debugging tool: Consider using a template debugging tool to help identify and fix issues.
By following these best practices, you can write clean, maintainable, and efficient Django templates.