Django Unleashed: A Comprehensive Guide to Powerful and Scalable Python

Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Built by experienced developers, it takes care of much of the hassle of web development, so you can concentrate on writing your app without needing to reinvent the wheel.
If you're looking for a comprehensive guide to Django, look no further. This blog post will cover everything you need to know, from the basics of setting up a Django project to more advanced topics like deployment and scalability.
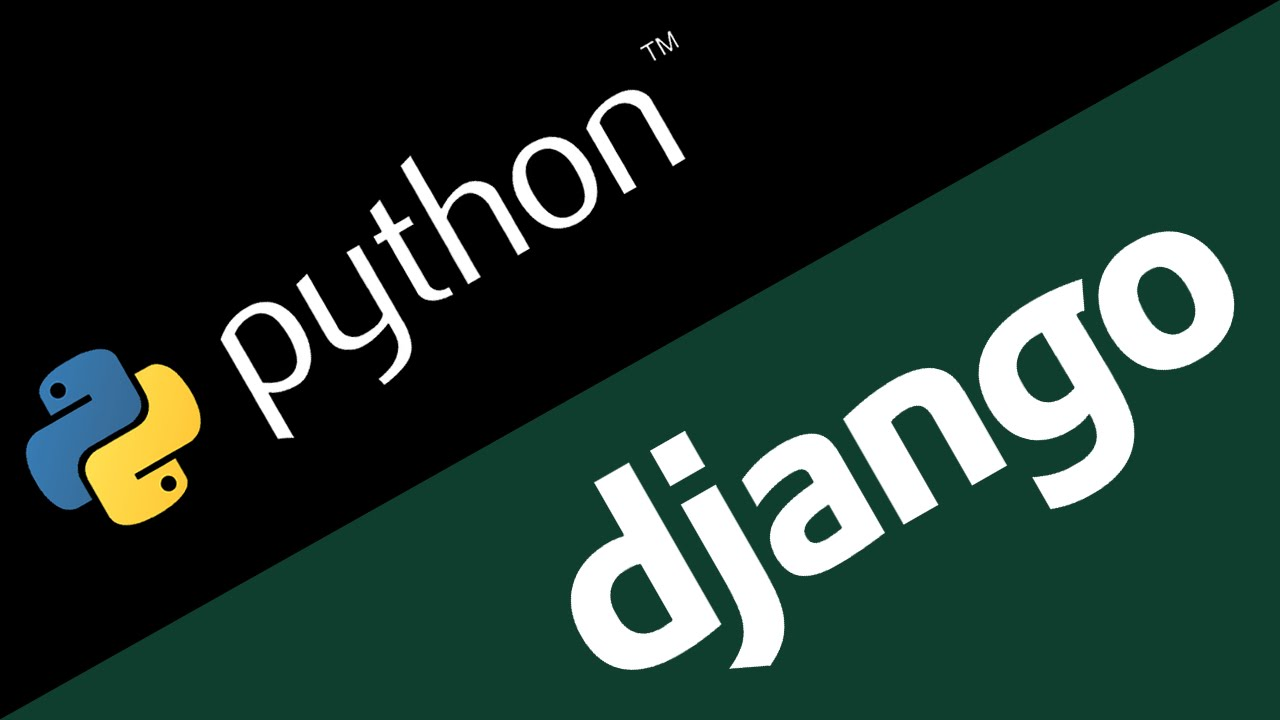
Django's core features
- Object-Relational Mapping (ORM): Django's ORM enables seamless communication between Python objects and the underlying database, abstracting away complex SQL queries. Developers can define database models using Python classes, making it easy to work with data and perform database operations.
- URL Routing and View Handling: Django's URL routing system maps URLs to specific views, allowing developers to define how different parts of their application respond to incoming requests. Views are Python functions or classes that process requests, retrieve data from the database, and render HTML templates to generate the response.
- Template Engine: Django's template engine provides a powerful way to generate dynamic HTML content. Templates are written using HTML markup with embedded Python code, allowing for the dynamic rendering of data from views.
- Forms and Validation: Django simplifies the creation and handling of HTML forms. It provides a form API that automatically generates HTML forms from Python code, handles form submissions, and performs validation on user input.
- Authentication and Authorization: Django includes robust authentication and authorization systems, making it easy to add user registration, login, and access control to web applications.
- Admin Interface: Django's admin interface is a built-in feature that automatically generates a user-friendly administration panel for managing site content, models, and data. It provides an intuitive interface for performing CRUD (Create, Read, Update, Delete) operations on database records.
Django is a popular choice for developing web applications of all sizes, from simple blogs to complex e-commerce sites. It is known for its ease of use, scalability, and security.
18 Benefits of Django
Django is a popular choice for web development for a number of reasons. Here are 20 of the benefits of using Django:
1. Python
Django is written in Python, which is a popular and versatile programming language. Python is known for its simplicity, readability, and wide range of libraries and frameworks. Using Python makes Django easier to learn and use, and it gives developers access to a vast ecosystem of tools and resources.
2. Batteries included
Django is a batteries-included framework, which means that it comes with a wide range of built-in features, such as:
- Object-relational mapper (ORM): Django's ORM makes it easy to interact with databases. The ORM provides a Pythonic interface for creating, reading, updating, and deleting data in the database.
- Template system: Django's template system makes it easy to generate HTML pages. The template system uses a simple syntax that is easy to learn and use.
- User authentication system: Django's user authentication system makes it easy to implement user authentication and authorization in your web applications.
- Session management system: Django's session management system makes it easy to track user sessions and store session data.
- Caching system: Django's caching system makes it easy to cache frequently accessed data, which can improve the performance of your web applications.
- Administration interface: Django's administration interface provides a web-based interface for managing your Django applications.
- Development server: Django's development server makes it easy to develop and test Django applications locally.
These features give developers a head start on building web applications, and they can be used to implement many common features without having to write any custom code.
3. Security
Django is designed with security in mind. It includes a number of features that help to protect web applications from common attacks, such as cross-site scripting (XSS), SQL injection, and clickjacking. Django also provides a number of tools for testing the security of web applications.
4. Scalability
Django is a scalable framework that can handle large amounts of traffic. It is used by a number of high-profile websites, such as Instagram, Pinterest, and Mozilla. Django's scalability is due in part to its use of asynchronous programming and caching.
5. Versatility
Django can be used to build a wide range of web applications, from simple websites to complex social networks. It is also well-suited for building e-commerce websites, content management systems, and APIs.
6. Large community
Django has a large and active community of users and developers. This means that there is a wealth of resources available to Django users, including documentation, tutorials, and support forums.
7. Easy to learn
Django is relatively easy to learn, especially for developers who are already familiar with Python. Django's documentation is clear and comprehensive, and there are a number of tutorials and online courses available.
8. Fast development
Django's built-in features and batteries-included approach can help developers to build web applications more quickly. Django also provides a number of tools for automating tasks, such as scaffolding and code generation.
9. Clean and maintainable code
Django encourages developers to write clean and maintainable code. Django's code conventions are well-established and easy to follow. Django also provides a number of tools for testing and debugging code.
10. Reusable components
Django applications are made up of reusable components, such as models, views, and templates. This makes it easy to reuse code and to build new applications based on existing code.
11. Third-party packages
There is a large ecosystem of third-party packages available for Django. These packages can be used to add new features and functionality to Django applications.
12. Administration interface
Django comes with a built-in administration interface that makes it easy to manage Django applications. The administration interface allows users to create, edit, and delete data, as well as to manage users and permissions.
13. Development server
Django comes with a built-in development server that makes it easy to develop and test Django applications. The development server can be used to run Django applications locally without having to deploy them to a production server.
14. Internationalization and localization support
Django provides built-in support for internationalization and localization. This makes it easy to build Django applications that can be used by users all over the world.
15. Security updates
Django is a well-maintained framework and the Django team regularly releases security updates. This helps to keep Django applications safe from known security vulnerabilities.
16. Commercial support
There are a number of companies that offer commercial support for Django. This can be useful for organizations that need help with Django development or deployment.
17. Open source
Django is an open source framework. This means that it is free to use and modify. Django's source code is available on GitHub and anyone can contribute to its development.
18. Large and active community
Django has a large and active community of users and developers. This means that there is a wealth of resources available to Django users, including documentation, tutorials, and support forums.
Getting started with Django
Installing Python and Django
To get started with Django, you'll need to install Python 3 and the Django framework. You can do this using the Python Package Index (PyPI):
Creating a Django project
Once you have Django installed, you can create a new Django project using the django-admin command:
This will create a new directory called myproject containing all of the necessary files for your Django project.
Creating a Django app
Next, you'll need to create a Django app. This is where you'll put all of your code for your web application:
This will create a new directory called myapp containing all of the necessary files for your Django app.
Running the Django development server
To run the Django development server, navigate to the mysite directory and run the following command:
This will start the Django development server on port 8000. You can now access your Django project at http://localhost:8000
in your web browser.
The runserver command
The runserver command is one of the most important commands in Django. It is used to start the Django development server, which allows you to test your Django applications locally.
The runserver command takes a number of options, such as the port number and the address to bind to. For example, to start the Django development server on port 8080
, you would use the following command:
To start the Django development server on a different address, you would use the --address
option. For example, to start the Django development server on the address 192.168.1.100
, you would use the following command:
You can also use the runserver command to start the Django development server in debug mode. This will allow you to see detailed error messages if your Django application encounters an error. To start the Django development server in debug mode, use the --debug
option. For example, to start the Django development server in debug mode on port 8080, you would use the following command:
The runserver command is a powerful tool that can be used to develop, test, and debug Django applications.
Now you're ready to start writing some code!
1. Defining a Model:
In Django, models are used to define the structure of the database tables. For example, to create a model for a blog post, you can define a class in Django's models.py file:
2. Creating Views:
Views are responsible for handling requests and returning responses. Here's an example of a view that fetches all blog posts from the database and renders them using a template:
3. URL Routing:
URL routing in Django helps map URLs to views. Here's an example of defining URL patterns in the urls.py file:
4. Template Rendering:
Django's template engine allows for the separation of HTML/CSS markup from the application logic. Here's an example of rendering a blog post using a template:
5. User Authentication:
Django provides built-in views and functionality for user authentication. Here's an example of a login view that authenticates users:
6.Deployment and scalability
Once you've built your Django application, you need to deploy it to a production server. There are many different ways to do this, but one popular option is to use a cloud hosting service like Heroku or AWS Elastic Beanstalk.
Once your application is deployed, you need to make sure it can scale to handle a large number of users.
These examples provide a glimpse into the coding aspects of Django. By exploring the Django documentation and tutorials, you can dive deeper into the framework's capabilities and learn how to build more complex web applications with Django.
Example: Building a Simple Blogging Platform
To illustrate Django's capabilities, let's consider an example of building a simple blogging platform. We can define models for blog posts, categories, and comments. With Django's ORM, we can easily create, read, update, and delete blog posts, associate categories with posts, and allow users to leave comments. By utilizing Django's built-in admin interface, we can efficiently manage and publish blog content.
Django is a versatile and feature-rich web framework that empowers developers to create powerful web applications using Python. Its simplicity, scalability, and extensive ecosystem make it an excellent choice for building projects of any size and complexity. By leveraging Django's key features, such as its ORM, admin interface, URL routing, and template engine, developers can streamline their web development process and deliver high-quality applications efficiently.