Control Flow Statements in C#: Conditional Logic and Loops
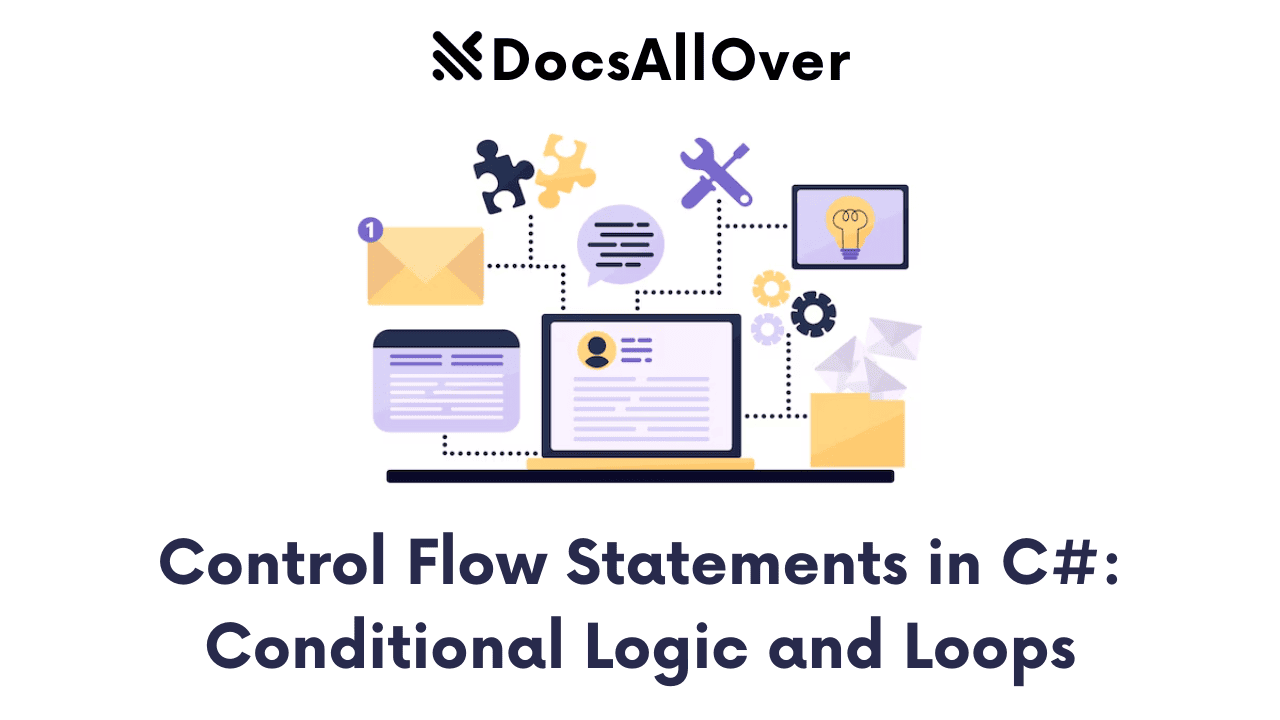
What are Control Flow Statements?
Control flow statements are programming constructs that determine the order in which statements are executed within a program. They allow you to make decisions, repeat actions, and control the flow of execution based on specific conditions.
Importance of Control Flow in Programming
Control flow statements are essential for building dynamic and flexible programs. They enable you to:
- Make decisions: Choose different code paths based on conditions.
- Repeat actions: Execute code multiple times.
- Control the flow of execution: Determine the order in which statements are executed.
Overview of Conditional Logic and Loops in C#
- Conditional logic: Statements that allow you to execute different code blocks based on conditions.
- Loops: Statements that allow you to repeat a block of code multiple times.
In the following sections, we'll explore the different types of control flow statements in C# and their applications.
Conditional Logic
if
Statement
The if
statement is used to execute a block of code if a specified condition is true.
Syntax & Example:
if-else
Statement
The if-else
statement allows you to execute one block of code if a condition is true, and another block if the condition is false.
Syntax & Example:
if-else-if
Ladder
The if-else-if
ladder allows you to check multiple conditions and execute a different block of code for each condition.
Syntax & Example:
switch
Statement
The switch
statement is used to select one of several code blocks to be executed based on the value of an expression.
Syntax & Example:
By understanding these conditional statements, you can effectively control the flow of execution in your C# programs.
Loops
for
Loop
The for
loop is used to iterate over a sequence of values or perform a task a specified number of times.
Syntax & Example:
while
Loop
The while
loop is used to repeat a block of code as long as a specified condition is true.
Syntax & Example:
do-while
Loop
The do-while
loop is similar to the while loop, but it guarantees that the code block is executed at least once, even if the condition is false from the beginning.
Syntax & Example:
Nested Loops
You can nest loops within other loops to create more complex control flow patterns.
Example:
By understanding these loop structures, you can effectively repeat code blocks and perform iterative tasks in your C# programs.
Best Practices for Control Flow
Indentation and Formatting
- Consistent indentation: Use consistent indentation to improve code readability.
- Meaningful variable names: Choose descriptive names for variables and conditions.
- Avoid unnecessary nesting: Try to keep nested loops and conditions to a minimum.
Code Readability and Maintainability
- Comments: Use comments to explain complex logic or the purpose of code blocks.
- Break down complex expressions: If expressions become too long or complex, consider breaking them down into smaller, more readable parts.
- Choose appropriate control flow structures: Select the most suitable control flow statement for the task at hand.
Avoiding Common Pitfalls
- Infinite loops: Ensure that loop conditions eventually become false to avoid infinite loops.
- Off-by-one errors: Be careful when using loop counters and conditions to avoid errors in indexing or counting.
- Nested conditions: Avoid excessive nesting of if statements, as it can make code difficult to read and understand.
By following these best practices, you can write more readable, maintainable, and error-free C# code.
Real-World Examples of Control Flow Statements
Menu-Driven Programs
Validating User Input
Iterating Over Collections
Implementing Game Logic
Creating a Simple Calculator
These examples demonstrate how control flow statements can be used in various real-world scenarios to create dynamic and interactive programs.