Delegates and Events in C#: Enabling Asynchronous Programming
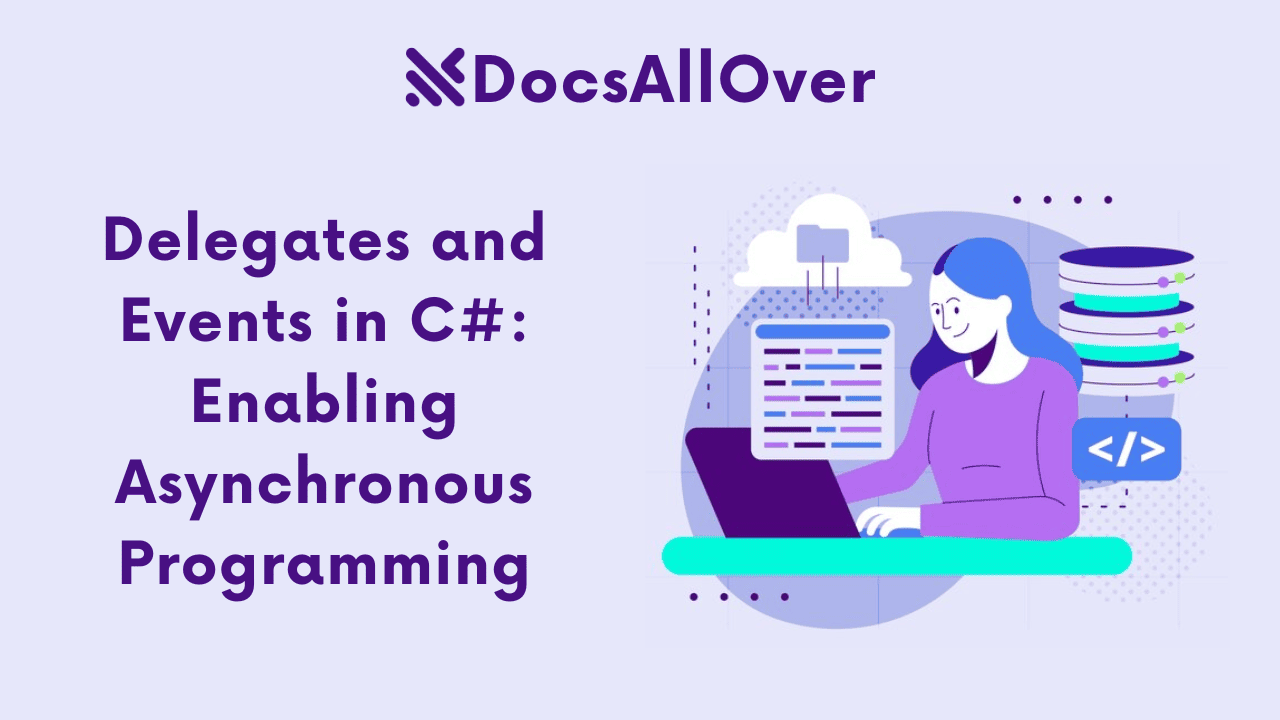
What are Delegates in C#?
- Definition: In C#, a delegate is a type that represents a reference to a method. Think of it as a function pointer, a placeholder for a method that will be executed later.
- Defining a Delegate Type: You define a delegate type using the
delegate
keyword, specifying the return type and parameters of the methods it can hold. - Using Delegates as Function Pointers: You can assign any method that matches the delegate's signature to a delegate instance.
What are Events in C#?
- Publishing and Subscribing to Events: An event is a notification mechanism. Objects can "publish" events, and other objects can "subscribe" to those events. When the event is "raised" (triggered), all subscribed objects are notified.
- Event Handlers: Event handlers are methods that are executed when an event is raised.
The Importance of Asynchronous Programming
- Modern applications often need to perform tasks concurrently to improve responsiveness and efficiency.
- Asynchronous programming allows you to execute tasks in the background without blocking the main thread of execution.
- Delegates and events play a crucial role in enabling asynchronous programming in C#.
Delegates in C#
Defining Delegate Types
- Syntax:
<return_type>
: The data type of the value returned by the methods that this delegate can hold.<delegate_name>
: The name of the delegate.<parameter_list>
: A comma-separated list of parameters for the methods that this delegate can hold, including their data types.- Example:
Using Delegates to Refer to Methods
- Assigning Methods to Delegate Instances: You can assign any method that matches the delegate's signature to a delegate instance.
- Invoking Methods Through Delegates: You can invoke the method referenced by a delegate instance by calling the delegate.
Practical Examples
- Using Delegates with Mathematical Operations:
- Using Delegates with Sorting Algorithms:
In the next section, we'll explore the concept of events in C#.
Events in C#
Publishing Events
- Declaring Events: In C#, you declare an event using the
event
keyword. Events are typically declared within a class. - Raising Events: To raise an event, you use the
+=
operator to invoke the event.
Subscribing to Events
- Creating Event Handlers: Event handlers are methods that respond to events. They must match the delegate signature specified in the event declaration.
- Subscribing to Events: To subscribe to an event, use the
+=
operator to assign the event handler method to the event. - Unsubscribing from Events: To stop receiving notifications for an event, use the
-=
operator to remove the event handler.
Example: Creating a Custom Event for Button Clicks
In this example, the Button
class publishes a Clicked
event. The Form
class subscribes to this event and handles the event by printing a message to the console.
In the next section, we'll explore how to use delegates and events for asynchronous programming.
Asynchronous Programming with Delegates and Events
Benefits of Asynchronous Programming
- Improved Responsiveness: Asynchronous operations allow your application to remain responsive while performing time-consuming tasks (e.g., network requests, file I/O).
- Increased Throughput: By executing tasks concurrently, you can improve the overall performance and throughput of your application.
- Better User Experience: Asynchronous operations prevent the user interface from freezing while long-running tasks are being performed.
Using Delegates to Invoke Methods Asynchronously
- You can use delegates to invoke methods asynchronously using techniques like:
- Threading: Create a new thread to execute the method.
- Task Parallel Library (TPL): Utilize the TPL to easily execute tasks asynchronously.
Using Events to Notify Other Components of Asynchronous Operations
You can use events to notify other components when an asynchronous operation starts, progresses, or completes.
Example: Implementing an Asynchronous Download Operation
In this example, the Downloader
class raises a DownloadProgressChanged
event periodically during the download operation. The DownloadProgressForm
subscribes to this event and updates the progress bar in the user interface.
Best Practices and Considerations
- Thread Safety and Synchronization
- When dealing with multithreaded applications, it's crucial to ensure thread safety.
- If multiple threads access and modify shared data concurrently, it can lead to unexpected behavior (race conditions) or data corruption.
- Use synchronization mechanisms like locks (mutexes) or semaphores to control access to shared resources and prevent race conditions.
- Avoiding Deadlocks
- A deadlock occurs when two or more threads are blocked indefinitely, waiting for resources held by each other.
- To avoid deadlocks:
- Acquire locks in a consistent order.
- Use timeouts to avoid indefinite waiting.
- Design your application carefully to minimize the risk of deadlocks.
- Using the
async
andawait
Keywords for Asynchronous Operations- The
async
andawait
keywords provide a more elegant and easier-to-read way to write asynchronous code in C#. - The
async
keyword marks a method as asynchronous. - The
await
keyword pauses the execution of theasync
method until the awaited task completes.
- The
In this example, the DownloadDataAsync
method is marked as async
. The await
keyword pauses the method's execution until the client.GetStringAsync()
task completes.
Key Takeaways:
- Delegates and events are powerful mechanisms for building event-driven and asynchronous applications in C#.
- Careful consideration of thread safety and synchronization is essential when working with concurrent operations.
- The async and await keywords provide a more modern and easier-to-use approach to asynchronous programming in C#.
Further Exploration:
- Explore advanced event patterns like the Observer pattern and the Publish-Subscribe pattern.
- Learn more about the Task Parallel Library (TPL) and its features.
- Investigate techniques for handling asynchronous exceptions.
By mastering the concepts of delegates and events, you can build more responsive, efficient, and robust applications in C#.