Functions in Python: Your toolkit for reusable code
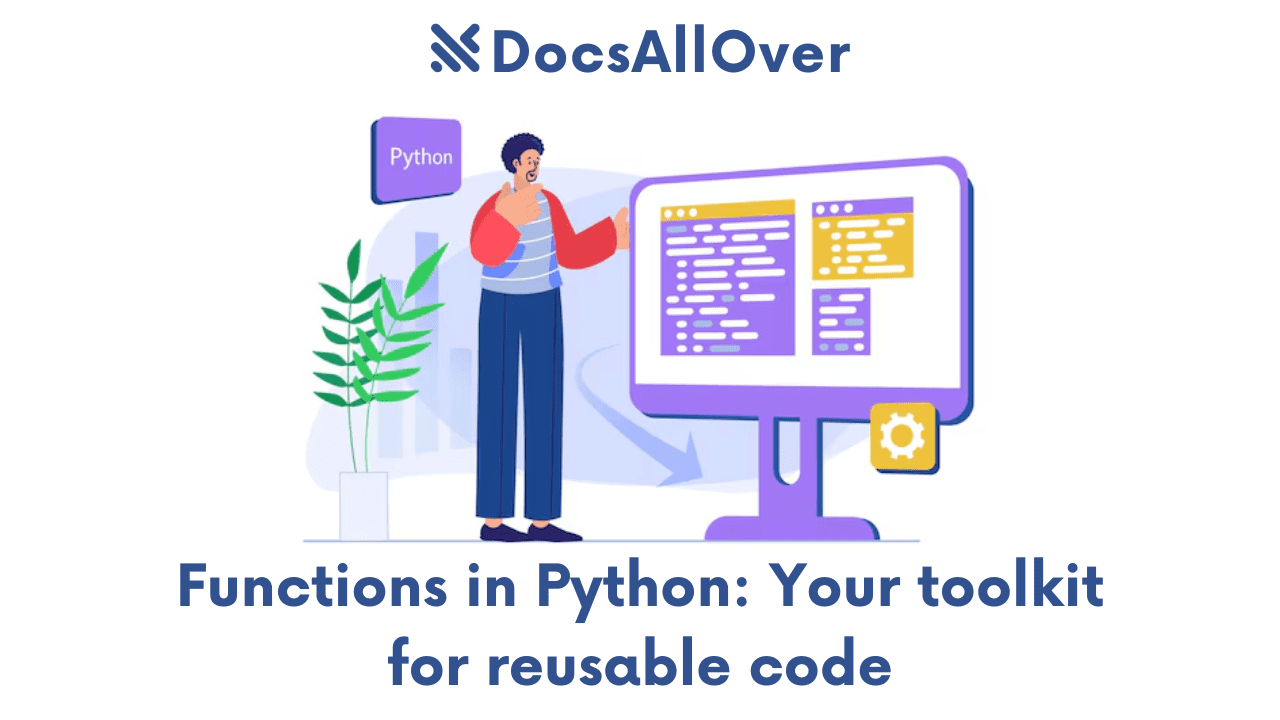
What are Functions in Python?
Functions are blocks of reusable code that perform a specific task. They allow you to break down your code into smaller, more manageable units, making it easier to organize, understand, and maintain.
Importance of Functions in Programming
Functions are essential for writing well-structured and efficient Python code. They offer several key benefits:
- Modularity: Functions break down complex problems into smaller, more manageable parts.
- Reusability: Functions can be called multiple times throughout your code, avoiding code duplication.
- Readability: Functions improve code readability by making it easier to understand the purpose of different sections.
- Maintainability: Functions make it easier to modify and update code without affecting other parts of the program.
- Testing: Functions can be tested independently, making it easier to ensure code correctness.
Benefits of Using Functions
- Code organization: Functions help structure your code and improve readability.
- Efficiency: Reusable functions can avoid redundant code execution.
- Maintainability: Functions make it easier to modify and update code without affecting other parts of the program.
- Collaboration: Functions can be shared and reused among team members.
Defining Functions
Function Syntax
In Python, functions are defined using the def
keyword followed by the function name, parentheses for parameters, and a colon. The function body is indented below the colon.
Example:
Parameters and Arguments
- Parameters: Variables defined within the parentheses of a function definition.
- Arguments: Values passed to a function when it's called.
Example:
Return Values
Functions can optionally return values using the return
statement.
Example:
Docstrings
Docstrings are optional strings that provide documentation for functions. They are placed immediately after the function definition.
Example:
Function Scope
Global and Local Variables
- Global variables: Variables defined outside of any function. They can be accessed from anywhere in the program.
- Local variables: Variables defined within a function. They are only accessible within that function and its nested functions.
Example:
Variable Scope Rules
- Variables defined within a function have local scope.
- Variables defined outside of functions have global scope.
- Inner functions can access variables from outer functions (closure).
Passing Arguments by Value or Reference
- Immutable types (numbers, strings, tuples): Arguments are passed by value, meaning a copy of the value is passed to the function.
- Mutable types (lists, dictionaries): Arguments are passed by reference, meaning the function can modify the original object.
Example:
In this example, my_list
is passed by reference, so the modification made within the function affects the original list.
Function Arguments
Positional Arguments
- Definition: Arguments passed to a function based on their position in the function call.
- Example:
Keyword Arguments
- Definition: Arguments passed to a function using keyword-argument pairs.
- Example:
Default Arguments
- Definition: Arguments that have a default value specified in the function definition.
- Example:
Variable-Length Arguments
*args
: Used to pass a variable number of positional arguments to a function.**kwargs
: Used to pass a variable number of keyword arguments to a function.
Example:
By understanding these different types of arguments, you can create more flexible and adaptable functions in your Python code.
Recursion: A Powerful Tool in Python
Understanding Recursion
Recursion is a programming technique where a function calls itself directly or indirectly. It's often used to solve problems that can be broken down into smaller, similar subproblems.
Recursive Functions
A recursive function has two essential parts:
- Base case: A condition that stops the recursion.
- Recursive case: A call to the function itself with modified arguments.
Example:
In this example, the base case is n == 0
, and the recursive case is n * factorial(n - 1)
.
Base Cases and Recursive Cases
- Base cases: Ensure that the recursion eventually terminates to avoid infinite loops.
- Recursive cases: Break down the problem into smaller subproblems and call the function recursively.
Tail Recursion Optimization
Tail recursion is a special case where the recursive call is the last operation in the function. In some languages (including Python in certain implementations), tail recursion can be optimized to avoid stack overflow errors for large recursion depths.
Example:
This function uses tail recursion to calculate the factorial.
Remember: While recursion can be a powerful tool, it's important to use it judiciously and avoid infinite recursion.
Best Practices for Functions
Naming Conventions
- Clear and descriptive names: Choose names that accurately reflect the function's purpose.
- Use verbs: Use verbs in function names to indicate actions.
- Avoid ambiguous names: Avoid using generic or misleading names.
Example:
Function Length and Complexity
- Keep functions short: Aim for functions that are no more than 20-30 lines long.
- Break down complex functions: If a function becomes too complex, consider breaking it down into smaller, more focused functions.
Code Readability and Maintainability
- Use comments: Explain the purpose of functions and complex logic.
- Consistent formatting: Follow consistent indentation and spacing.
- Meaningful variable names: Choose descriptive names for variables and parameters.
Testing Functions
- Write unit tests: Test functions individually to ensure they work as expected.
- Use testing frameworks: Consider using frameworks like pytest or unittest for automated testing.
Example:
By following these best practices, you can write functions that are well-structured, easy to understand, and maintainable.
Advanced Topics: Exploring Functional Programming in Python
Higher-Order Functions
Higher-order functions are functions that can take other functions as arguments or return functions as results. They are a fundamental concept in functional programming.
Examples of higher-order functions:
- map: Applies a function to each element of an iterable and returns a new iterable.
- filter: Filters elements from an iterable based on a predicate function.
- reduce: Applies a function to successive elements of an iterable and returns a single value.
Example:
Lambda Functions
Lambda functions, also known as anonymous functions, are concise ways to define functions without giving them a name. They are often used as arguments to higher-order functions.
Example:
Decorators
Decorators are a powerful mechanism in Python that allow you to modify the behavior of functions or classes without directly altering their code. They are implemented using functions that take other functions as arguments and return new functions.
Example:
In this example, the my_decorator
function is applied to the greet
function using the @
syntax. This modifies the behavior of the greet
function, adding additional logging before and after the function call.
By understanding higher-order functions, lambda functions, and decorators, you can write more expressive and concise Python code.