CSS Basics: Selectors, Properties, and Values for Styling Your Website
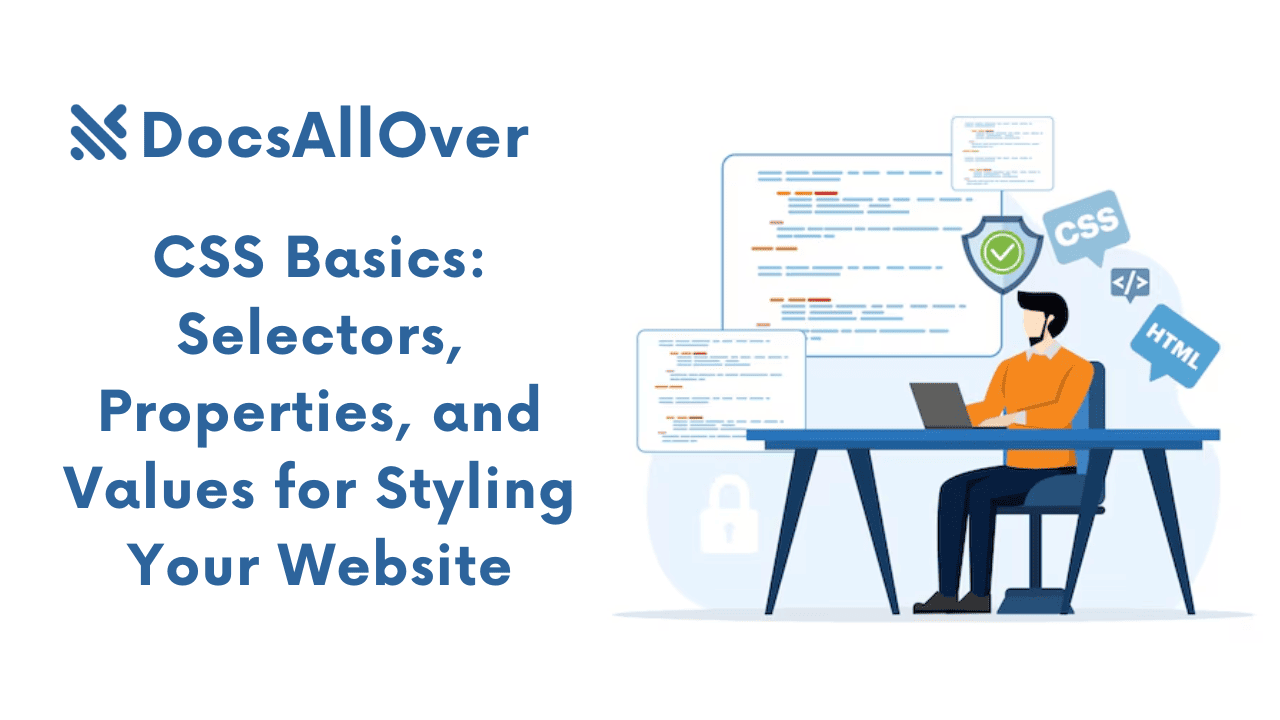
What is CSS?
CSS, or Cascading Style Sheets, is a style sheet language used to describe the presentation of a document written in a markup language like HTML. It controls the layout, visual format, and overall appearance of a webpage.
Why is CSS Important?
- Separation of Concerns: CSS separates the content (HTML) from the presentation (CSS), making your code more organized and maintainable.
- Consistent Styling: You can apply styles consistently across multiple pages of your website.
- Improved Web Performance: By optimizing CSS, you can reduce page load times.
- Enhanced User Experience: CSS allows you to create visually appealing and user-friendly websites.
Basic Structure of a CSS Stylesheet
A CSS stylesheet typically consists of three main parts:
- Selector: Specifies the HTML element(s) you want to style.
- Property: The specific style attribute you want to apply.
- Value: The value assigned to the property.
Example:
In this example, the p
selector targets all paragraph elements. The color
property sets the text color to blue, and the font-size
property sets the font size to 16 pixels.
Ways to Link a CSS Stylesheet to an HTML Document:
- Internal Style Sheet:
- External Style Sheet:
In this case, you create a separate
.css
file and link it to your HTML file.
CSS Selectors
CSS selectors are used to target specific HTML elements to which you want to apply styles. Here are the main types of selectors:
Element Selectors
- Target elements based on their tag name.
- Syntax:
element_name
This will style all paragraph elements on the page.
Class Selectors
- Target elements based on a class attribute.
- Syntax:
.class_name
You can apply this class to multiple elements as shown above.
ID Selectors
- Target a specific element based on a unique ID attribute.
- Syntax:
#id_name
You should use IDs sparingly, as they should be unique within a document.
Attribute Selectors
- Target elements based on their attributes.
- Syntax:
[attribute_name="value"]
This will style all anchor links that start with "https://".
Pseudo-classes
Target elements based on their state or position in the document.
Examples:
:hover
: Styles an element when the mouse hovers over it.:active
: Styles an element when it is active (e.g., a clicked link).:focus
: Styles an element when it has focus (e.g., a focused input field).:first-child
: Styles the first child element of its parent.:last-child
: Styles the last child element of its parent.
Pseudo-elements
Target specific parts of an element.
Examples:
::before
: Inserts content before the element.::after
: Inserts content after the element.::first-letter
: Styles the first letter of an element.::first-line
: Styles the first line of an element.
By understanding these selectors, you can precisely target specific elements and apply custom styles to them.
CSS Properties and Values
CSS properties are used to define the visual style of HTML elements. Here are some of the most common CSS properties:
Font Properties
- font-family: Specifies the font family for the text.
- font-size: Sets the font size.
- font-weight: Sets the font weight (e.g., bold, normal).
- font-style: Sets the font style (e.g., italic, normal).
- font-variant: Sets the font variant (e.g., small-caps).
Color Properties
- color: Sets the color of the text.
- background-color: Sets the background color of an element.
- opacity: Sets the opacity of an element.
Background Properties
- background-color: Sets the background color.
- background-image: Sets a background image.
- background-repeat: Sets how the background image is repeated.
- background-position: Sets the position of the background image.
- background-size: Sets the size of the background image.
- background-attachment: Sets whether the background image is fixed or scrolls with the content.
Border Properties
- border-width: Sets the width of the border.
- border-style: Sets the style of the border (e.g., solid, dashed, dotted).
- border-color: Sets the color of the border.
- border-radius: Sets the radius of the border's corners.
Box Model Properties
- margin: Sets the margin around an element.
- padding: Sets the padding around an element's content.
- border: Sets the border around an element.
Text Properties
- text-align: Sets the horizontal alignment of text.
- text-decoration: Sets text decorations like underline or strikethrough.
- text-transform: Sets text transformations like uppercase or lowercase.
- text-shadow: Adds a shadow effect to text.
Layout Properties
- display: Sets how an element is displayed (e.g., block, inline, inline-block, flex, grid).
- position: Sets the positioning of an element (e.g., static, relative, absolute, fixed).
- float: Floats an element to the left or right of its container.
- clear: Clears floats from previous elements.
Transform and Transition Properties
- transform: Applies transformations to an element (e.g., rotate, scale, translate).
- transition: Creates smooth transitions between different styles.
CSS Box Model
The CSS Box Model is a fundamental concept in CSS that explains how elements are structured and laid out on a web page. It consists of four main components:
- Content: The actual content of the element, such as text or images.
- Padding: The space between the content and the border.
- Border: The border that surrounds the padding and content.
- Margin: The space outside the border, separating the element from other elements.
Controlling the Layout of Elements
You can manipulate these components to control the layout of elements on your web page. Here are some key properties:
- margin: Sets the space outside the border.
margin-top
,margin-right
,margin-bottom
,margin-left
margin: 10px;
(sets all sides to 10px)
- padding: Sets the space inside the border.
padding-top
,padding-right
,padding-bottom
,padding-left
padding: 10px;
(sets all sides to 10px)
- border: Sets the border style, width, and color.
border: 1px solid black;
- width: Sets the width of the element.
- height: Sets the height of the element.
Example:
This CSS code will create a div with a width of 300px, a height of 200px, a 1px black border, 20px of padding, and a 10px margin on all sides.
CSS Layout Techniques
CSS offers various techniques to control the layout of elements on a web page. Here are some of the most common techniques:
CSS Positioning
- Static Positioning:
- Default positioning.
- Elements are positioned according to the normal document flow.
- Relative Positioning:
- Elements are positioned relative to their normal position.
- Use
top
,bottom
,left
, andright
properties to adjust the position.
- Absolute Positioning:
- Elements are positioned relative to the nearest positioned ancestor or the viewport.
- Use
top
,bottom
,left
, andright
properties to position the element.
- Fixed Positioning:
- Elements are positioned relative to the viewport and remain fixed even when the page is scrolled.
- Use
top
,bottom
,left
, andright
properties to position the element.
- Sticky Positioning:
- Elements are positioned relative to the viewport until they hit a specified threshold, then they become fixed.
Flexbox Layout
Flexbox is a powerful layout model that allows you to arrange items flexibly within a container. It's ideal for creating responsive layouts, aligning items, and distributing space.
Key Flexbox Properties:
display: flex;
flex-direction
: Controls the main axis of the flex container.flex-wrap
: Controls whether items wrap to the next line.justify-content
: Aligns items along the main axis.align-items
: Aligns items along the cross axis.flex-grow
: Controls how items grow to fill available space.flex-shrink
: Controls how items shrink to fit within the container.flex-basis
: Sets the initial size of an item.
Grid Layout
Grid layout is another powerful layout model that allows you to create complex layouts by dividing a page into rows and columns.
Key Grid Layout Properties:
display: grid;
grid-template-rows
: Defines the rows of the grid.grid-template-columns
: Defines the columns of the grid.grid-gap
: Sets the gap between rows and columns.grid-template-areas
: Defines named areas within the grid.grid-auto-rows
: Sets the size of automatically created rows.grid-auto-columns
: Sets the size of automatically created columns.
Best Practices for Writing CSS
CSS Organization and Structure
- Modular CSS: Break down your CSS into smaller, more manageable files based on components or pages.
- CSS Preprocessors: Use preprocessors like Sass or Less to organize your CSS, write cleaner code, and use advanced features like variables, mixins, and nesting.
- CSS Frameworks: Consider using CSS frameworks like Bootstrap or Tailwind CSS to accelerate development and ensure consistency.
CSS Specificity and Inheritance
- Specificity: Understand how CSS specificity works to determine which styles are applied to an element.
- More specific selectors (e.g., IDs, classes, inline styles) override less specific ones.
- Inheritance: Child elements inherit styles from their parent elements.
Using CSS Preprocessors
- Sass and Less: These preprocessors offer features like variables, mixins, nesting, and partials, which can help you write more efficient and maintainable CSS.
- Benefits:
- Improved code organization and reusability
- Faster development workflow
- Enhanced maintainability
Cross-Browser Compatibility
- CSS Reset: Use a CSS reset to normalize styles across different browsers.
- Browser Testing: Test your website on different browsers and devices to identify and fix compatibility issues.
- CSS Frameworks: Use well-maintained CSS frameworks that have built-in cross-browser compatibility.
- CSS Compatibility Tables: Refer to compatibility tables to check browser support for specific CSS features.