Bootstrap 4 Login Form with Social Media Integration
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <!-- Bootstrap CSS --> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.0.0/dist/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous"> <link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.7.2/css/all.css"> <style> @import url('https://fonts.googleapis.com/css2?family=Poppins&display=swap'); * { padding: 0; margin: 0; box-sizing: border-box; font-family: 'Poppins', sans-serif } body { height: 100vh; background: linear-gradient(to top, #c9c9ff 50%, #9090fa 90%) no-repeat } </style> </head> <body> <div class="container"> <div class="row"> <div class="offset-md-2 col-lg-5 col-md-7 offset-lg-4 offset-md-3"> <div class="panel border bg-white"> <div class="panel-heading"> <h3 class="pt-3 font-weight-bold">Login</h3> </div> <div class="panel-body p-3"> <form action="login_script.php" method="POST"> <div class="form-group py-2"> <div class="input-field"> <span class="far fa-user p-2"></span> <input type="text" placeholder="Username or Email" required> </div> </div> <div class="form-group py-1 pb-2"> <div class="input-field"> <span class="fas fa-lock px-2"></span> <input type="password" placeholder="Enter your Password" required> <button class="btn bg-white text-muted"> <span class="far fa-eye-slash"></span> </button> </div> </div> <div class="form-inline"> <input type="checkbox" name="remember" id="remember"> <label for="remember" class="text-muted">Remember me</label> <a href="#" id="forgot" class="font-weight-bold">Forgot password?</a> </div> <div class="btn btn-primary btn-block mt-3">Login</div> <div class="text-center pt-4 text-muted">Don't have an account? <a href="#">Sign up</a> </div> </form> </div> <div class="mx-3 my-2 py-2 bordert"> <div class="text-center py-3"> <a href="https://wwww.facebook.com" target="_blank" class="px-2"> <img src="https://www.dpreview.com/files/p/articles/4698742202/facebook.jpeg" alt=""> </a> <a href="https://www.google.com" target="_blank" class="px-2"> <img src="https://www.freepnglogos.com/uploads/google-logo-png/google-logo-png-suite-everything-you-need-know-about-google-newest-0.png" alt=""> </a> <a href="https://www.github.com" target="_blank" class="px-2"> <img src="https://www.freepnglogos.com/uploads/512x512-logo-png/512x512-logo-github-icon-35.png" alt=""> </a> </div> </div> </div> </div> </div> </div> <!-- Optional JavaScript --> <!-- jQuery first, then Popper.js, then Bootstrap JS --> <script src="https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity="sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin="anonymous"> </script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.12.9/dist/umd/popper.min.js" integrity="sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin="anonymous"> </script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.0.0/dist/js/bootstrap.min.js" integrity="sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin="anonymous"> </script> </body> </html>
.container { margin: 50px auto } .panel-heading { text-align: center; margin-bottom: 10px } #forgot { min-width: 100px; margin-left: auto; text-decoration: none } a:hover { text-decoration: none } .form-inline label { padding-left: 10px; margin: 0; cursor: pointer } .btn.btn-primary { margin-top: 20px; border-radius: 15px } .panel { min-height: 380px; box-shadow: 20px 20px 80px rgb(218, 218, 218); border-radius: 12px } .input-field { border-radius: 5px; padding: 5px; display: flex; align-items: center; cursor: pointer; border: 1px solid #ddd; color: #4343ff } input[type='text'], input[type='password'] { border: none; outline: none; box-shadow: none; width: 100% } .fa-eye-slash.btn { border: none; outline: none; box-shadow: none } img { width: 40px; height: 40px; object-fit: cover; border-radius: 50%; position: relative } a[target='_blank'] { position: relative; transition: all 0.1s ease-in-out } .bordert { border-top: 1px solid #aaa; position: relative } .bordert:after { content: "or connect with"; position: absolute; top: -13px; left: 33%; background-color: #fff; padding: 0px 8px } @media(max-width: 360px) { #forgot { margin-left: 0; padding-top: 10px } body { height: 100% } .container { margin: 30px 0 } .bordert:after { left: 25% } }
//Doesn't require any JS.
This Bootstrap 4 snippet provides a visually appealing and user-friendly login form that allows users to sign in using their existing social media accounts. It offers a convenient and efficient way for users to access your website or application without requiring them to create a new account. The login form is designed to be responsive and customizable, ensuring a seamless user experience across different devices.
Key Features:
- Social Media Integration: The login form integrates with popular social media platforms like Facebook, Google, and GitHub, allowing users to sign in using their existing credentials.
- Responsive Layout: The form adapts to various screen sizes, providing a consistent user experience on desktops, tablets, and mobile devices.
- Clear Input Labels: The input fields are accompanied by clear and concise labels, guiding users in entering their login information.
- Customizable Styling: Easily modify the appearance of the login form using Bootstrap's CSS classes to match your website's design and branding.
Implementation:
- Include Bootstrap 4: Ensure you have Bootstrap 4 installed and configured in your project.
- Create the HTML Structure: Set up the basic HTML structure for the login form, including the container, input fields, submit button, and social media login buttons.
- Apply Bootstrap Classes: Use Bootstrap's CSS classes to style the login form and its elements, such as form, form-group, input, btn, card, and social-login-button.
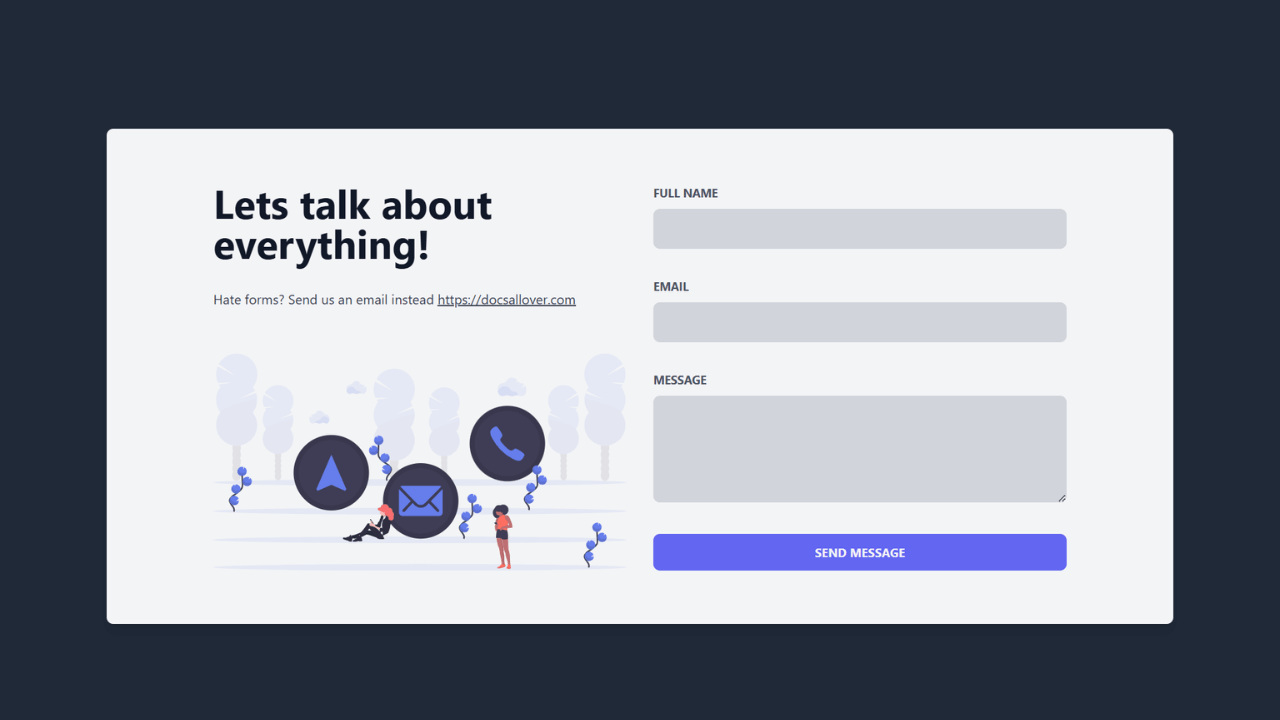
Stylish Contact Form with Image in Tailwind CSS
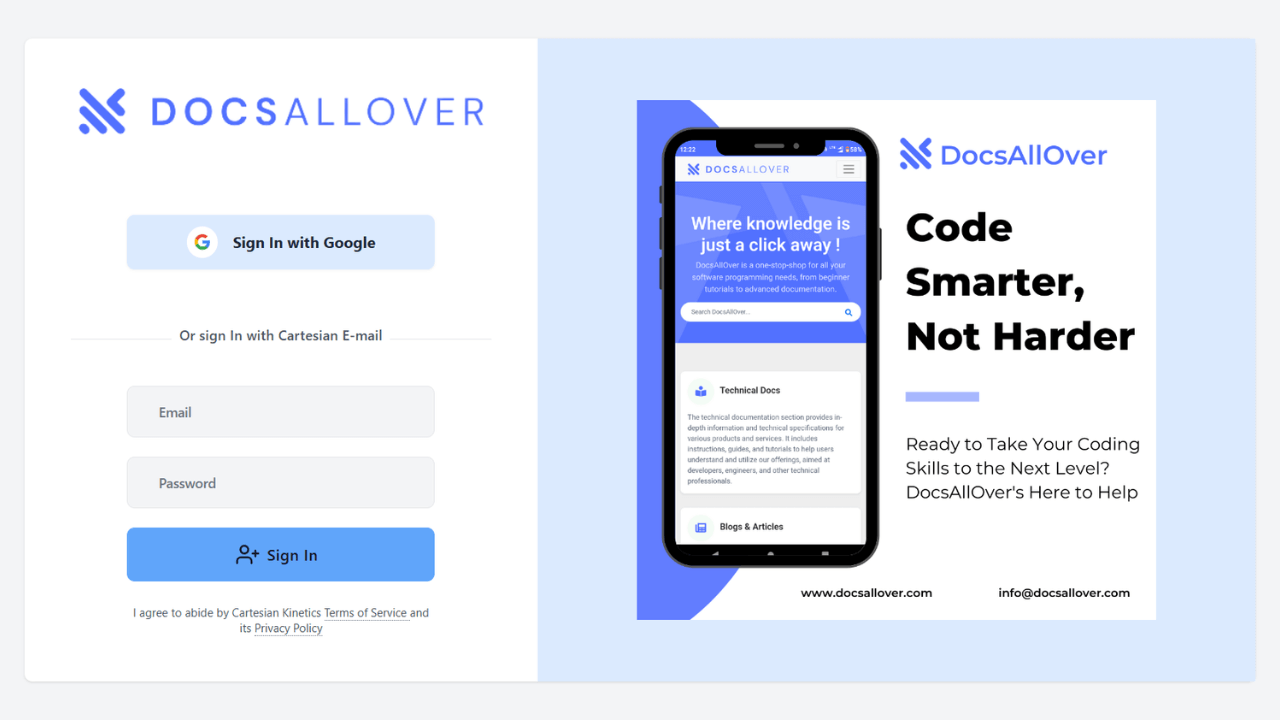
Responsive Registration Form with Image in Tailwind CSS
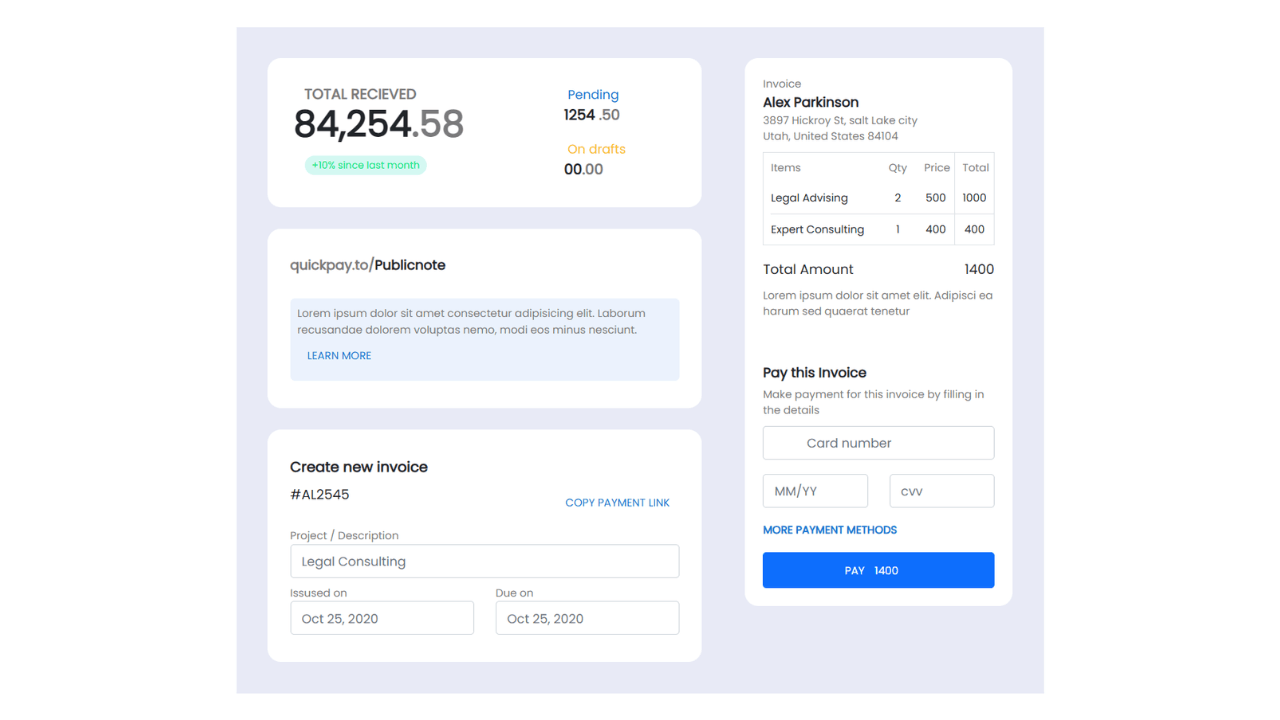
Bootstrap 5 Payment Method Form Page with Invoice
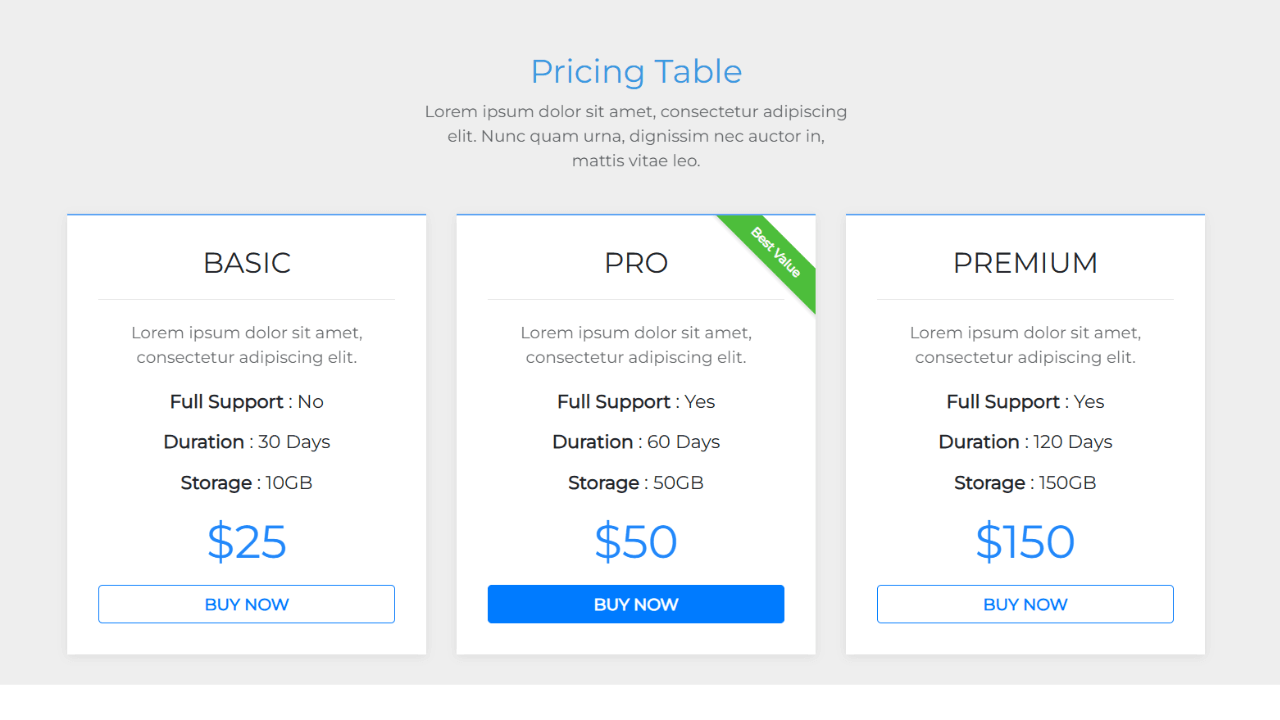
Modern Pricing Table Design with Bootstrap 4
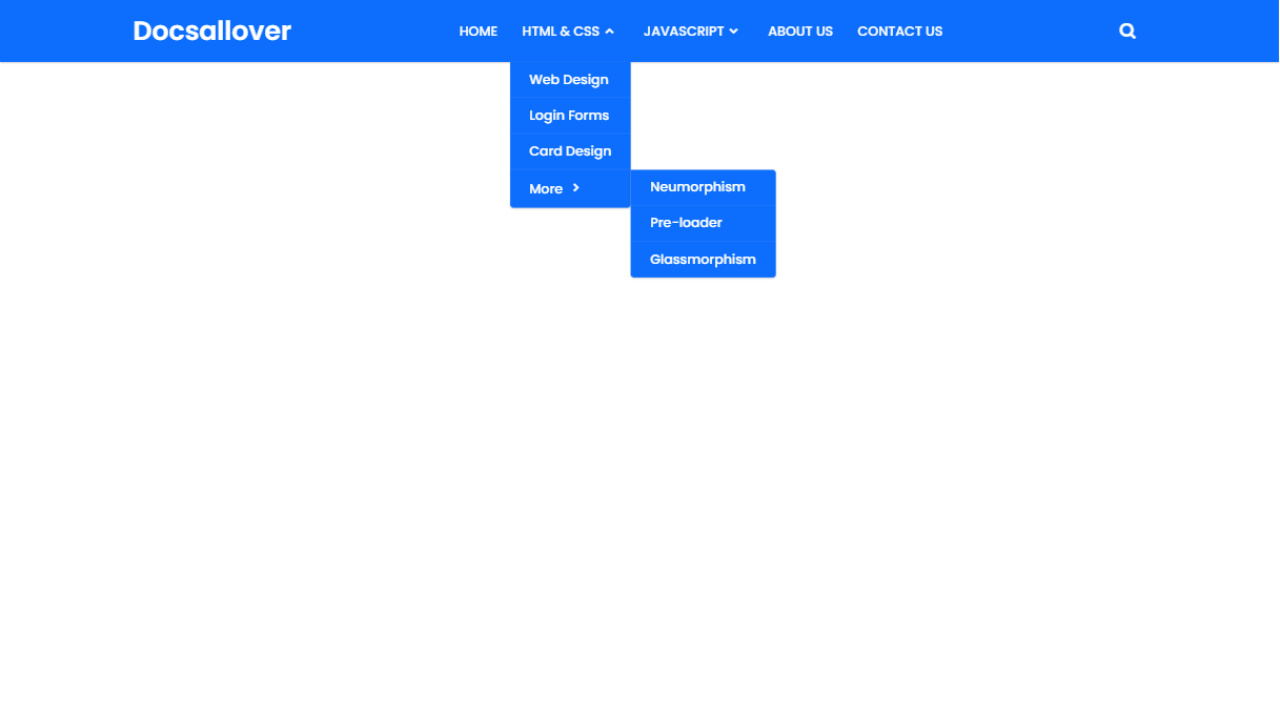
Responsive Multi-Level Drop-Down Navigation Menu in CSS
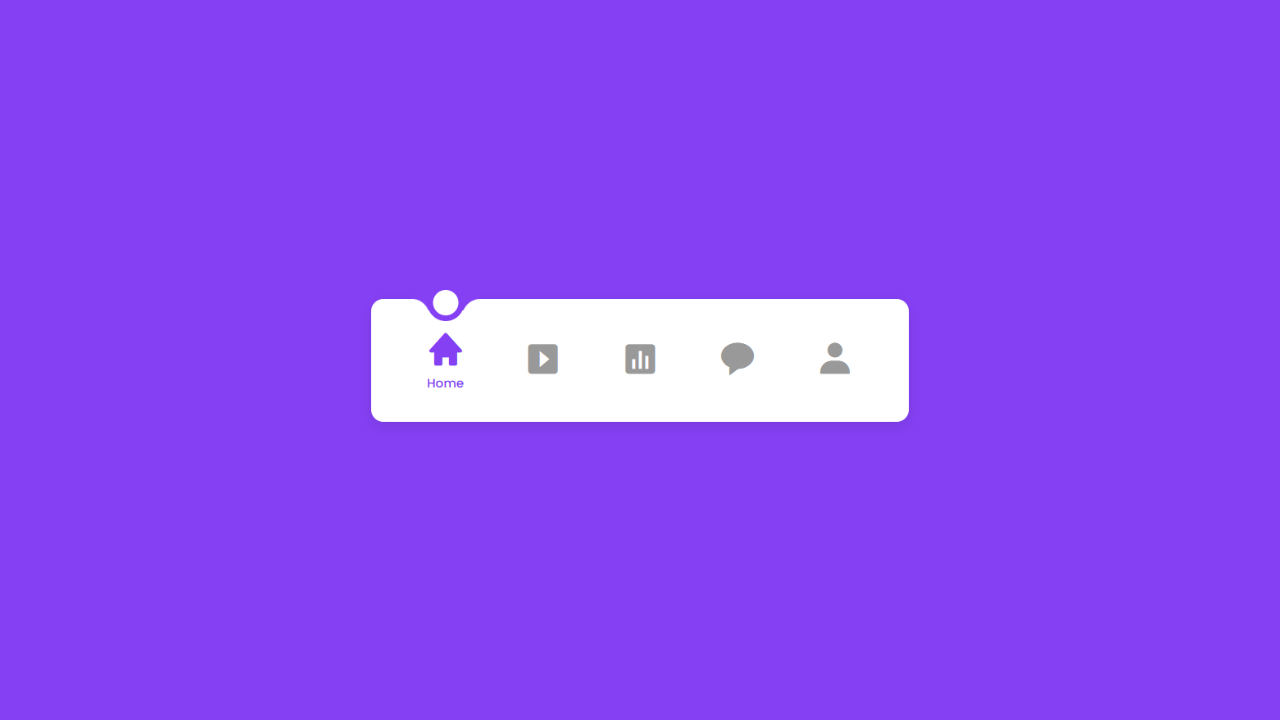
Interactive Bottom Navigation Bar Indicator with CSS

Real-time Password Strength Indicator in Bootstrap 5
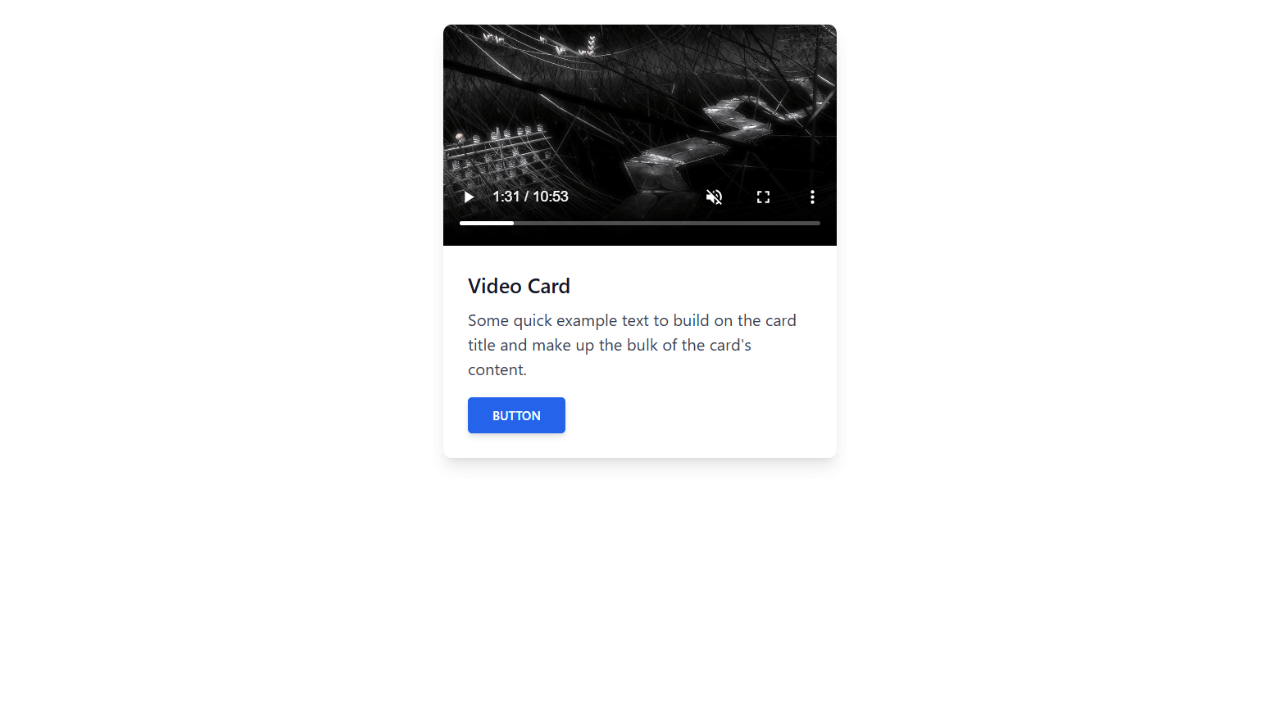
Responsive Video Card Component for Tailwind CSS
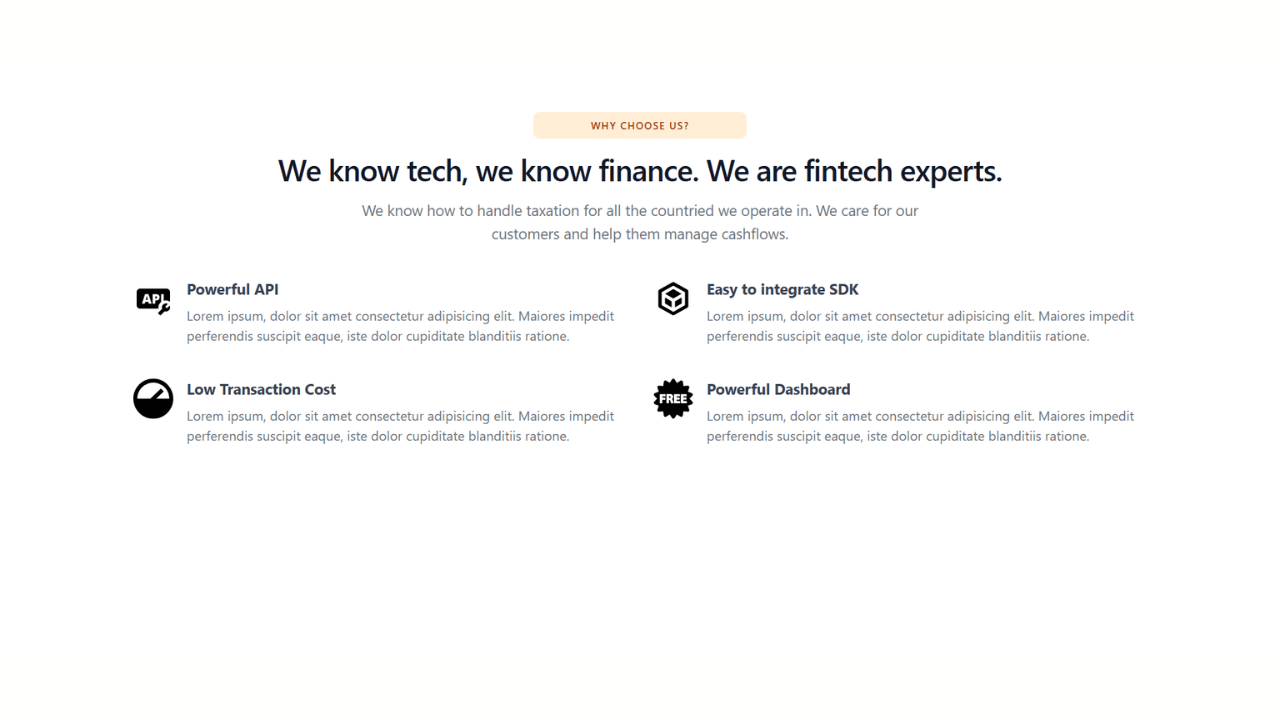
Responsive Feature Highlight Component with Tailwind CSS
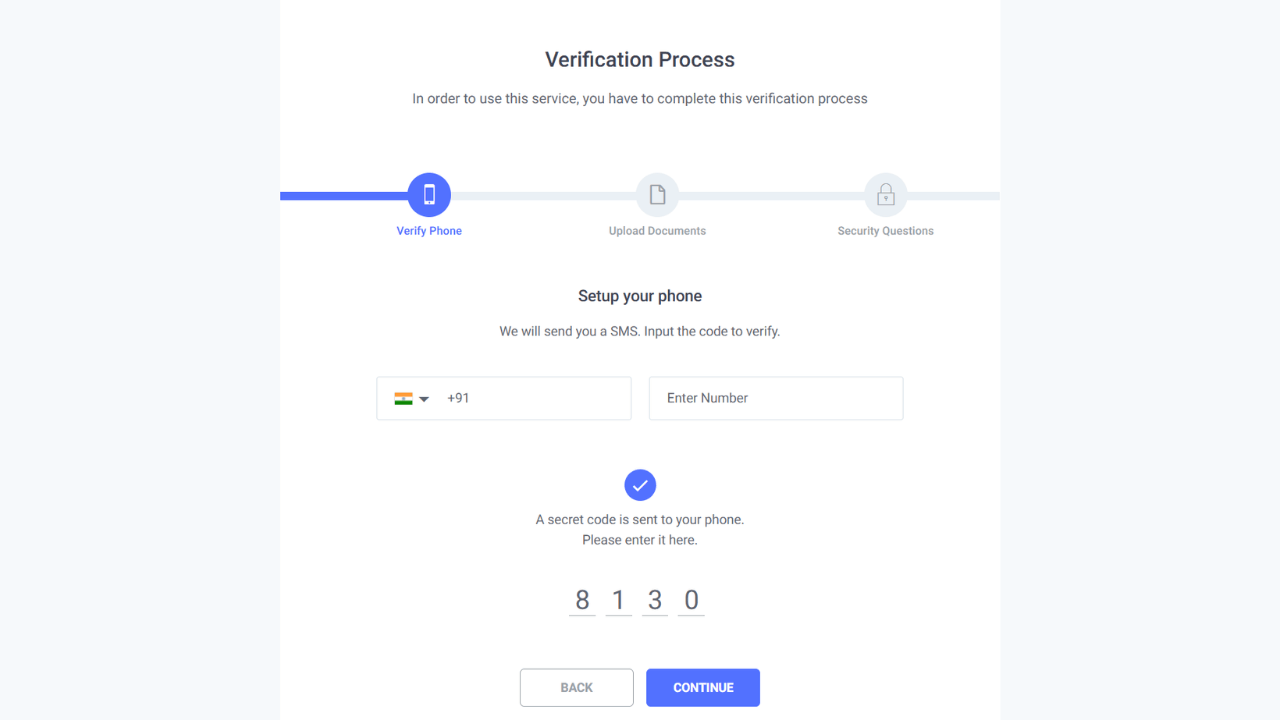
Multi-Step Form with International Phone Input and Nice Select
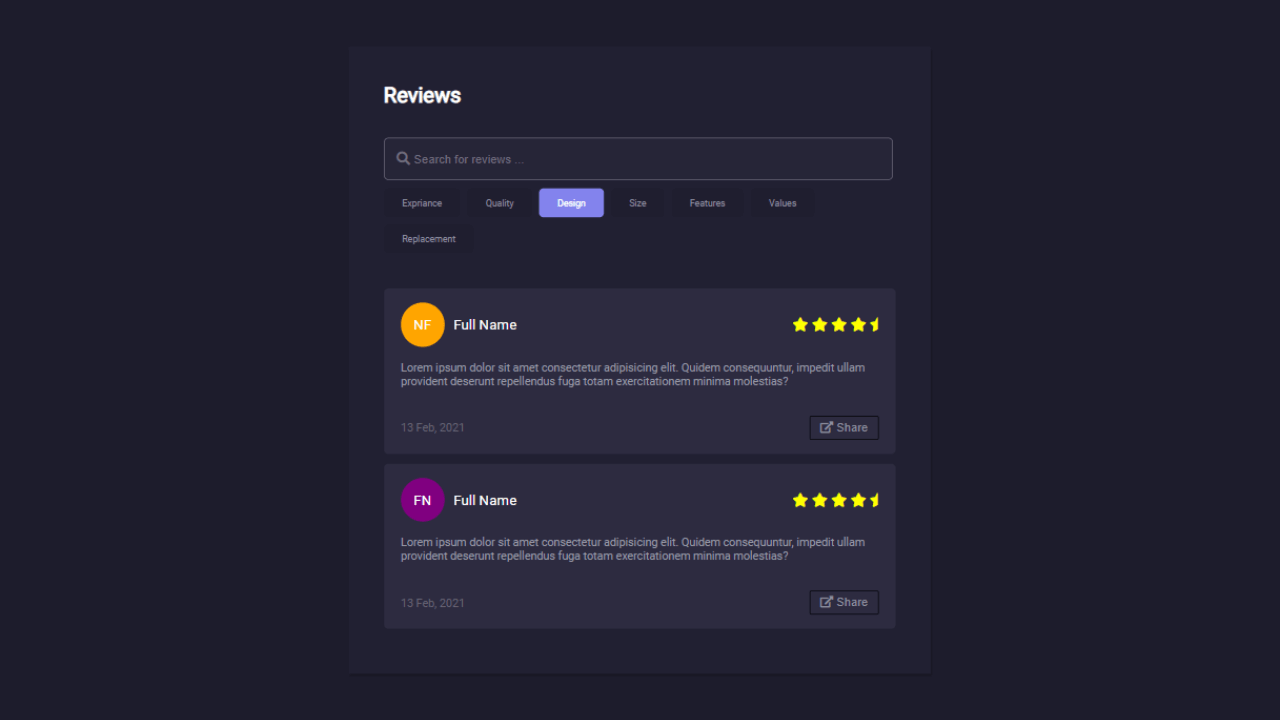
Responsive Review Cards Component with CSS3
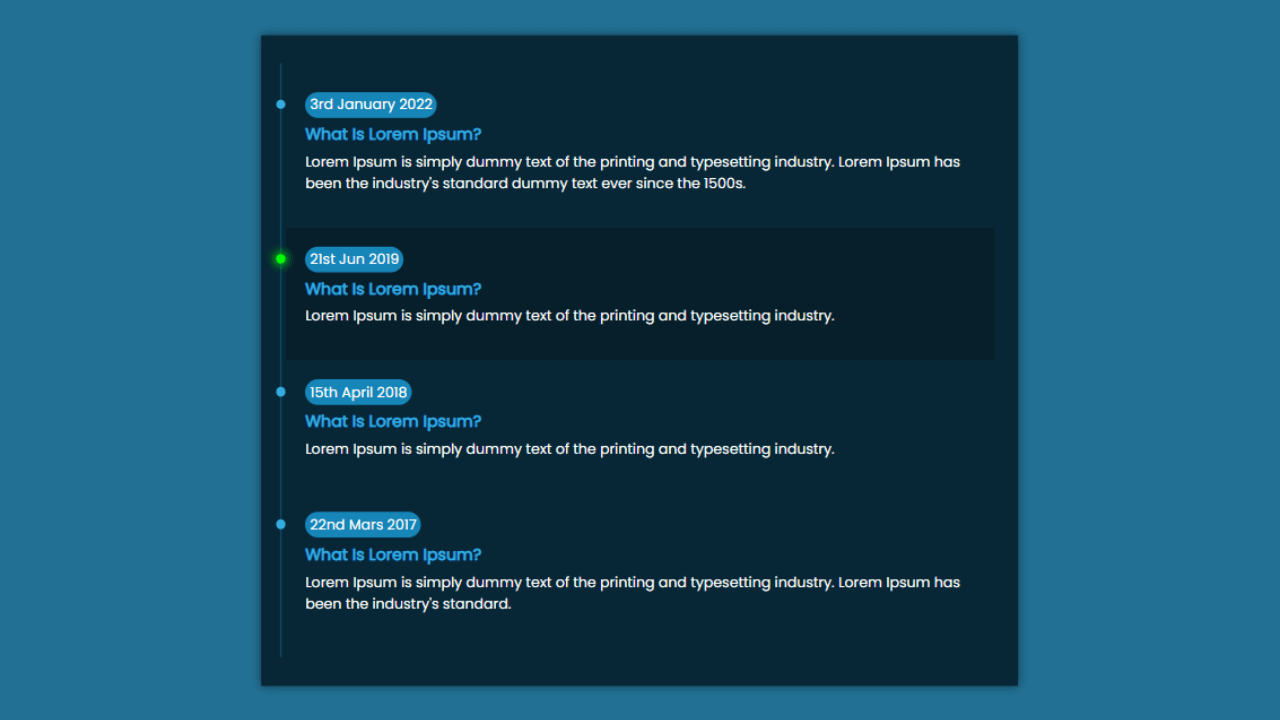
Responsive CSS3 Timeline Design With Hover Effects
Building Blocks for Your Web Pages
Explore a collection of pre-written HTML,CSS and Javascript
snippets to jumpstart your web development projects.