Fancy User Login Form in Bootstrap 5 with Background Gradient Color
<!doctype html> <html> <head> <meta charset='utf-8'> <meta name='viewport' content='width=device-width, initial-scale=1'> <link href='https://stackpath.bootstrapcdn.com/bootstrap/5.0.0-alpha1/css/bootstrap.min.css' rel='stylesheet'> <link href='https://use.fontawesome.com/releases/v5.7.2/css/all.css' rel='stylesheet'> <style> @import url('https://fonts.googleapis.com/css2?family=Kaushan+Script&family=Poppins&display=swap'); * { padding: 0; margin: 0; box-sizing: border-box } body { background-color: #eee; height: 100vh; font-family: 'Poppins', sans-serif; background: linear-gradient(to top, #fff 10%, rgba(93, 42, 141, 0.4) 90%) no-repeat } .wrapper { max-width: 500px; border-radius: 10px; margin: 50px auto; padding: 30px 40px; box-shadow: 20px 20px 80px rgb(206, 206, 206) } .h2 { font-family: 'Kaushan Script', cursive; font-size: 3.5rem; font-weight: bold; color: #400485; font-style: italic } .h4 { font-family: 'Poppins', sans-serif } .input-field { border-radius: 5px; padding: 5px; display: flex; align-items: center; cursor: pointer; border: 1px solid #400485; color: #400485 } .input-field:hover { color: #7b4ca0; border: 1px solid #7b4ca0 } input { border: none; outline: none; box-shadow: none; width: 100%; padding: 0px 2px; font-family: 'Poppins', sans-serif } .fa-eye-slash.btn { border: none; outline: none; box-shadow: none } a { text-decoration: none; color: #400485; font-weight: 700 } a:hover { text-decoration: none; color: #7b4ca0 } .option { position: relative; padding-left: 30px; cursor: pointer } .option label.text-muted { display: block; cursor: pointer } .option input { display: none } .checkmark { position: absolute; top: 3px; left: 0; height: 20px; width: 20px; background-color: #fff; border: 1px solid #ddd; border-radius: 50%; cursor: pointer } .option input:checked~.checkmark:after { display: block } .option .checkmark:after { content: ""; width: 13px; height: 13px; display: block; background: #400485; position: absolute; top: 48%; left: 53%; border-radius: 50%; transform: translate(-50%, -50%) scale(0); transition: 300ms ease-in-out 0s } .option input[type="radio"]:checked~.checkmark { background: #fff; transition: 300ms ease-in-out 0s; border: 1px solid #400485 } .option input[type="radio"]:checked~.checkmark:after { transform: translate(-50%, -50%) scale(1) } .btn.btn-block { border-radius: 20px; background-color: #400485; color: #fff } .btn.btn-block:hover { background-color: #55268be0 } @media(max-width: 575px) { .wrapper { margin: 10px } } @media(max-width:424px) { .wrapper { padding: 30px 10px; margin: 5px } .option { position: relative; padding-left: 22px } .option label.text-muted { font-size: 0.95rem } .checkmark { position: absolute; top: 2px } .option .checkmark:after { top: 50% } #forgot { font-size: 0.95rem } } </style> </head> <body> <div class="wrapper bg-white"> <div class="h2 text-center">Docsallover</div> <div class="h4 text-muted text-center pt-2">Enter your login details</div> <form class="pt-3"> <div class="form-group py-2"> <div class="input-field"> <span class="far fa-user p-2"></span> <input type="text" placeholder="Username or Email Address" required class=""> </div> </div> <div class="form-group py-1 pb-2"> <div class="input-field"> <span class="fas fa-lock p-2"></span> <input type="text" placeholder="Enter your Password" required class=""> <button class="btn bg-white text-muted"> <span class="far fa-eye-slash"></span> </button> </div> </div> <div class="d-flex align-items-start"> <div class="remember"> <label class="option text-muted"> Remember me <input type="radio" name="radio"> <span class="checkmark"></span> </label> </div> <div class="ml-auto"> <a href="#" id="forgot">Forgot Password?</a> </div> </div> <button class="btn btn-block text-center my-3">Log in</button> <div class="text-center pt-3 text-muted">Not a member? <a href="#">Sign up</a></div> </form> </div> <script type='text/javascript' src='https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js'></script> <script type='text/javascript' src='https://stackpath.bootstrapcdn.com/bootstrap/5.0.0-alpha1/js/bootstrap.min.js'></script> </body> </html>
/* Doesn't require any CSS. */
//Doesn't require any JS.
This Bootstrap 5 snippet provides a visually appealing and user-friendly login form with a modern design. It features a clean layout, intuitive input fields, and a visually striking color scheme. The login form is designed to be responsive and customizable, ensuring a seamless user experience across different devices.
Key Features:
- Modern Design: The login form incorporates a sleek and contemporary design, enhancing the overall aesthetic of your website.
- Responsive Layout: The form adapts to various screen sizes, providing a consistent user experience on desktops, tablets, and mobile devices.
- Clear Input Labels: The input fields are accompanied by clear and concise labels, guiding users in entering their credentials.
- Placeholder Text: Placeholder text within the input fields provides helpful hints to users, reducing the need for additional instructions.
- Customizable Styling: Easily modify the appearance of the login form using Bootstrap's CSS classes to match your website's design and branding.
Implementation:
- Include Bootstrap 5: Ensure you have Bootstrap 5 installed and configured in your project.
- Create the HTML Structure: Set up the basic HTML structure for the login form, including the container, input fields, and submit button.
- Apply Bootstrap Classes: Use Bootstrap's CSS classes to style the login form and its elements, such as form, form-group, input, btn, and card.
- Customize as Needed: Modify the Bootstrap classes and HTML structure to customize the appearance and behavior of the login form according to your specific requirements.
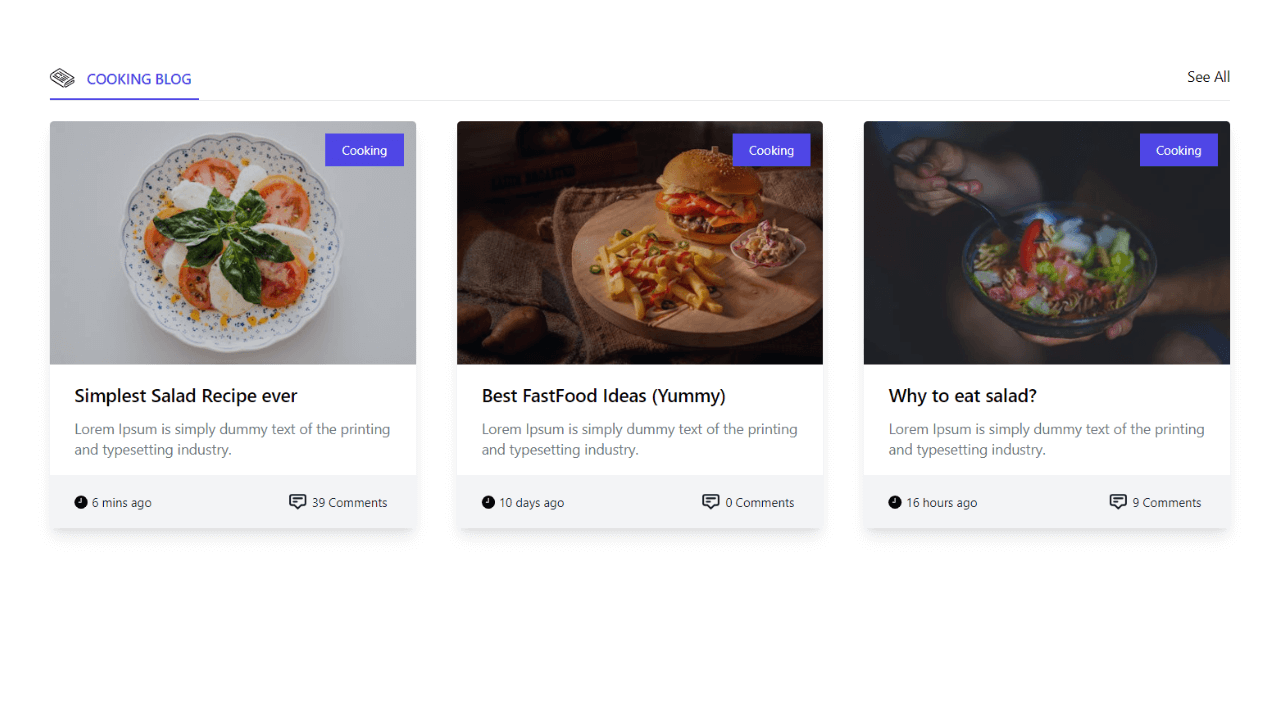
Responsive Blog Grid with Tailwind CSS

User-Friendly Travel Booking Form in Bootstrap 5
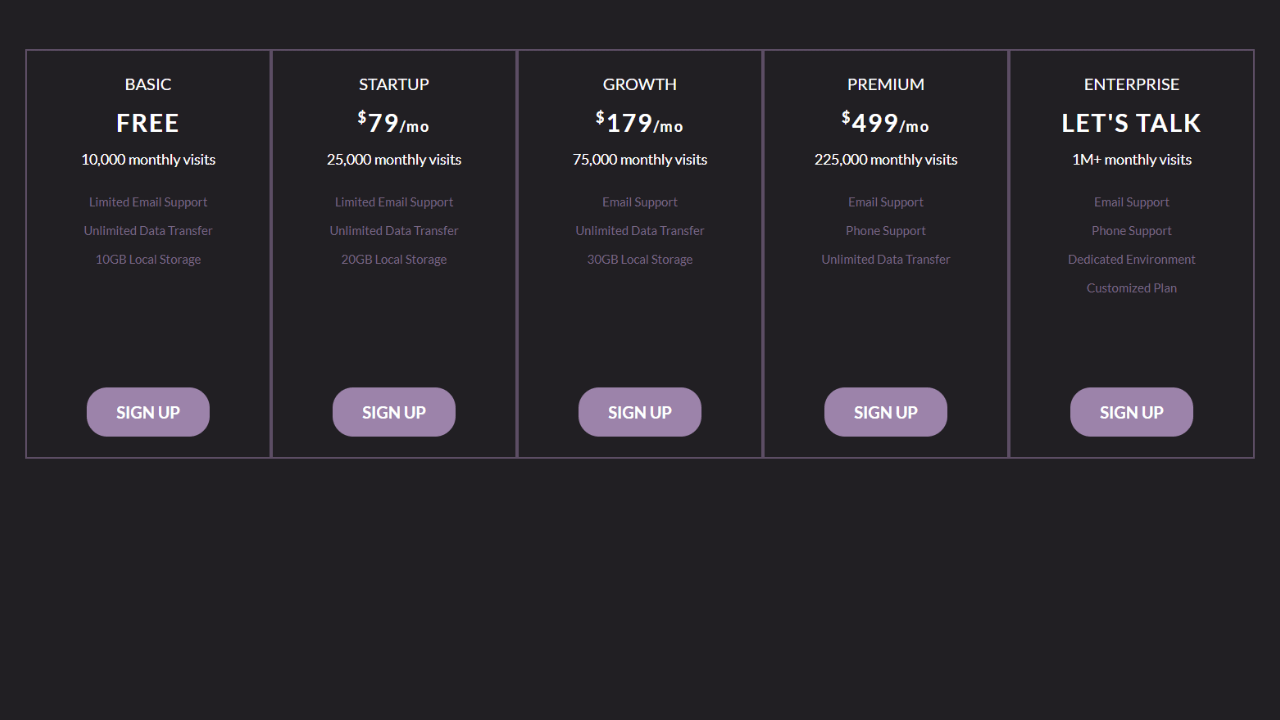
Responsive CSS3 Pricing Table with Hover Animation
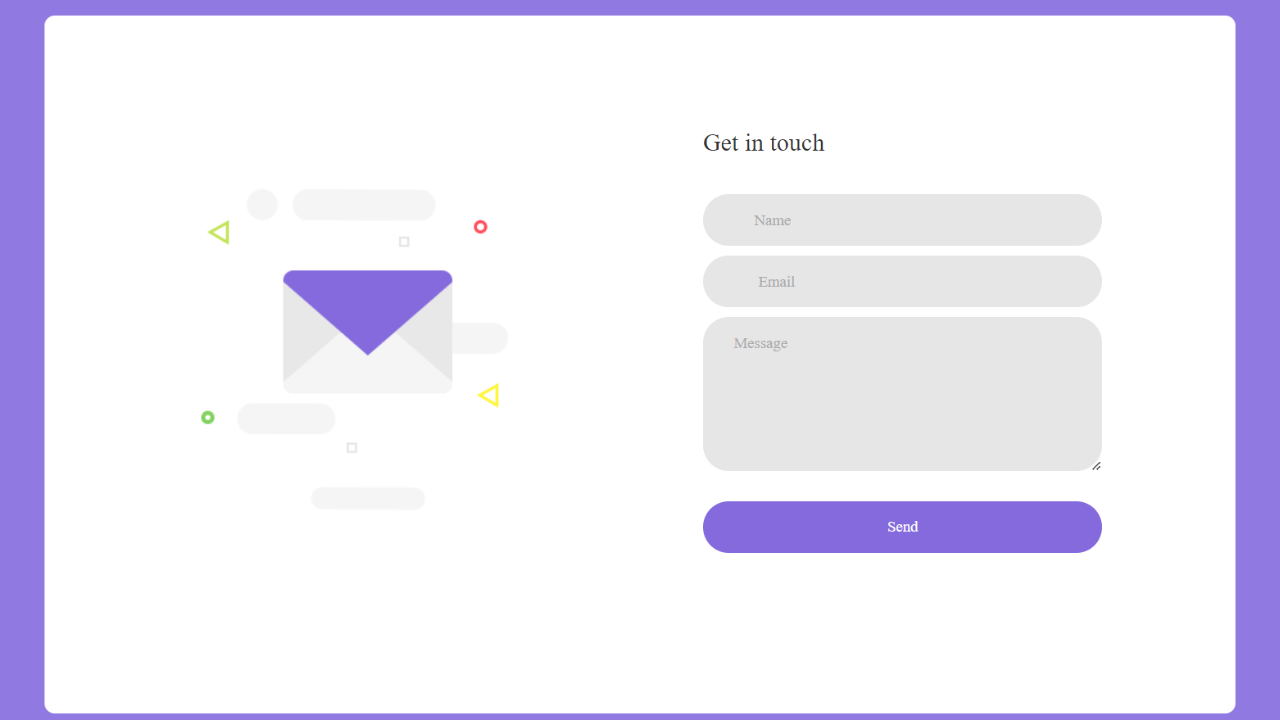
Responsive Contact Us Forms in Bootstrap 5
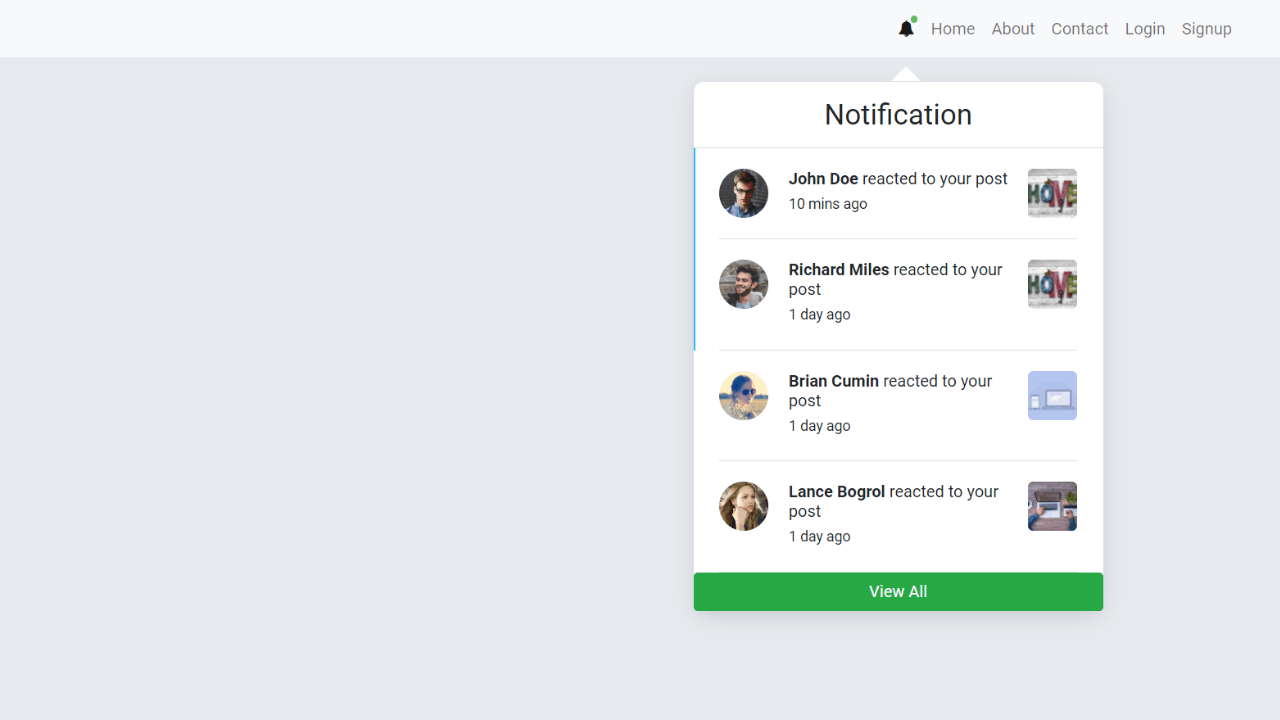
Notification UI Design with Navbar in Bootstrap 5
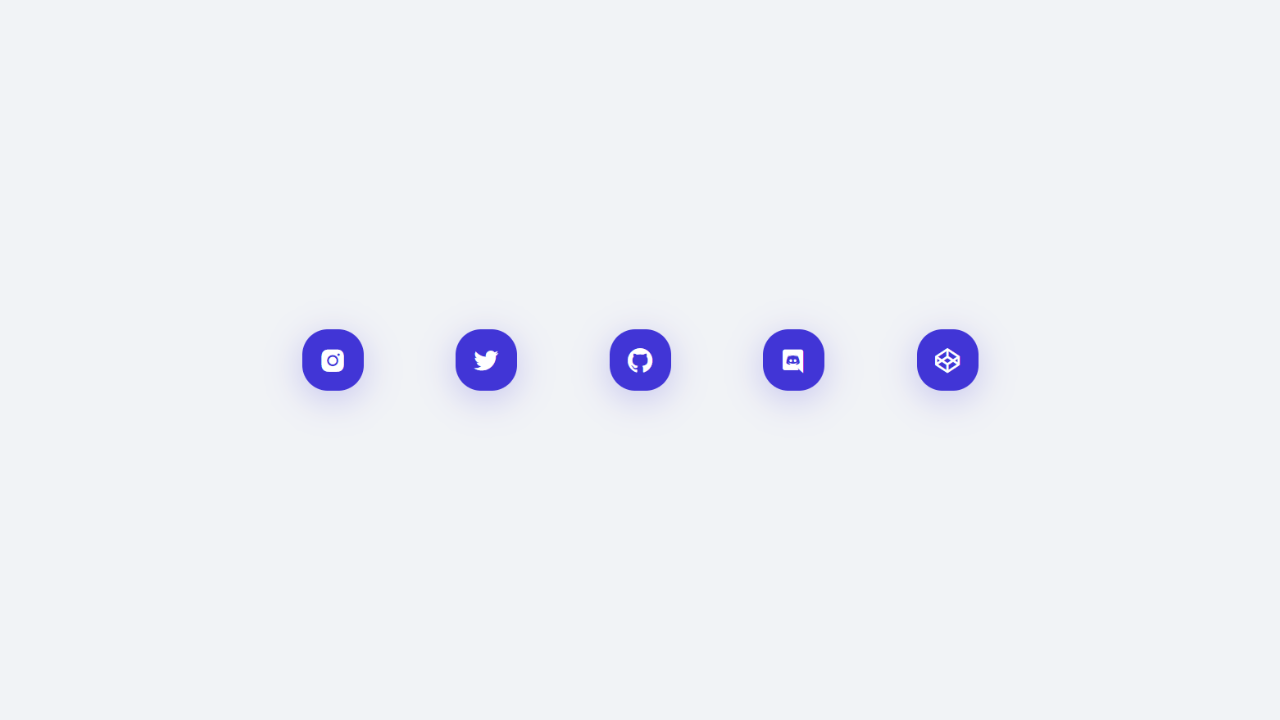
Interactive Social Media Buttons with CSS and JS
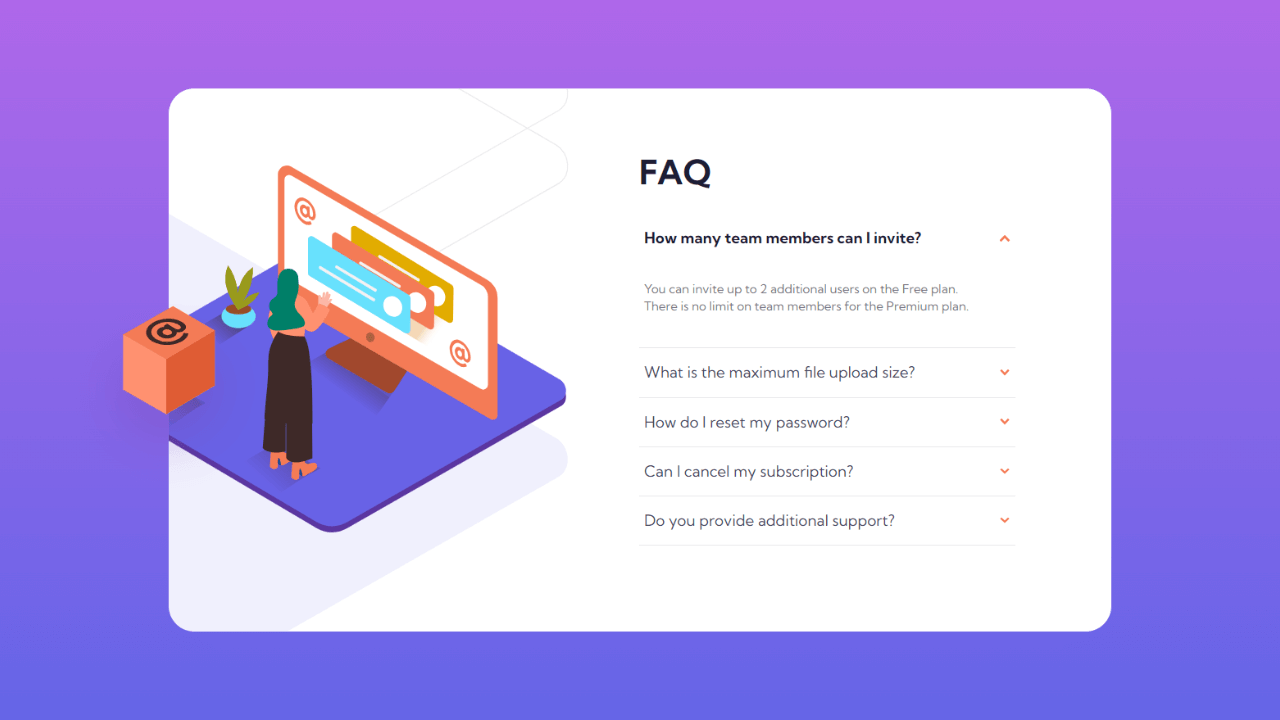
Lightweight FAQ Accordion Component with CSS
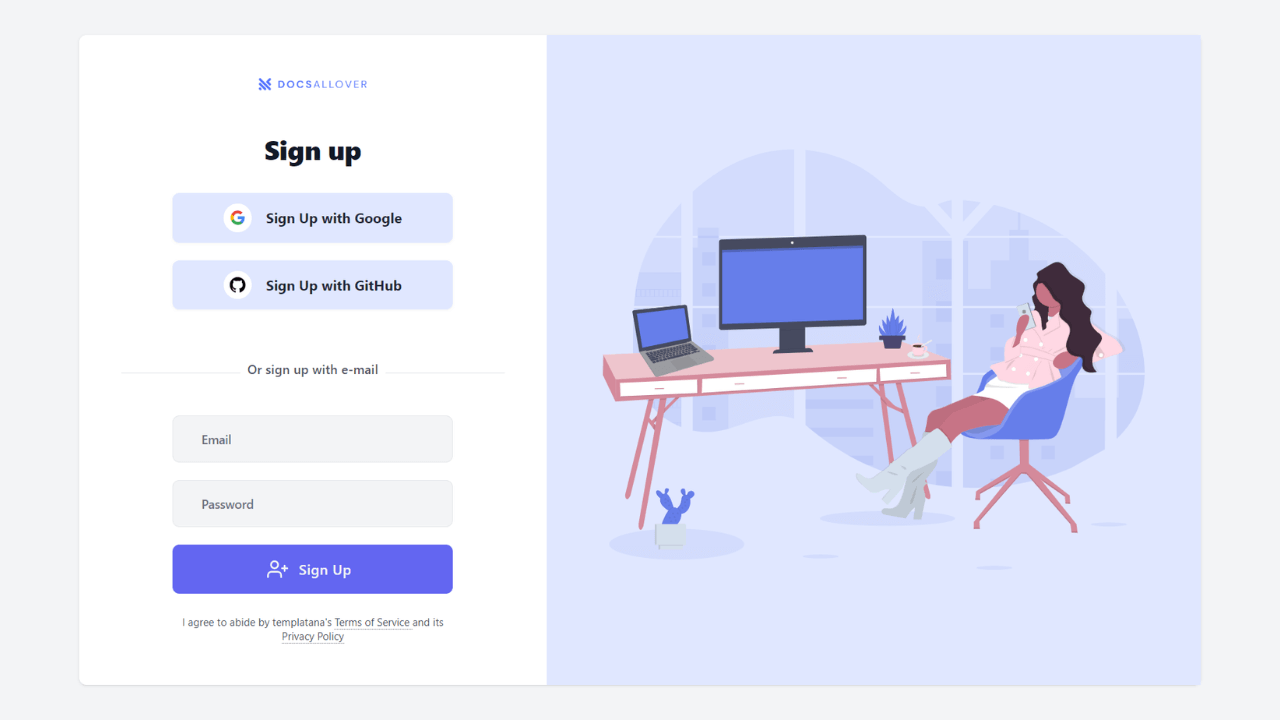
Responsive Registration Form Page with Image in Tailwind

Timeline Roadmap Example in Tailwind CSS
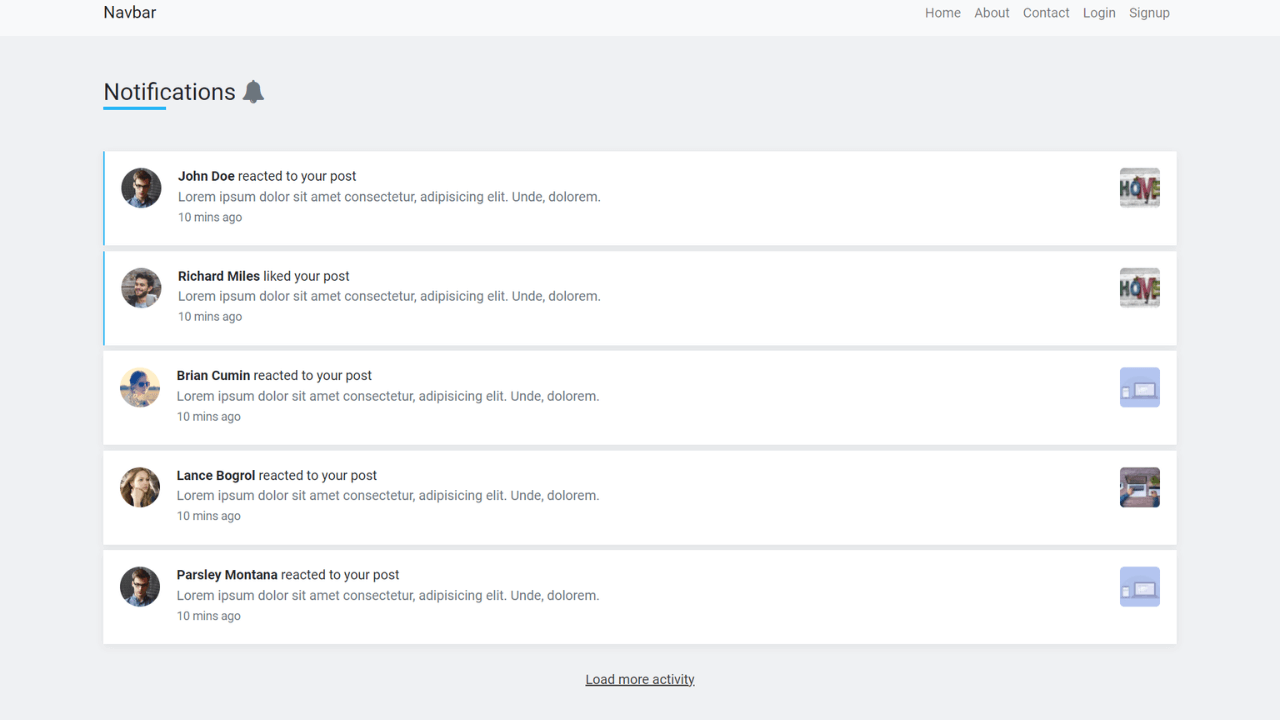
Notification Page Design Examples in Bootstrap 5
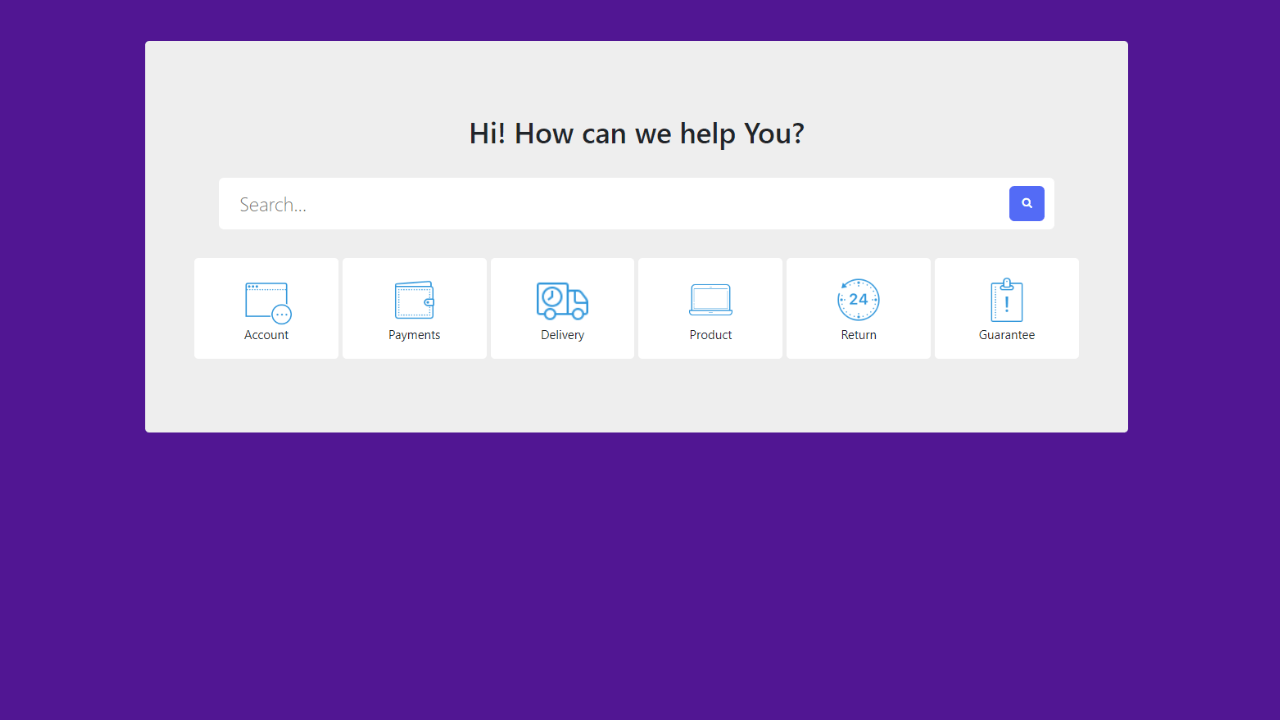
Need Help Support Page with Search in Bootstrap 5
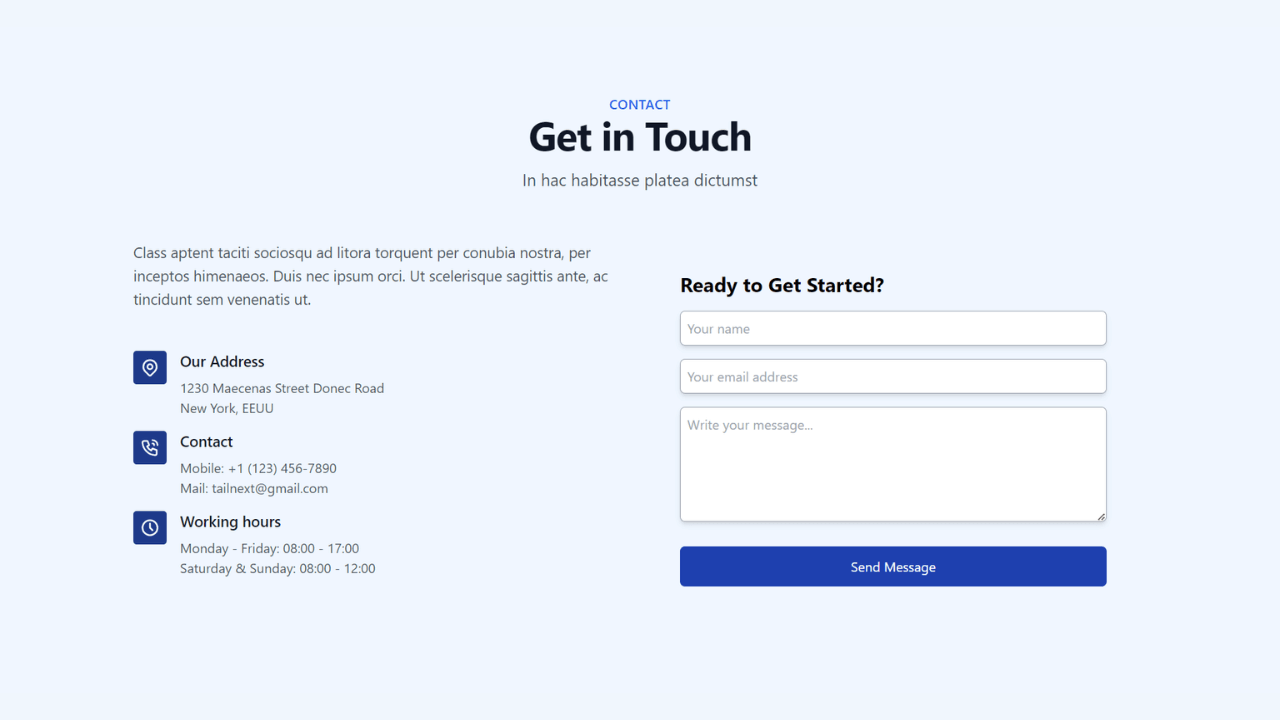
Responsive Get In Touch Contact Form in Tailwind CSS
Building Blocks for Your Web Pages
Explore a collection of pre-written HTML,CSS and Javascript
snippets to jumpstart your web development projects.