Real-time Password Strength Indicator in Bootstrap 5
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title> Password Strength Meter Example </title> <link rel='stylesheet' href='https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css'> </head> <body> <main> <body class="container-fluid bg-body-tertiary d-block"> <div class="row justify-content-center"> <div class="row justify-content-center"> <div class="col-12 col-md-6 col-lg-4" style="min-width: 500px;"> <div class="card bg-white mb-5 mt-5 border-0" style="box-shadow: 0 12px 15px rgba(0, 0, 0, 0.02);"> <div class="card-body p-5"> <label for="password-input" class="form-label fw-bold">Password</label> <input type="password" class="form-control" id="password-input" autocomplete="off" aria-autocomplete="list" aria-label="Password" aria-describedby="passwordHelp"> <div class="password-meter"> <div class="meter-section rounded me-2"></div> <div class="meter-section rounded me-2"></div> <div class="meter-section rounded me-2"></div> <div class="meter-section rounded"></div> </div> <div id="passwordHelp" class="form-text text-muted">Use 8 or more characters with a mix of letters, numbers & symbols. </div> </div> </div> </div> </div> </div> </body> </main> <script src='https://cdn.jsdelivr.net/npm/@popperjs/core@2.11.8/dist/umd/popper.min.js'></script> <script src='https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.min.js'></script> </body> </html>
.password-meter { display: flex; height: 5px; margin-top: 10px; } .meter-section { flex: 1; background-color: #ddd; } .weak { background-color: #ff4d4d; } .medium { background-color: #ffd633; } .strong { background-color: #00b300; } .very-strong { background-color: #009900; }
const passwordInput = document.getElementById('password-input'); const meterSections = document.querySelectorAll('.meter-section'); passwordInput.addEventListener('input', updateMeter); function updateMeter() { const password = passwordInput.value; let strength = calculatePasswordStrength(password); // Remove all strength classes meterSections.forEach((section) => { section.classList.remove('weak', 'medium', 'strong', 'very-strong'); }); // Add the appropriate strength class based on the strength value if (strength >= 1) { meterSections[0].classList.add('weak'); } if (strength >= 2) { meterSections[1].classList.add('medium'); } if (strength >= 3) { meterSections[2].classList.add('strong'); } if (strength >= 4) { meterSections[3].classList.add('very-strong'); } } function calculatePasswordStrength(password) { const lengthWeight = 0.2; const uppercaseWeight = 0.5; const lowercaseWeight = 0.5; const numberWeight = 0.7; const symbolWeight = 1; let strength = 0; // Calculate the strength based on the password length strength += password.length * lengthWeight; // Calculate the strength based on uppercase letters if (/[A-Z]/.test(password)) { strength += uppercaseWeight; } // Calculate the strength based on lowercase letters if (/[a-z]/.test(password)) { strength += lowercaseWeight; } // Calculate the strength based on numbers if (/\\d/.test(password)) { strength += numberWeight; } // Calculate the strength based on symbols if (/[^A-Za-z0-9]/.test(password)) { strength += symbolWeight; } return strength; }
This Bootstrap 5 snippet showcases a visually appealing and interactive password strength indicator that provides real-time feedback on the complexity of the entered password. It helps users create strong, secure passwords by highlighting the password strength using color-coded indicators.
Key Features:
- Real-time Feedback: The password strength is evaluated as the user types, providing immediate feedback on the password's complexity.
- Color-Coded Indicators: Different colors are used to represent different strength levels, making it easy for users to understand the password's security.
- Customizable Strength Criteria: The password strength criteria can be customized to meet specific security requirements.
- Clear Guidance: A message is displayed below the password field, providing specific recommendations for improving password strength.
Implementation:
- Include Bootstrap 5: Ensure you have Bootstrap 5 installed and configured in your project.
- Create the HTML Structure: Set up the basic HTML structure for the password field, strength indicator, and message container.
- Apply Bootstrap Classes: Use Bootstrap's CSS classes to style the input field and the strength indicator, such as form-group, input-group, progress, and progress-bar.
- Add JavaScript Functionality: Implement JavaScript code to evaluate the password strength based on predefined criteria, such as length, character types, and complexity. Update the strength indicator's color and the message accordingly. You can use JavaScript libraries like jQuery or native JavaScript for this purpose.
By following these steps, you can create a secure and user-friendly password strength indicator that helps users create strong and memorable passwords.
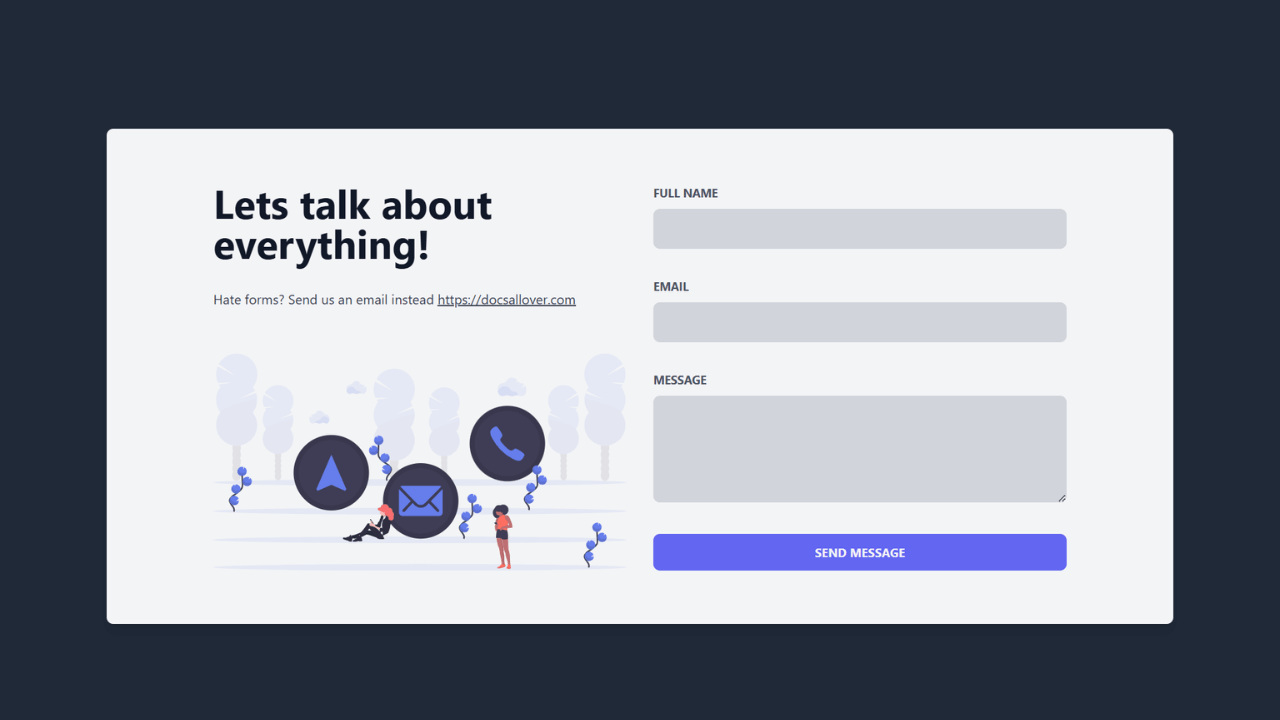
Stylish Contact Form with Image in Tailwind CSS
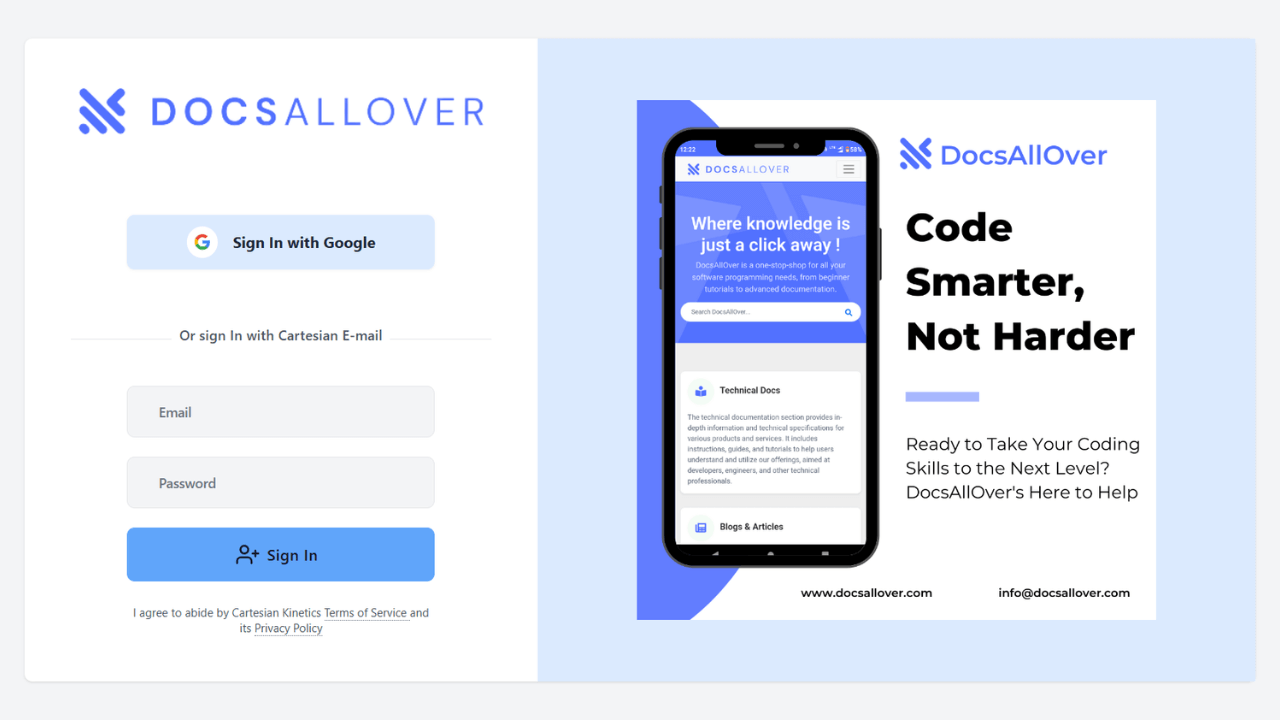
Responsive Registration Form with Image in Tailwind CSS
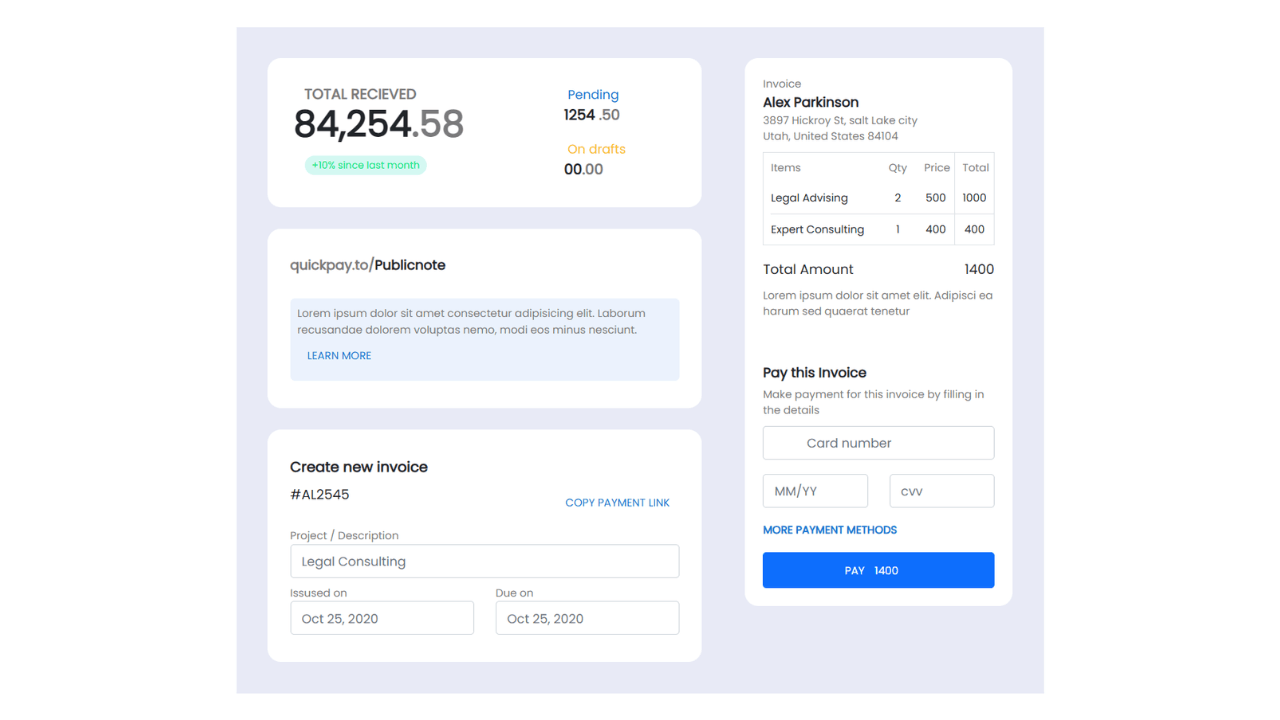
Bootstrap 5 Payment Method Form Page with Invoice
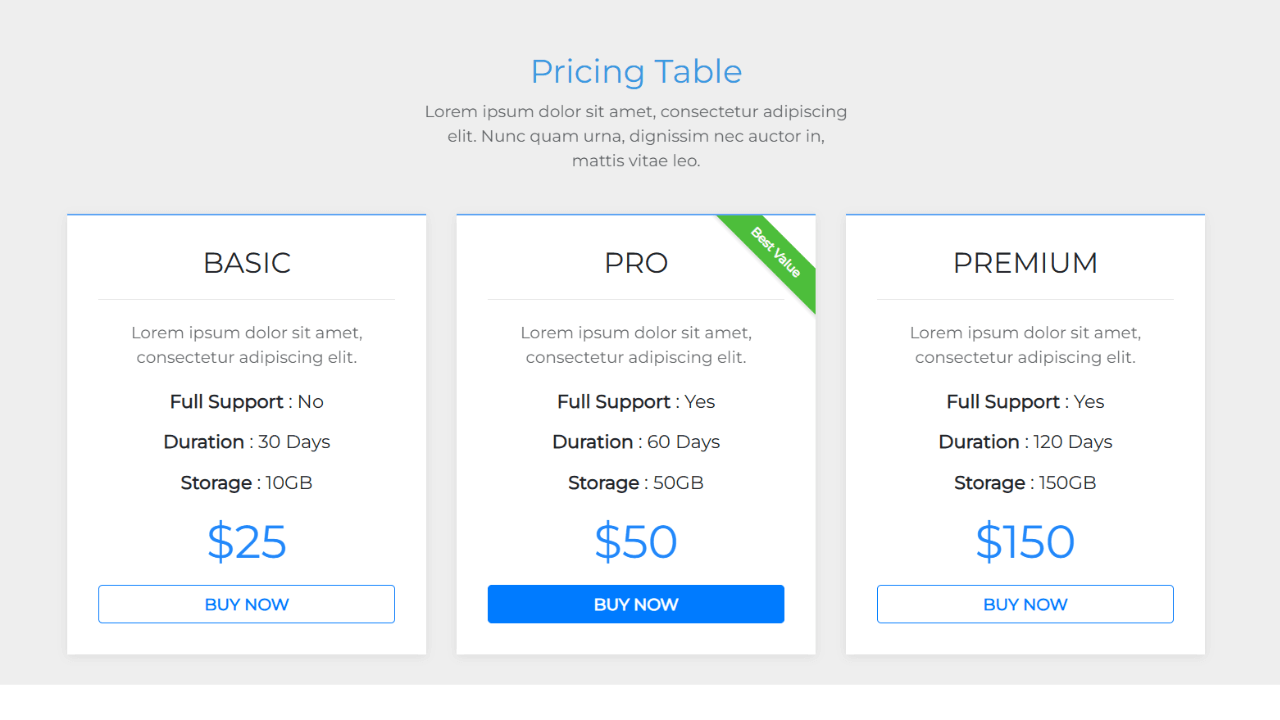
Modern Pricing Table Design with Bootstrap 4
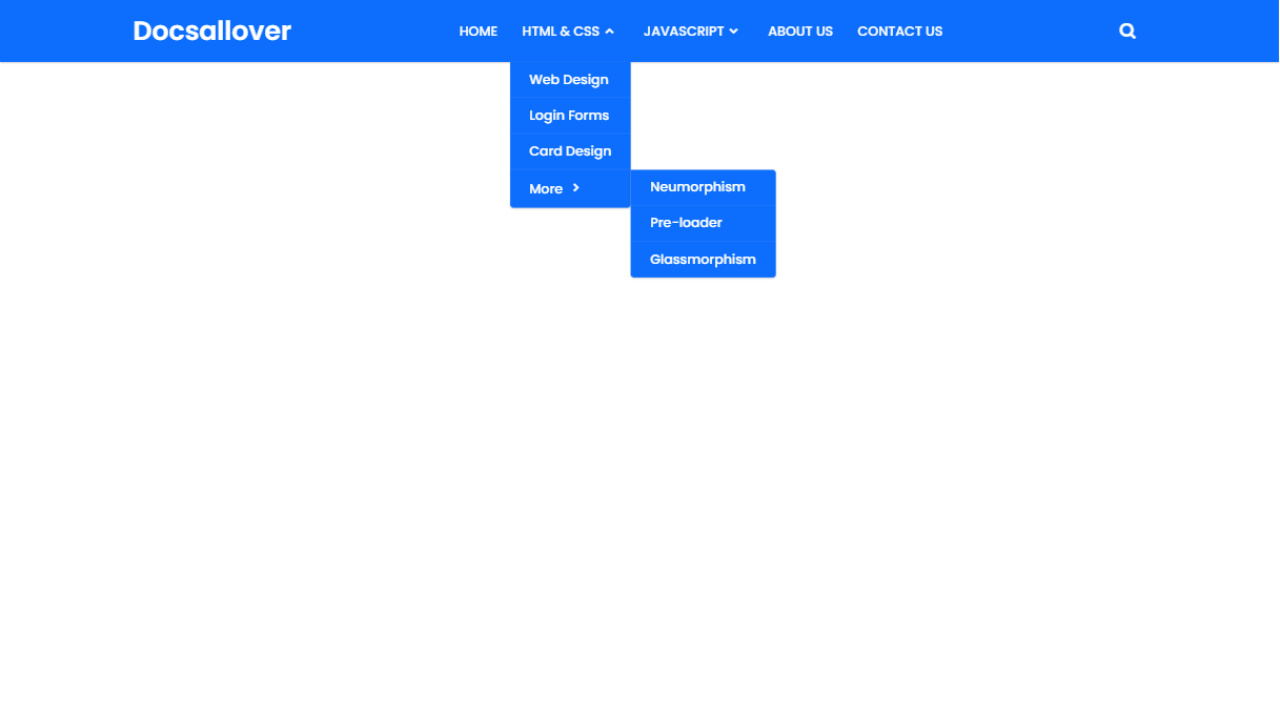
Responsive Multi-Level Drop-Down Navigation Menu in CSS
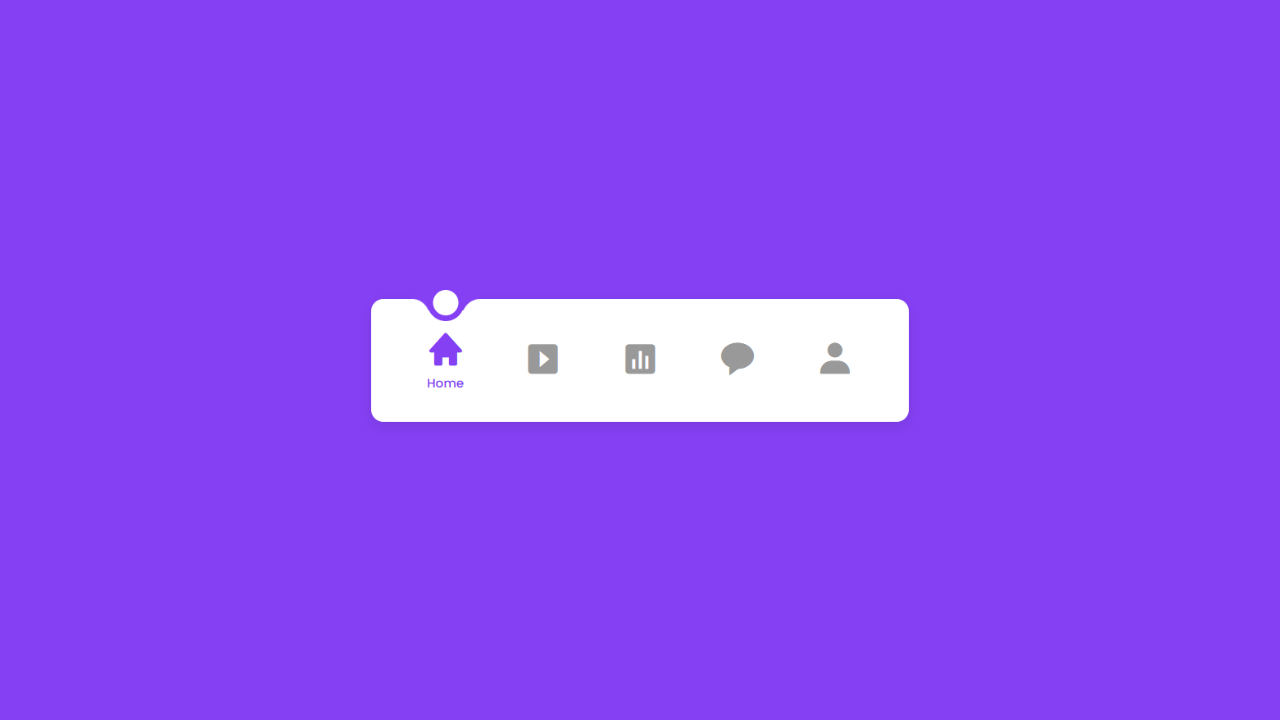
Interactive Bottom Navigation Bar Indicator with CSS
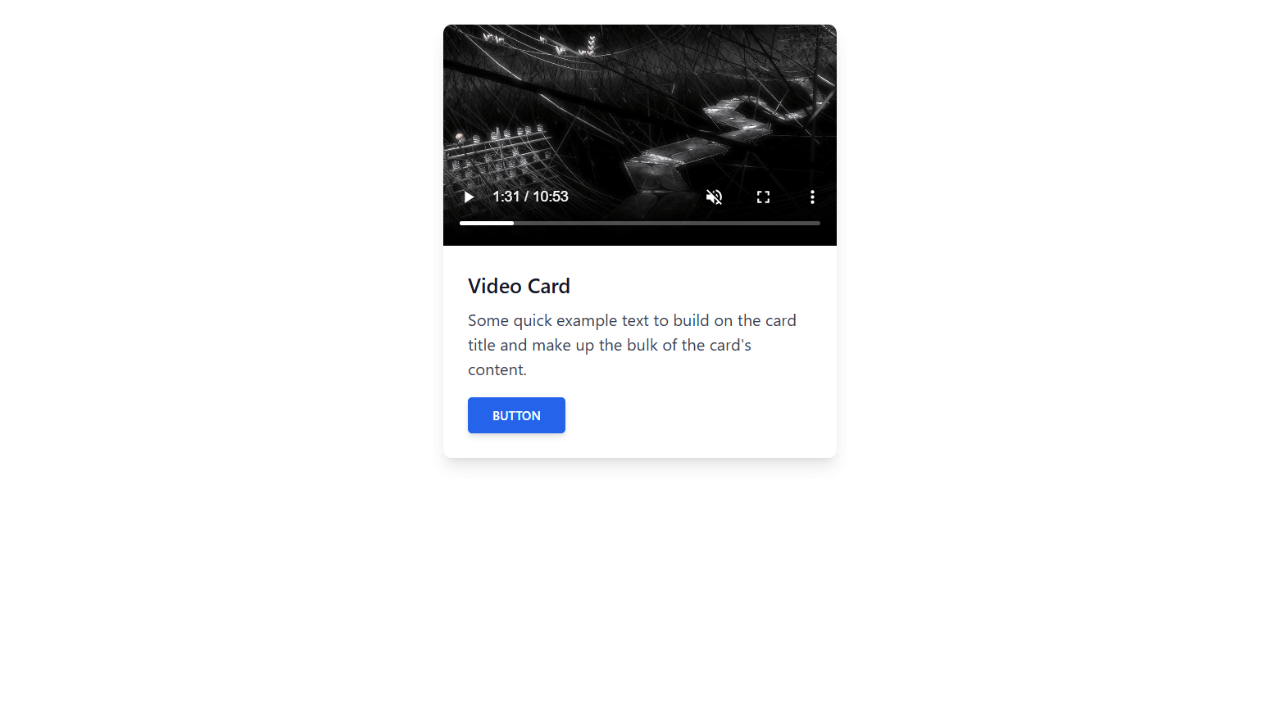
Responsive Video Card Component for Tailwind CSS
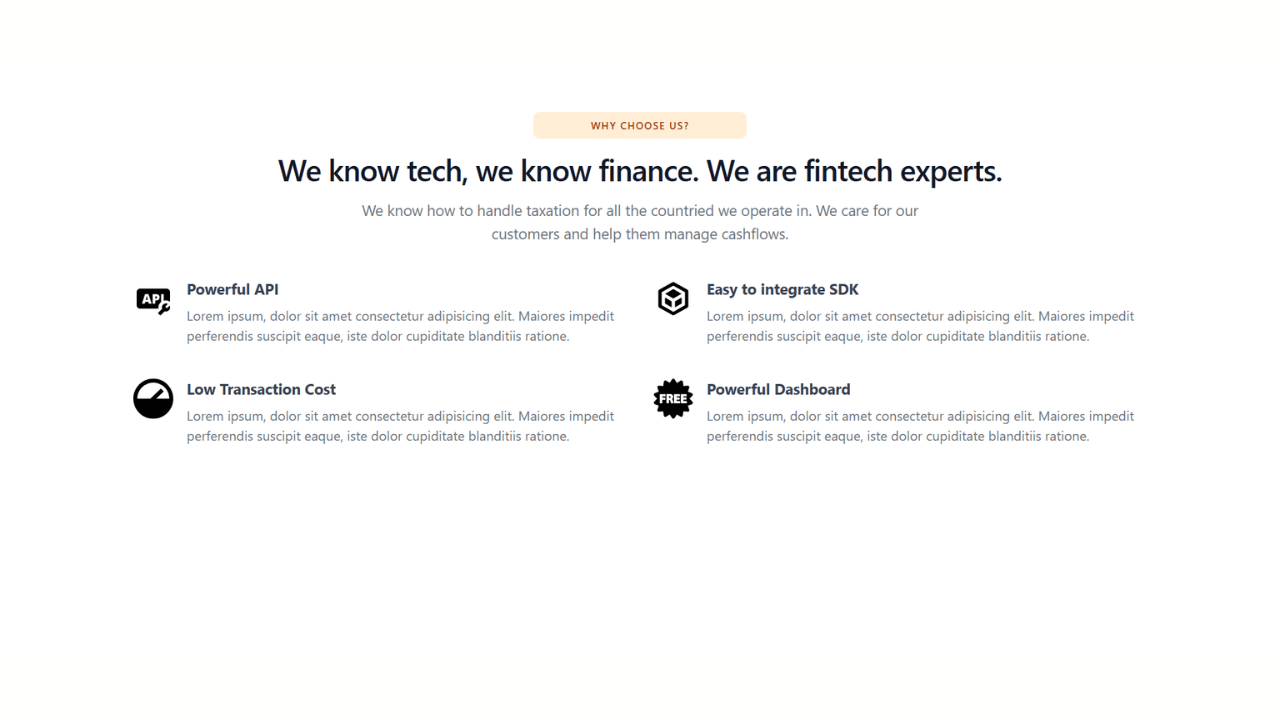
Responsive Feature Highlight Component with Tailwind CSS
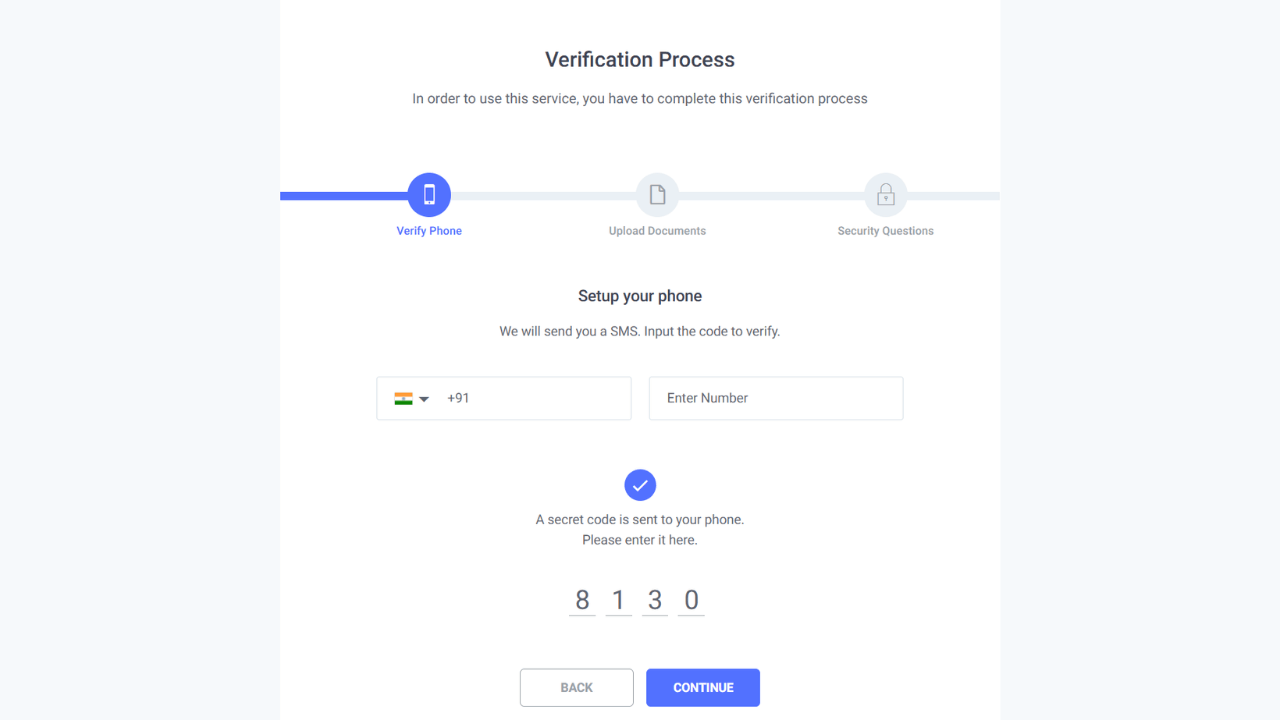
Multi-Step Form with International Phone Input and Nice Select
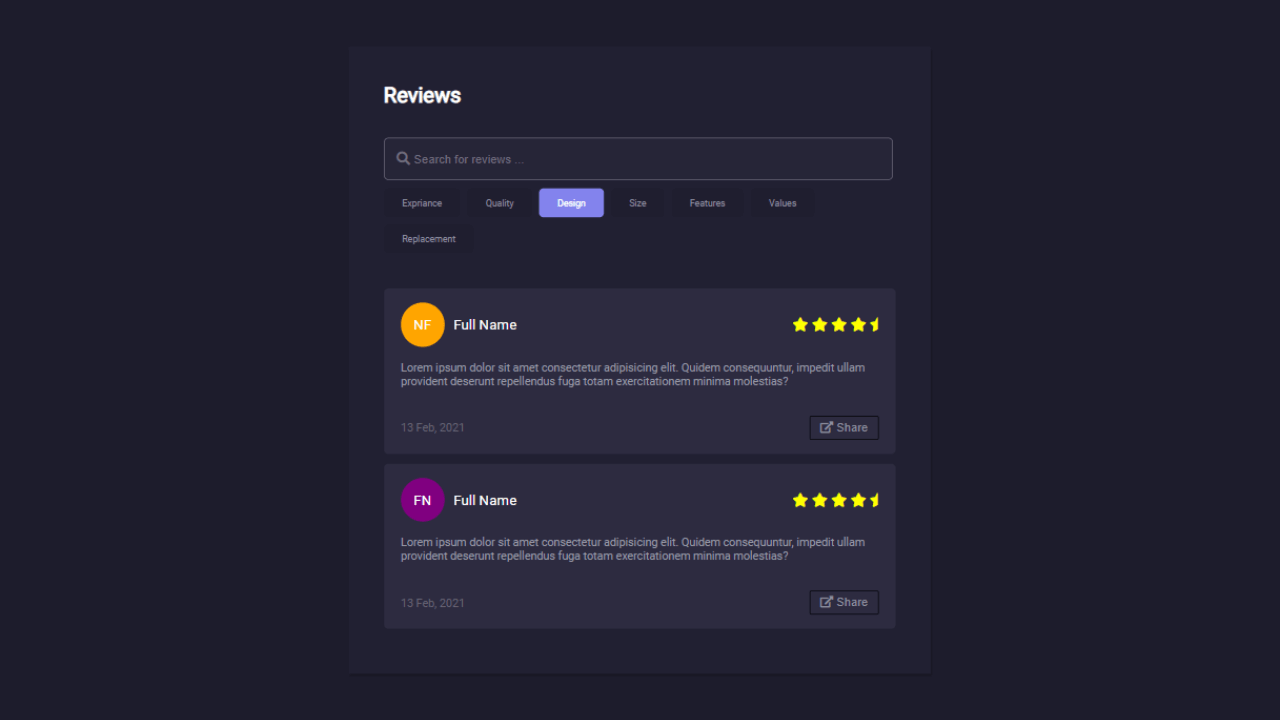
Responsive Review Cards Component with CSS3
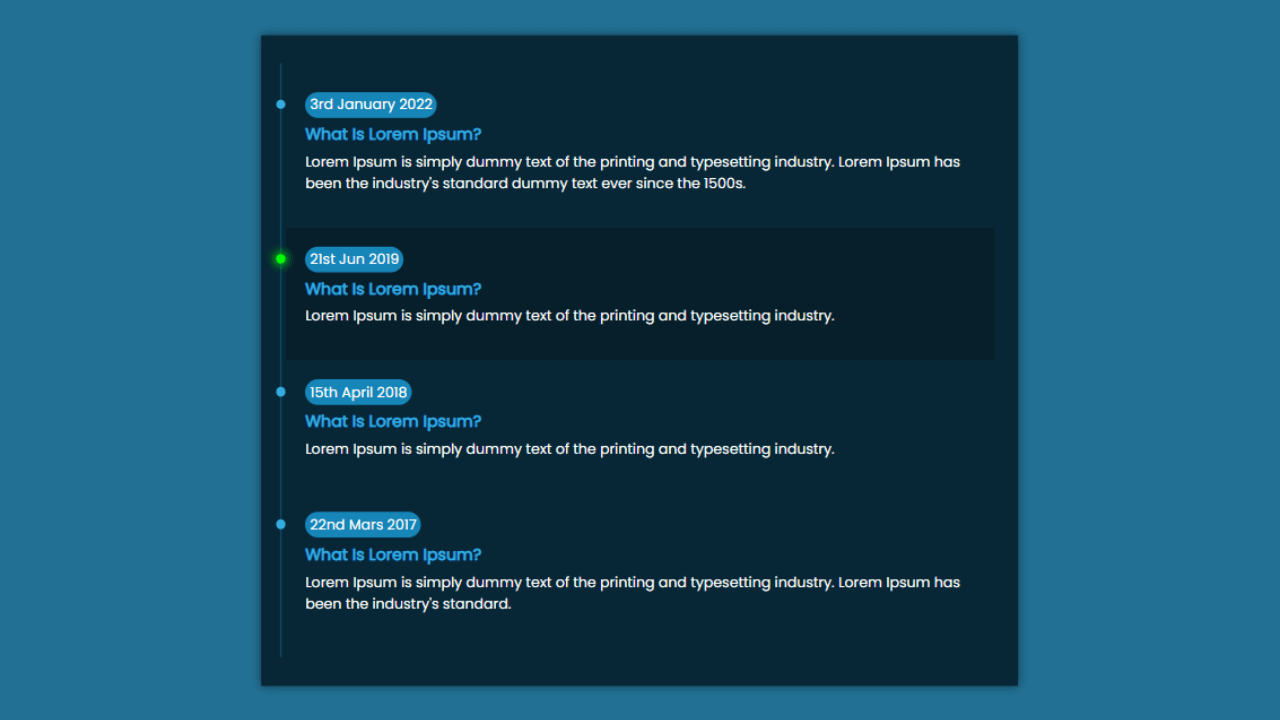
Responsive CSS3 Timeline Design With Hover Effects
Building Blocks for Your Web Pages
Explore a collection of pre-written HTML,CSS and Javascript
snippets to jumpstart your web development projects.